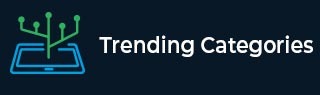
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

390 Views
To vertically align numeric values in Java, use Formatter. For working with Formatter class, import the following package.import java.util.Formatter;Take an array −double arr[] = { 2.5, 4.8, 5.7, 6.5, 9.4, 8.4, 9.5, 10.2, 11.5 };While displaying this double array values, use the %f to set spaces −for (double d : arr) { f.format("%12.2f %12.2f %12.2f", d, Math.ceil(d), Math.floor(d)); }Above, we have also set the decimal places i.e. 12.2f is for 2 decimal places.The following is an example −Example Live Demoimport java.util.Formatter; public class Demo { public static void main(String[] argv) throws Exception { ... Read More

347 Views
For Floating-point hexadecimal form, use the %a format specifier.For Formatter, import the following package −import java.util.Formatter;Now create a Formatter object like this −Formatter f = new Formatter();Use the format() method for floating-point hexadecimal −f.format("%a", 298.45)The following is an example −Example Live Demoimport java.util.Formatter; public class Demo { public static void main(String args[]) { Formatter f = new Formatter(); // Floating-point hexadecimal form System.out.println(f.format("%a", 298.45)); } }Output0x1.2a73333333333p8

156 Views
Format specifier for Hash Code us %h.Firstly, create a new object for Formatter and Calendar −Formatter f = new Formatter(); Calendar c = Calendar.getInstance();For hash code −f.format("%h", c)The following is an example that finds the hash code −Example Live Demoimport java.util.Calendar; import java.util.Formatter; public class Demo { public static void main(String args[]) { Formatter f = new Formatter(); Calendar c = Calendar.getInstance(); System.out.println("Hash Code: "+f.format("%h", c)); } }OutputHash Code: 4b899958

151 Views
For Formatter, import the following package −import java.util.Formatter;Now create a Formatter object like this −Formatter f1 = new Formatter(); Formatter f2 = new Formatter(); Formatter f3 = new Formatter();If you want a Format specifier for Octal, use %o −f3.format("Octal values %o %o %o", 15, 55, 78);If you want a Format specifier for Hexadecimal, use %x −f2.format("Hexadecimal values %x %x %x", 24, 98, 110);The following is an example −Example Live Demoimport java.util.Formatter; public class Demo { public static void main(String args[]) { Formatter f1 = new Formatter(); ... Read More

232 Views
For Formatter, import the following package −import java.util.Formatter;Now create a Formatter object −Formatter f1 = new Formatter();Now, we will format the output with Formatter −f1.format("Rank and Percentage of %s = %d %f", "John", 2, 98.5);The following is an example −Example Live Demoimport java.util.Formatter; public class Demo { public static void main(String args[]) { Formatter f1 = new Formatter(); Formatter f2 = new Formatter(); f1.format("Rank and Percentage of %s = %d %f", "John", 2, 98.5); ... Read More

98 Views
For locale-specific formatting, firstly import the following packages.import java.util.Calendar; import java.util.Formatter; import java.util.Locale;Create a Formatter and Calendar object −Formatter f = new Formatter(); Calendar c = Calendar.getInstance();We are formatting for different Locales −f.format(Locale.TAIWAN, "Locale.TAIWAN: %tc", c); f.format(Locale.ITALY, "Locale.ITALY: %tc", c);The following is an example −Example Live Demoimport java.util.Calendar; import java.util.Formatter; import java.util.Locale; public class Demo { public static void main(String args[]) { Formatter f = new Formatter(); Calendar c = Calendar.getInstance(); f.format(Locale.TAIWAN, "Locale.TAIWAN: %tc", c); ... Read More

848 Views
To get the temporary directory in Java, use the System.getProperty() method. Use theIt’s syntax is −String getProperty(String key)Above, the key is the name of the system property. Since, we want the Java Home Directory name, therefore we will add the key as −java.io.tmpdirThe following is an example −Example Live Demopublic class Demo { public static void main(String []args){ String strTmp = System.getProperty("java.io.tmpdir"); System.out.println("OS current temporary directory: " + strTmp); System.out.println("OS Name: " + System.getProperty("os.name")); ... Read More

52 Views
Let us first set the calendar object −Calendar calendar = Calendar.getInstance();Use the getMaximum() method in Java to returns the maximum value for the given calendar field. We will use it to set the minute, second and milliseconds.For seconds −calendar.set(Calendar.SECOND, calendar.getMaximum(Calendar.SECOND));For milliseconds −calendar.set(Calendar.MILLISECOND, calendar.getMaximum(Calendar.MILLISECOND));The following is an example that returns a Date set to the last possible millisecond of the minute −Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] argv) throws Exception { Calendar calendar = Calendar.getInstance(); // seconds calendar.set(Calendar.SECOND, calendar.getMaximum(Calendar.SECOND)); // milliseconds ... Read More

110 Views
Use the getMaximum() method in Java to returns the maximum value for the given calendar field. We will use it to set the minute, second and milliseconds.Let us first declare a calendar object −Calendar dateNoon = Calendar.getInstance();Now, we will set the hour, minute, second and millisecond to the first possible millisecond of the month after midnight −// hour, minute and second calendar.set(Calendar.HOUR_OF_DAY, calendar.getMaximum(Calendar.HOUR_OF_DAY)); calendar.set(Calendar.MINUTE, calendar.getMaximum(Calendar.MINUTE)); calendar.set(Calendar.SECOND, calendar.getMaximum(Calendar.SECOND)); // millisecond calendar.set(Calendar.MILLISECOND, calendar.getMaximum(Calendar.MILLISECOND));The following is an example that return a Date set to the first possible millisecond of the month after midnight −Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { ... Read More

240 Views
Use the getMaximum() method in Java to returns the maximum value for the given calendar field. We will use it to set the minute, second and millisecond.Let us first declare a calendar object −Calendar dateNoon = Calendar.getInstance();Now, we will set the hour, minute, second and millisecond to the last possible millisecond on the month before midnight.// hour, minute and second calendar.set(Calendar.HOUR_OF_DAY, calendar.getMaximum(Calendar.HOUR_OF_DAY)); calendar.set(Calendar.MINUTE, calendar.getMaximum(Calendar.MINUTE)); calendar.set(Calendar.SECOND, calendar.getMaximum(Calendar.SECOND)); // millisecond calendar.set(Calendar.MILLISECOND, calendar.getMaximum(Calendar.MILLISECOND));Now, do the following for month and day of month −calendar.set(Calendar.DAY_OF_MONTH, 1); // first day of month calendar.add(Calendar.MONTH, 1); calendar.add(Calendar.DAY_OF_MONTH, -1);The following is an example to return a Date set to ... Read More