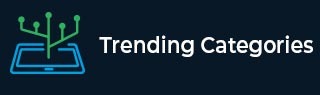
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

867 Views
In fixed-delay execution, each execution is scheduled with respect to the original execution time of the preceding execution. If an execution is delayed for a particular reason (case in point, garbage collection), the subsequent executions will be delayed as well.There are two ways in which a task can be scheduled for repeated fixed-delay execution. They are as follows −Scheduling a task for repeated fixed-delay execution at a specified timeScheduling a task for repeated fixed-delay execution after a specified delayScheduling a task for repeated fixed-delay execution at a specified timeThe void schedule(TimerTask task, Date firstTime, long period) method schedules tasks for ... Read More

447 Views
One of the methods in the Timer class is the void schedule(TimerTask task, Date firstTime, long period) method. This method schedules tasks for repeated fixed-delay execution, beginning at the specified time.In fixed-delay execution, each execution is scheduled with respect to the original execution time of the preceding execution. If an execution is delayed for a particular reason (case in point, garbage collection), the subsequent executions will be delayed as well.Declaration −The java.util.Timer.schedule(TimerTask task, Date firstTime, long period) is declared as follows −public void schedule(TimerTask task, Date firstTime, long period)Here, task is the task to be scheduled, firstTime is the first ... Read More

1K+ Views
One of the methods in the Timer class is the void schedule(Timertask task, long delay) method. This method schedules the specified task for execution after the specified delay.Declaration −The java.util.Timer.schedule(Timertask task, long delay) is declared as follows −public void schedule(Timertask task, long delay)There are few exceptions thrown by the schedule(Timertask task, long delay) method. They are as follows −IllegalArgumentExceptionThis exception is thrown if delay is negative, or delay + System.currentTimeMillis() is negative.IllegalStateExceptionThis exception is thrown if task was scheduled or cancelled beforehand, timer was cancelled, or timer thread terminated.NullPointerExceptionThis exception is thrown if the task is null.Let us see a ... Read More

5K+ Views
One of the methods in the Timer class is the void schedule(Timertask task, Date time) method. This method schedules the specified task for execution at the specified time. If the time is in the past, it schedules the task for immediate execution.Declaration −The java.util.Timer.schedule(Timertask task, Date time) is declared as follows −public void schedule(Timertask task, Date time)There are few exceptions thrown by the schedule(Timertask task, Date time) method. They are as follows −IllegalArgumentExceptionThis exception is thrown if time.getTime() is negativeIllegalStateExceptionThis exception is thrown if task was scheduled or cancelled beforehand, timer was cancelled, or timer thread terminated.NullPointerExceptionThis exception is thrown ... Read More

263 Views
One of the methods of the Timer class is the int purge() method. The purge() method removes all the canceled tasks from the timer’s task queue. Invoking this method does not affect the behavior of the timer, rather it eliminates references to the canceled tasks from the queue. The purge() method came into existence since JDK 1.5.The purge() method acts as a medium for space-time tradeoff where it trades time for space. More specifically, the time complexity of the method is proportional to n + c log n, where n is the number of tasks in the queue and c ... Read More

1K+ Views
One of the methods of the Timer class is the cancel() method. It is used to terminate the current timer and get rid of any presently scheduled tasks.The java.util.Timer.cancel() method is declared as follows −public void cancel()Let us see a program which uses the cancel() methodExample Live Demoimport java.util.*; public class Example { Timer t; public Example(int seconds) { t = new Timer(); t .schedule(new Running(), seconds); } class Running extends TimerTask { public void run() { System.out.println("Task is cancelled"); ... Read More

414 Views
The Timer Class in Java is a facility for threads to plan tasks for future execution in a background thread. Tasks may be executed a single time or multiple times. The Timer class is thread-safe i.e. the threads of the class do not require external synchronization and can share a single Timer object. A point to be noted is that all constructors start a Timer thread.The Timer Class in Java came into existence since JDK 1.3. This class ascends up to large numbers of concurrently scheduled tasks. Internally, it uses a binary heap in the memory to represent its task ... Read More

148 Views
To get the arc cosine of a given value in Java, we use the java.lang.Math.acos() method. The acos() method accepts a double value whose angle needs to be computed. The range of the angle returned lies in the range 0 to pi. If the argument is NaN or greater than 1 or less than -1 then the result is NaN.Declaration −The java.lang.Math.acos() method is declared as follows −public static double acos(double a)Here, a is the value whose arc cosine is computed.Let us see a program to get the arc cosine of a given value in Java.Example Live Demoimport java.lang.Math; public class ... Read More

110 Views
To get the arc tangent of a given value in Java, we use the java.lang.Math.atan() method. The atan() method accepts a double value whose angle needs to be computed. The range of the angle returned lies in the range -pi/2 to pi/2. If the argument is NaN, then the result is NaN.When the argument is zero, then the output is a zero with the same sign as the argument.Declaration −The java.lang.Math.atan() method is declared as follows −public static double atan(double a)where a is the value whose arc tangent is computed.Let us see a program to get the arc tangent of ... Read More

3K+ Views
In order to generate Random float type numbers in Java, we use the nextFloat() method of the java.util.Random class. This returns the next random float value between 0.0 (inclusive) and 1.0 (exclusive) from the random generator sequence.Declaration −The java.util.Random.nextFloat() method is declared as follows −public float nextFloat()Let us see a program to generate random float type numbers in Java.Example Live Demoimport java.util.Random; public class Example { public static void main(String[] args) { Random rd = new Random(); // creating Random object System.out.println(rd.nextFloat()); // displaying a random float value between 0.0 and 1.0 } ... Read More