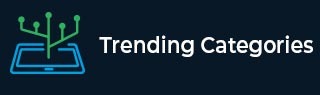
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
DateFormat.MEDIUM is a constant for medium style pattern.Firstly, we will create date object −Date dt = new Date(); DateFormat dateFormat;Let us format date for different locale with DateFormat.MEDIUM −// CHINESE dateFormat = DateFormat.getDateInstance(DateFormat.MEDIUM, Locale.CHINESE); // CANADA dateFormat = DateFormat.getDateInstance(DateFormat. MEDIUM, Locale.CANADA);The following is an example −Example Live Demoimport java.text.DateFormat; import java.util.Date; import java.util.Locale; public class Demo { public static void main(String args[]) { Date dt = new Date(); DateFormat dateFormat; // Date Format MEDIUM constant ... Read More

260 Views
To parse a number to binary, use the Integer.parseInt() method with binary as the first parameter and radix as 2 passed as a 2nd parameter.Let’s say the following is our integer −int val = 566;Now, use the Integer.parseInt() method −val = Integer.parseInt("11111111", 2);Using the Integer.toString() method will format the above value into binary −String str = Integer.toString(val, 2);The following is an example −Example Live Demopublic class Demo { public static void main(String []args){ int val = 566; val = Integer.parseInt("11111111", 2); System.out.println(val); String str = Integer.toString(val, 2); System.out.println(str); } }Output255 11111111

226 Views
Use System.nanoTime() method in Java to get the current value of the system timer in nanoseconds. It d returns the current value of the most precise available system timer, in nanoseconds.We are getting the system timer value in a long type −long res = System.nanoTime();The following is an example −Example Live Demopublic class Demo { public static void main(String[] args) { long res = System.nanoTime(); System.out.println("Current Value: " + res + " nanoseconds"); } }OutputCurrent Value: 49908709882168 nanoseconds

113 Views
Use arraycopy() method in Java to copy an array from the specified source array.Here, we have two arrays −int arr1[] = { 10, 20, 30, 40}; int arr2[] = { 3, 7, 20, 30};Now, we will use the arraycopy() method to copy the first two elements of the 1st array to the 2nd array −System.arraycopy(arr1, 0, arr2, 2, 2);The following is an example −Example Live Demoimport java.lang.*; public class Demo { public static void main(String[] args) { int arr1[] = { 10, 20, 30, 40}; int arr2[] = { 3, 7, 20, 30}; System.arraycopy(arr1, 0, arr2, 2, 2); System.out.print("New Array = "); System.out.print(arr2[0] + " "); System.out.print(arr2[1] + " "); System.out.print(arr2[2] + " "); System.out.print(arr2[3] + " "); } }OutputNew Array = 3 7 10 20

110 Views
Let’s say we need to round number to 3 decimal places, therefore use the following format −DecimalFormat decFormat = new DecimalFormat("0.000");Now, format the number −decFormat.format(37878.8989)Since we have used the DecimalFloat class above, therefore do import the following package −import java.text.DecimalFormat;The following is an example that rounds a number to 3 decimal places −Example Live Demoimport java.text.DecimalFormat; public class Demo { public static void main(String[] args) { DecimalFormat decFormat = new DecimalFormat("0.000"); System.out.println("Result = " + decFormat.format(37878.8989)); } }OutputResult = 37878.899Let us see another example that rounds a number ... Read More

71 Views
DecimalFormat is a concrete subclass of NumberFormat that formats decimal numbers. Let us set DecimalFormat("###E0") and use the format() method as well.DecimalFormat decFormat = new DecimalFormat("##E0"); System.out.println(decFormat.format(-267.9965)); System.out.println(decFormat.format(8.19)); System.out.println(decFormat.format(9897.88));Since we have used DecimalFormat class in Java, therefore importing the following package is a must −import java.text.DecimalFormat;The following is the complete example −Example Live Demoimport java.text.DecimalFormat; public class Demo { public static void main(String[] argv) throws Exception { DecimalFormat decFormat = new DecimalFormat("###E0"); System.out.println(decFormat.format(-267.9965)); System.out.println(decFormat.format(8.19)); ... Read More

55 Views
DecimalFormat is a concrete subclass of NumberFormat that formats decimal numbers. Let us set DecimalFormat("##E0") and use the format() method as well.DecimalFormat decFormat = new DecimalFormat("##E0"); System.out.println(decFormat.format(-189.8787)); System.out.println(decFormat.format(8.19)); System.out.println(decFormat.format(9897.88));Since, we have used DecimalFormat class in Java, therefore importing the following package is a must −import java.text.DecimalFormat;The following is the complete example −Example Live Demoimport java.text.DecimalFormat; public class Demo { public static void main(String[] argv) throws Exception { DecimalFormat decFormat = new DecimalFormat("##E0"); System.out.println(decFormat.format(-189.8787)); System.out.println(decFormat.format(8.19)); ... Read More

239 Views
DecimalFormat is a concrete subclass of NumberFormat that formats decimal numbers. Let us set DecimalFormat("00.00E0") and use the format() method as well.DecimalFormat decFormat = new DecimalFormat("00.00E0"); System.out.println(decFormat.format(-289.8787)); System.out.println(decFormat.format(8.19));Since, we have used DecimalFormat class in Java, therefore importing the following package is a must −import java.text.DecimalFormat;The following is the complete example −Example Live Demoimport java.text.DecimalFormat; public class Demo { public static void main(String[] argv) throws Exception { DecimalFormat decFormat = new DecimalFormat("00.00E0"); System.out.println(decFormat.format(-289.8787)); System.out.println(decFormat.format(8.19)); System.out.println(decFormat.format(9897.88)); ... Read More

151 Views
To display a currency in Java, use the following DecimalFormat −DecimalFormat decFormat = new DecimalFormat("\u00a4#, ##0.00");Since, we have used the DecimalFormat class, therefore do not forget to import the following package −import java.text.DecimalFormat;Now, let us learn how to display percentage −decFormat.format(877.80) decFormat.format(8.19) decFormat.format(9897.88)The above will be displayed as −$877.80 $8.19 $9, 897.88The following is the complete example −Example Live Demoimport java.text.DecimalFormat; public class Demo { public static void main(String[] argv) throws Exception { // for currency DecimalFormat decFormat = new DecimalFormat("\u00a4#, ##0.00"); ... Read More

1K+ Views
To display a percentage in Java, use the following DecimalFormat.DecimalFormat decFormat = new DecimalFormat("#%");Since, we have used the DecimalFormat class, therefore do not forget to import the following package −import java.text.DecimalFormat;Now, let us learn how to display percentage −decFormat.format(0.80) decFormat.format(-0.19) decFormat.format(1.88)The above will be displayed as −80% -19% 188%The following is the complete example −Example Live Demoimport java.text.DecimalFormat; public class Demo { public static void main(String[] argv) throws Exception { DecimalFormat decFormat = new DecimalFormat("#%"); System.out.println(decFormat.format(0.80)); System.out.println(decFormat.format(-0.19)); ... Read More