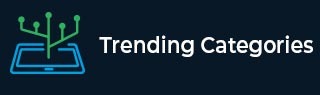
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

136 Views
The toString() method gives string representation to a Long. Let’s say we have the following Long object −Long longObj = new Long(70); System.out.println("Long: " + longObj);To convert it to String, the toString() method is used −String myStr = longObj.toString();The following is the complete example −Example Live Demopublic class Demo { public static void main(String[] args) { // Long Long longObj = new Long(70); System.out.println("Long: " + longObj); // conversion String myStr = longObj.toString(); System.out.println("Converted to String: " + myStr); } }OutputLong: 70 Converted to String: 70

308 Views
To convert String to Long, use the valueOf() method.Let’s say the following is our string.// string String myStr = "5"; System.out.println("String: "+myStr);To convert it to Long, we have used valueOf() −Long longObj = Long.valueOf(myStr);The following is the complete example to convert a String value to Long −Example Live Demopublic class Demo { public static void main(String[] args) { // string String myStr = "5"; System.out.println("String: "+myStr); // long Long longObj = Long.valueOf(myStr); System.out.println("Converted to Long: "+longObj); } }OutputString: 5 Converted to Long: 5

59 Views
Firstly, let us declare and initialize three long values.long val1 = 88799; long val2 = 98567; long val3 = 98768;Now, find the minimum of three long values using the following condition −// checking for maximum if (val2 < val1) { val1 = val2; } if (val3 < val1) { val1 = val3; }The following is the complete example to get the minimum value −Example Live Demopublic class Demo { public static void main(String[] args) { long val1 = 88799; long ... Read More

139 Views
Firstly, let us declare and initialize three long values −long val1 = 98799; long val2 = 98567; long val3 = 98768;Now, find the maximum of three long values using the following condition −// checking for maximum if (val2 > val1) { val1 = val2; } if (val3 > val1) { val1 = val3; }The following is the complete example to get the maximum value −Example Live Demopublic class Demo { public static void main(String[] args) { long val1 = 98799; ... Read More

230 Views
The “m” format is to format minutes i.e. 1, 2, 3, 4, etc. Here, we will use the following.SimpleDateFormat("m");Let us see an example −// displaying minutes in m format SimpleDateFormat simpleformat = new SimpleDateFormat("m"); String strMinute = simpleformat.format(new Date()); System.out.println("Minutes in m format = "+strMinute);Above, we have used the SimpleDateFormat class, therefore the following package is imported.import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time ... Read More

144 Views
The “KK” format in Java Date is used to display hour in 00-11.. Use SimpleDateFormat("KK") to get the same format −// displaying hour in KK format SimpleDateFormat simpleformat = new SimpleDateFormat("KK"); String strHour = simpleformat.format(new Date()); System.out.println("Hour in KK format = "+strHour);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time ... Read More

1K+ Views
To calculate distance light travels, we need to follow the basic formulae to calculate distance.Distance = Speed x TimeHere, the following are the parameters −speed = 186000; days = 365; seconds = days * 24 * 60 * 60;Above, we calculated the time in seconds for an year −days = 365; seconds = days * 24 * 60 * 60;The following is the complete example to calculate distance light travels −Example Live Demopublic class Demo { public static void main(String[] args) { int speed; long days, seconds, dist; speed = 186000; days = 365; seconds = days * 24 * 60 * 60; dist = speed * seconds; System.out.print("Light travels: "+dist + " miles"); } }OutputLight travels: 5865696000000 miles

7K+ Views
To convert long primitive to Long object, follow the below steps.Let’s say the following is our long primitive.// primitive long val = 45; System.out.println("long primitive: "+val);Now, to convert it to Long object is not a tiresome task. Include the same long value while creating a new Long object −// object Long myObj = new Long(val); System.out.println("Long object: "+myObj);The following is the complete example −Example Live Demopublic class Demo { public static void main(String[] args) { // primitive long val = 45; ... Read More

485 Views
Let’s say we have Long object here.Long myObj = new Long("9879");Now, if we want to convert this Long to short primitive data type. For that, use the in-built shortValue() method −// converting to short primitive types short shortObj = myObj.shortValue(); System.out.println(shortObj);In the same way convert Long to another numeric primitive data type int. For that, use the in-built intValue() method −// converting to int primitive types int intObj = myObj.intValue(); System.out.println(intObj);The following is an example wherein we convert Long to numeric primitive types short, int, float, etc −Example Live Demopublic class Demo { public static void main(String[] args) ... Read More

620 Views
To check for Long overflow, we need to check the Long.MAX_VALUE with the subtracted long result. Here, Long.MAX_VALUE is the maximum value of Long type in Java.Let us see an example wherein long integers are subtracted and if the result is still more than the Long.MAX_VALUE, then an exception is thrown −The following is an example showing how to check for Long overflow −Example Live Demopublic class Demo { public static void main(String[] args) { long val1 = 70123; long val2 = 10567; ... Read More