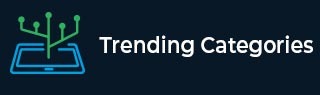
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

58 Views
In order to get a copy of a TimeZone object in Java, we use the clone() method. The clone() method creates of a copy of the TimeZone.Declaration −The java.util.TimeZone.clone() method is declared as follows −public Object clone()Let us see a Java program which creates a copy of the TimeZone object using the clone() method −Example Live Demoimport java.util.*; public class Example { public static void main( String args[] ) { // creating object of TimeZone TimeZone obj = TimeZone.getDefault(); System.out.println("Initial object: " + obj); // copying the TimeZone ... Read More

12K+ Views
In order to generate Random boolean in Java, we use the nextBoolean() method of the java.util.Random class. This returns the next random boolean value from the random generator sequence.Declaration −The java.util.Random.nextBoolean() method is declared as follows −public boolean nextBoolean()Let us see a program to generate random boolean in Java −Example Live Demoimport java.util.Random; public class Example { public static void main(String[] args) { Random rd = new Random(); // creating Random object System.out.println(rd.nextBoolean()); // displaying a random boolean } }OutputtrueNote − The output might vary on Online Compilers.

208 Views
To get the elapsed time of an operation in days in Java, we use the System.currentTimeMillis() method. The java.lang.System.currentTimeMillis() returns the current time in milliseconds.Declaration −The java.lang.System.currentTimeMillis() is declared as follows −public static long currentTimeMillis()The method returns time difference in milliseconds between the current time and midnight, January 1, 1970 (UTC or epoch time).Let us see a program to compute the elapsed time of an operation in days in Java −Example Live Demopublic class Example { public static void main(String[] args) throws Exception { // finding the time before the operation is executed long ... Read More

368 Views
To get the elapsed time of an operation in hours in Java, we use the System.currentTimeMillis() method. The java.lang.System.currentTimeMillis() returns the current time in milliseconds.Declaration −The java.lang.System.currentTimeMillis() is declared as follows −public static long currentTimeMillis()The method returns time difference in milliseconds between the current time and midnight, January 1, 1970 (UTC or epoch time).Let us see a program to compute the elapsed time of an operation in hours in Java −Example Live Demopublic class Example { public static void main(String[] args) throws Exception { // finding the time before the operation is executed long ... Read More

2K+ Views
To get the elapsed time of an operation in minutes in Java, we use the System.currentTimeMillis() method. The java.lang.System.currentTimeMillis() returns the current time in milliseconds.Declaration −The java.lang.System.currentTimeMillis() is declared as follows −public static long currentTimeMillis()The method returns time difference in milliseconds between the current time and midnight, January 1, 1970 (UTC or epoch time).Let us see a program to compute the elapsed time of an operation in minutes in Java −Example Live Demopublic class Example { public static void main(String[] args) throws Exception { // finding the time before the operation is executed long ... Read More

162 Views
To compute the elapsed time of an operation in Java, we use the System.currentTimeMillis() method. The java.lang.System.currentTimeMillis() returns the current time in milliseconds.Declaration −The java.lang.System.currentTimeMillis() is declared as follows −public static long currentTimeMillis()The method returns time difference in milliseconds between the current time and midnight, January 1, 1970 (UTC or epoch time).Let us see a program to compute the elapsed time of an operation in Java −Example Live Demopublic class Example { public static void main(String[] args) throws Exception { // finding the time before the operation is executed long start = System.currentTimeMillis(); ... Read More

11K+ Views
To compute the elapsed time of an operation in seconds in Java, we use the System.currentTimeMillis() method. The java.lang.System.currentTimeMillis() returns the current time in milliseconds.Declaration −The java.lang.System.currentTimeMillis() is declared as follows −public static long currentTimeMillis()The method returns time difference in milliseconds between the current time and midnight, January 1, 1970 (UTC or epoch time).Let us see a program to compute the elapsed time of an operation in Java −Example Live Demopublic class Example { public static void main(String[] args) throws Exception { // finding the time before the operation is executed long start = ... Read More

318 Views
Two int arrays can be compared in Java using the java.util.Arrays.equals() method. This method returns true if the arrays are equal and false otherwise. The two arrays are equal if they contain the same number of elements in the same order.A program that compares two int arrays using the Arrays.equals() method is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { boolean flag = Arrays.equals(new int[] { 45, 12, 90 }, new int[] { 45, 12, 90 }); System.out.println("The two int arrays are equal? ... Read More

634 Views
The java.util.TimerTask.run() method looks onto the action to be performed by the task. It is used to carry out the action performed by the task.Declaration −The java.util.TimerTask.run() method is declared as follows −public abstract void run()Let us see an example program to call the run() method of the Timer Task −Example Live Demoimport java.util.*; class MyTask extends TimerTask { public void run() { System.out.println("Running"); } } public class Example { public static void main(String[] args) { // creating timer task, timer TimerTask task = new MyTask(); ... Read More

5K+ Views
In order to cancel the Timer Task in Java, we use the java.util.TimerTask.cancel() method. The cancel() method returns a boolean value, either true or false. The cancel() method is used to cancel the timer task.Declaration −The java.util.TimerTask.cancel() method is declared as follows −public boolean cancel()The cancel() methods returns true when the task is scheduled for one-time execution and has not executed until now and returns false when the task was scheduled for one-time execution and has been executed already.Let us see a program to illustrate the use of the java.util.TimerTask.cancel() method −Example Live Demoimport java.util.*; class MyTask extends TimerTask { ... Read More