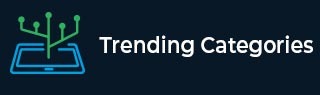
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

263 Views
Use the zzzz format like this for TimeZone.SimpleDateFormat("zzzz");Let us see an example −// displaying timezone in zzzz format simpleformat = new SimpleDateFormat("zzzz"); String strTimeZone = simpleformat.format(new Date()); System.out.println("TimeZone in zzzz format = "+strTimeZone);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar cal = Calendar.getInstance(); ... Read More

181 Views
The z format means General Time Zone. We will use it like this.SimpleDateFormat("z");Let us see an example −// displaying timezone in z format SimpleDateFormat simpleformat = new SimpleDateFormat("z"); String strTimeZone = simpleformat.format(new Date()); System.out.println("TimeZone in z format = "+strTimeZone);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar ... Read More

92 Views
The ss format for seconds is like representing seconds 01, 02, 03, 04, etc. We will use it like this.SimpleDateFormat("ss");Let us see an example −// displaying seconds in ss format simpleformat = new SimpleDateFormat("ss"); String strSeconds = simpleformat.format(new Date()); System.out.println("Seconds in ss format = "+strSeconds);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time ... Read More

110 Views
The “s” format for seconds is like representing 1, 2, 3, 4 seconds, etc. We will use it like this.SimpleDateFormat("s");Let us see an example −// displaying seconds in s format simpleformat = new SimpleDateFormat("s"); String strSeconds = simpleformat.format(new Date()); System.out.println("Seconds in s format = "+strSeconds);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time ... Read More

189 Views
The “M” format is to format months i.e. 1, 2, 3, 4, etc. Here, we will use the following.SimpleDateFormat("M");Let us see an example −// displaying month with M format simpleformat = new SimpleDateFormat("M"); String strMonth = simpleformat.format(new Date()); System.out.println("Month in M format = "+strMonth);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time ... Read More

50 Views
DecimalFormat is a concrete subclass of NumberFormat that formats decimal numbers. Let us set DecimalFormat("0000000000E0") first.Format f = new DecimalFormat("0000000000E0");Now, we will format a number and display the result in a string using the format() method −String res = f.format(-97579.9146);Since, we have used both Format and DecimalFormat class in Java, therefore importing the following packages in a must −import java.text.DecimalFormat; import java.text.Format;The following is the complete example −Example Live Demoimport java.text.DecimalFormat; import java.text.Format; public class Demo { public static void main(String[] argv) throws Exception { Format f = new DecimalFormat("0000000000E0"); ... Read More

72 Views
DecimalFormat is a concrete subclass of NumberFormat that formats decimal numbers.Let us set DecimalFormat("000E00") first −Format f = new DecimalFormat("000E00");Now, we will format a number and display the result in a string using the format() method −String res = f.format(-5977.3427);Since, we have used both Format and DecimalFormat class in Java, therefore importing the following packages in a must −import java.text.DecimalFormat; import java.text.Format;The following is an example −Example Live Demoimport java.text.DecimalFormat; import java.text.Format; public class Demo { public static void main(String[] argv) throws Exception { Format f = new DecimalFormat("000E00"); ... Read More

87 Views
DecimalFormat is a concrete subclass of NumberFormat that formats decimal numbers.Let us set DecimalFormat("00E00") first.Format f = new DecimalFormat("00E00");Now, we will format a number and display the result in a string using the format() method −String res = f.format(-5977.3427);Since, we have used both Format and DecimalFormat class in Java, therefore importing the following packages in a must −import java.text.DecimalFormat; import java.text.Format;The following the complete example −Example Live Demoimport java.text.DecimalFormat; import java.text.Format; public class Demo { public static void main(String[] argv) throws Exception { Format f = new DecimalFormat("00E00"); ... Read More

183 Views
The “mm” format is to format minutes i.e. 01, 02, 03, 04, etc. Here, we will use the following −SimpleDateFormat("mm");Let us see an example −// displaying minutes in mm format SimpleDateFormat simpleformat = new SimpleDateFormat("mm"); String strMinute = simpleformat.format(new Date()); System.out.println("Minutes in mm format = "+strMinute);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time ... Read More

143 Views
The “K” format in Java Date is used to display hour in 0-11 in AM/PM format. Use SimpleDateFormat("K") to get the same format.// displaying hour in K format SimpleDateFormat simpleformat = new SimpleDateFormat("K"); String strHour = simpleformat.format(new Date()); System.out.println("Hour in K format = "+strHour);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time ... Read More