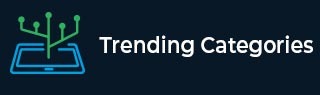
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

21K+ Views
Before converting a String to UTF-8-bytes, let us have a look at UTF-8.UTF-8 is a variable width character encoding. UTF-8 has ability to be as condense as ASCII but can also contain any unicode characters with some increase in the size of the file. UTF stands for Unicode Transformation Format. The '8' signifies that it allocates 8-bit blocks to denote a character. The number of blocks needed to represent a character varies from 1 to 4.In order to convert a String into UTF-8, we use the getBytes() method in Java. The getBytes() method encodes a String into a sequence of ... Read More

5K+ Views
Before moving onto their conversions, let us learn about Unicode and UTF-8.Unicode is an international standard of character encoding which has the capability of representing a majority of written languages all over the globe. Unicode uses hexadecimal to represent a character. Unicode is a 16-bit character encoding system. The lowest value is \u0000 and the highest value is \uFFFF.UTF-8 is a variable width character encoding. UTF-8 has the ability to be as condense as ASCII but can also contain any unicode characters with some increase in the size of the file. UTF stands for Unicode Transformation Format. The '8' signifies ... Read More

11K+ Views
Before moving onto their conversions, let us learn about Unicode and UTF-8.Unicode is an international standard of character encoding which has the capability of representing a majority of written languages all over the globe. Unicode uses hexadecimal to represent a character. Unicode is a 16-bit character encoding system. The lowest value is \u0000 and the highest value is \uFFFF.UTF-8 is a variable width character encoding. UTF-8 has the ability to be as condensed as ASCII but can also contain any Unicode characters with some increase in the size of the file. UTF stands for Unicode Transformation Format. The '8' signifies ... Read More

159 Views
In order to reverse the sequence of characters in a StringBuffer Object, we use the reverse() method. The reverse() method replaces the character sequence with the reverse of its own.Declaration − The java.lang.StringBuffer.reverse method is declared as follows−public StringBuffer reverse()Let us see a program to reverse the sequence of characters in a StringBuffer Object using the reverse() method.Example Live Demopublic class Example { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Hello World"); System.out.println("Original StringBuffer Object: " + sb); sb.reverse(); System.out.println("New StringBuffer Object: " +sb); ... Read More

9K+ Views
In order to remove a substring from a Java StringBuilder Object, we use the delete() method. The delete() method removes characters in a range from the sequence. The delete() method has two parameters, start, and end. Characters are removed from start to end-1 index.Declaration − The java.lang.StringBuilder.delete() method is declared as follows −public StringBuilder delete(int start, int end)Let us see an example which removes a substring from a StringBuilder.Example Live Demopublic class Example { public static void main(String[] args) { StringBuilder sb = new StringBuilder("Welcome to Java Programming"); System.out.println("Original StringBuilder Object: " + sb.toString()); ... Read More

1K+ Views
In order to remove characters in a specific range from a Java StringBuffer Object, we use the delete() method. The delete() method removes characters in a range from the sequence. The delete() method has two parameters , start and end. Characters are removed from start to end-1 index.Declaration − The java.lang.StringBuffer.delete() method is declared as follows −Let us see a program to delete characters in a specific range from a Java StringBuffer Object.Example Live Demopublic class Example { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Hello World"); System.out.println("Original StringBuffer Object: " ... Read More

321 Views
In order to remove a character from a Java StringBuffer object, we use the deleteCharAt() method. The deleteCharAt() removes the character at the specified index. The length of resultant sequence of characters of the StringBuffer object is reduced by one.Declaration − The java.lang.StringBuffer.deleteCharAt() method is declared as follows−public StringBuffer deleteCharAt(int index)Let us see a program to illustrate the use of the deleteCharAt()Example Live Demopublic class Example { public static void main(String[] args) { StringBuffer sb = new StringBuffer("Hello World"); sb.deleteCharAt(7); System.out.println(sb); } }OutputHello Wrld

158 Views
To get the arc sine of a given value in Java, we use the java.lang.Math.asin() method. The asin() method accepts a double value whose angle needs to be computed. The range of the angle returned lies in the range -pi/2 to pi/2. If the argument is NaN, then the result is NaN.When the argument is zero, then the output is a zero with the same sign as the argument.Declaration − The java.lang.Math.asin() method is declared as follows−public static double asin(double a)where a is the value whose arc sine is computed.Let us see a program to get the arc sine of ... Read More

1K+ Views
In order to change a single character in a StringBuffer object in Java, we use the setCharAt() method. The setCharAt() method sets the character at the index specified as a parameter to another character whose value is passed parameter of the setCharAt() method. The method sets a new character sequence with the only change being the character passed as the parameter at the specified index.Declaration - The java.lang.StringBuffer.setCharAt() method is declared as follows−public void setCharAt(int index, char ch)If index is greater than the length of the StringBuffer object or is negative, an IndexOutOfBoundsException is generated.Let us see a program to ... Read More

124 Views
To display the position of a Substring in Java, we use the lastindexOf() method in Java. The lastindexOf() method returns the rightmost occurrence of the substring within a particular StringBuffer object.If the substring occurs more than once in the StringBuffer object, the index of the first character of the rightmost occured substring is returned. If the substring is not found, the lastIndexOf() method returns -1.Declaration − The java.lang.StringBuffer.lastIndexOf() method is declared as follows−public int lastIndexOf(String s)where s is the substring whose index needs to be foundLet us see a program which displays the position of a Substring.Example Live Demopublic class Example ... Read More