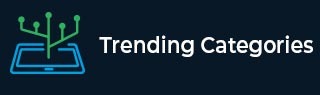
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

347 Views
Use the ‘j’ date conversion character to display three-digit day of the year.System.out.printf("Three-digit Day of the Year: %tj/%Tj", d, d);Above, d is a date object −Date d = new Date();The following is an example −Example Live Demoimport java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] args) throws Exception { Date d = new Date(); DateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy hh:mm:ss a"); String format = dateFormat.format(d); System.out.println("Current date and time = " + format); System.out.printf("Four-digit Year = %TY", d); ... Read More

1K+ Views
Use the ‘y’ date conversion character to display two-digit year.System.out.printf("Two-digit Year = %TY", d);Above, d is a date object −Date d = new Date();The following is an example −Example Live Demoimport java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] args) throws Exception { Date d = new Date(); DateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy hh:mm:ss a"); String format = dateFormat.format(d); System.out.println("Current date and time = " + format); System.out.printf("Four-digit Year = %TY", d); System.out.printf("Two-digit Year = %ty", d); ... Read More

593 Views
Use the ‘Y’ date conversion character to display four-digit year.System.out.printf("Four-digit Year = %TY",d);Above, d is a date object −Date d = new Date();The following is an example −Example Live Demoimport java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] args) throws Exception { Date d = new Date(); DateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy hh:mm:ss a"); String format = dateFormat.format(d); System.out.println("Current date and time = " + format); System.out.printf("Four-digit Year = %TY",d); } }OutputCurrent date and time = 26/11/2018 11:56:26 AM Four-digit Year = 2018

351 Views
Use the ‘C’ date conversion character to display two digits of year −System.out.printf("Two-digit Year (Century Name) = %tC/%TC", d, d);Above, d is a date object −Date d = new Date();The following is an example −Example Live Demoimport java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] args) throws Exception { Date d = new Date(); DateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy hh:mm:ss a"); String format = dateFormat.format(d); System.out.println("Current date and time = " + format); System.out.printf("Localized day name = %tA/%TA", d, d); ... Read More

223 Views
Use the ‘a’ date conversion character to display short day-in-week.System.out.printf("Localized short day name = %ta/%Ta", d, d);Above, d is a date object −Date d = new Date();The following is an example −Example Live Demoimport java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] args) throws Exception { Date d = new Date(); DateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy hh:mm:ss a"); String format = dateFormat.format(d); System.out.println("Current date and time = " + ... Read More

141 Views
The following is an example.Example Live Demoimport java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] args) throws Exception { Date d = new Date(); DateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy hh:mm:ss a"); String format = dateFormat.format(d); System.out.println("Current date and time = " + format); System.out.printf("Localized day name = %tA/%TA", d, d); } }OutputCurrent date and time = 26/11/2018 11:44:44 AM Localized day name = Monday/MONDAY

1K+ Views
Set width in output as shown below. Here, %10 sets a 10 character fieldSystem.out.printf("d1 = %10.2f d2 = %8g", d1, d2);Above, the value after the decimal is for decimal places i.e. 5.3 is for 3 decimal places −System.out.printf("d1 = %5.3f d2 = %2.5f", d1, d2);The following is an example −Example Live Demopublic class Demo { public static void main(String[] args) throws Exception { double d1 = 399.8877; double d2 = 298.87690; System.out.printf("d1 = %10.2f d2 = %8g", d1, d2); System.out.printf("d1 = %5.3f d2 = %2.5f", d1, d2); } }Outputd1 = 399.89 d2 = 298.877 d1 = 399.888 d2 = 298.87690

87 Views
First, we have taken a double variable and displayed it twice.double d = 399.8877; System.out.printf("d1 = %2$f d2 = %1$g", d, d);After that, we have formatted numerical int data −int val1 = 90, val2 = 35, val3 = 88, val4 = 600; System.out.printf("val1 = %d, val2 = %x, val3 = %o, val4 = %d", val1, val2, val3, val4); System.out.printf("val2 = %4$d, val2 = %3$d, val3 = %2$o, val4 = %1$d", val1, val2, val3, val4);Above, we have displayed %o for octal, %x for hexadecimal, %d for integer, etc.The following is the complete example −Example Live Demopublic class Demo { public static ... Read More

284 Views
To format year in yyyy format is like displaying the entire year. For example, 2018.Use the yyyy format like this −SimpleDateFormat("yyyy");Let us see an example −// year in yyyy format SimpleDateFormat simpleformat = new SimpleDateFormat("yyyy"); String strYear = simpleformat.format(new Date()); System.out.println("Current Year = "+strYear);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time ... Read More

983 Views
To format year in yy format is like displaying year as 01, 02, 03, 04, etc. For example, 18 for 2018.Use the yy format like this.SimpleDateFormat("yy");Let us see an example −// year in yy format SimpleDateFormat simpleformat = new SimpleDateFormat("yy"); String strYear = simpleformat.format(new Date()); System.out.println("Current Year = "+strYear);Above, we have used the SimpleDateFormat class, therefore the following package is imported −import java.text.SimpleDateFormat;The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current ... Read More