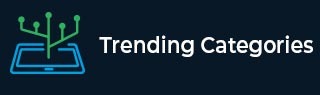
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

777 Views
Use the System.getProperty() method in Java to get the Java Home Directory.It’s syntax is −String getProperty(String key)Above, the key is the name of the system property. Since, we want the Java Home Directory name, therefore we will add the key as −java.homeThe following is an example −Example Live Demopublic class Demo { public static void main(String[] args) { System.out.println("Get Java Home Directory = " + System.getProperty("java.home")); System.out.print("Java Specification Version: "); System.out.println(System.getProperty("java.specification.version")); System.out.print("java Runtime Environment ... Read More

151 Views
To display the path separator, use the Properties class and import the following package −import java.util.Properties;Use the getProperties() method first and create an object −Properties p = System.getProperties();Now, set the key for path separator −p.getProperty("path.separator")The following is an example −Example Live Demoimport java.util.Properties; public class Demo { public static void main(String[] args) { Properties p = System.getProperties(); System.out.println("Separator is "+p.getProperty("path.separator")); } }OutputSeparator is :

1K+ Views
Use the System.getProperty() method in Java to get the Operating System name and version.It’s syntax is −String getProperty(String key)Above, the key is the name of the system property. Since, we want the OS name and version, therefore we will add the key as −Operating System name - os.name Operating System version - os.versionThe following is an example −Example Live Demopublic class Demo { public static void main(String []args){ System.out.println("OS Name: " + System.getProperty("os.name")); System.out.println("OS Version: " + System.getProperty("os.version")); } }OutputOS Name: Linux OS ... Read More

503 Views
Use the System.getProperty() method in Java to get the Java Runtime Environment.It’s syntax is −String getProperty(String key)Above, the key is the name of the system property. Since, we want the Java Runtime Environment name, therefore we will add the key as −java.versionThe following is an example −Example Live Demopublic class Demo { public static void main(String[] args) { System.out.print("Java Specification Version: "); System.out.println(System.getProperty("java.specification.version")); System.out.print("java Runtime Environment (JRE) version: "); System.out.println(System.getProperty("java.version")); } ... Read More

284 Views
Use the System.getProperty() method in Java to get the Java Specification Version.It’s syntax is −String getProperty(String key)Above, the key is the name of the system property. Since, we want the Java Specification Version name, therefore we will add the key as −java.specification.versionThe following is an example −Example Live Demopublic class Demo { public static void main(String[] args) { System.out.print("Java Specification Version: "); System.out.println(System.getProperty("java.specification.version")); } }OutputJava Specification Version: 1.8

8K+ Views
When the full name is provided, the initials of the name are printed with the last name is printed in full. An example of this is given as follows −Full name = Amy Thomas Initials with surname is = A. ThomasA program that demonstrates this is given as follows −Example Live Demoimport java.util.*; public class Example { public static void main(String[] args) { String name = "John Matthew Adams"; System.out.println("The full name is: " + name); System.out.print("Initials with surname is: "); int len = name.length(); ... Read More

164 Views
To compare Float in Java, use the following methods −Method 1 − compareTo(newFloat) method in JavaThe java.lang.Float.compareTo() method compares two Float objects. This method returns the value 0 if the new float value is numerically equal to this Float; a value less than 0 if this Float is numerically less than new float; and a value greater than 0 if this Float is numerically greater than new float.Here is an example −Example Live Demoimport java.lang.*; public class Demo { public static void main(String args[]) { Float f1 = new Float("25.2"); Float f2 = new ... Read More

549 Views
To apply modulus (%) operator to floating-point values in an effortless task. Let us see how.We have the following two values −double one = 9.7; double two = 1.2;Let us now apply the modulus operator −one % twoThe following is the complete example that displays the output as well −Example Live Demopublic class Demo { public static void main(String args[]) { double one = 9.7; double two = 1.2; System.out.println( one % two ); } }Output0.09999999999999964

100 Views
The following is the supplied byte array −byte[] b = new byte[]{'x', 'y', 'z'};We have created a custom method “display” here and passed the byte array value. The same method converts byte array to hex string −public static String display(byte[] b1){ StringBuilder strBuilder = new StringBuilder(); for(byte val : b1){ strBuilder.append(String.format("%02x", val&0xff)); } return strBuilder.toString(); }Let us see the entire example now −Example Live Demopublic class Demo { public static void main(String args[]) { byte[] b = new byte[]{'x', 'y', ... Read More

1K+ Views
DateFormat.LONG is a constant for long style pattern.Firstly, we will create date object −Date dt = new Date(); DateFormat dateFormat;Let us format date for different locale with DateFormat.LONG −// ITALY dateFormat = DateFormat.getDateInstance(DateFormat.LONG, Locale.ITALY); // CANADA dateFormat = DateFormat.getDateInstance(DateFormat. LONG, Locale.CANADA);The following is an example −Example Live Demoimport java.text.DateFormat; import java.util.Date; import java.util.Locale; public class Demo { public static void main(String args[]) { Date dt = new Date(); DateFormat dateFormat; // Date Format LONG constant ... Read More