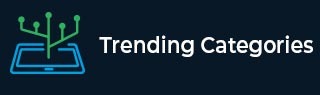
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

6K+ Views
In order to handle the assertion error, we need to declare the assertion statement in the try block and catch the assertion error in the catch block.Let us see a program on how to handle Assertion Error in Java −Examplepublic class Example { public static void main(String[] args) throws Exception { try { assert args.length > 0; } catch (AssertionError e) { System.out.println(e.getMessage()); } } }Output

402 Views
By default, assertions are disabled in Java. In order to enable them we use the following command −java -ea Example (or) java -enableassertions ExampleHere, Example is the name of the Java file.Let us see an example for generation of an assertion error by the JVM −Example Live Demopublic class Example { public static void main(String[] args) { int age = 14; assert age >= 18 : "Cannot Vote"; System.out.println("The voter's age is " + age); } }OutputThe voter's age is 14

532 Views
In order to compile assert in Java, we simply set the boolean expression as false.Let us see an example program −Example Live Demopublic class Example { public static void main(String[] args) { assert false; System.out.println("Compiled and executed successfully!!!"); } }OutputCompiled and executed successfully!!!

6K+ Views
An assertion is a statement in Java which ensures the correctness of any assumptions which have been done in the program. When an assertion is executed, it is assumed to be true. If the assertion is false, the JVM will throw an Assertion error. It finds it application primarily in the testing purposes. Assertion statements are used along with boolean expressions.Assertions in Java can be done with the help of the assert keyword. There are two ways in which an assert statement can be used.First Way −assert expression;Second Way −assert expression1 : expression2By default, assertions are disabled in Java. In ... Read More

3K+ Views
In order to get the name of the Thread, we use the getName() method in Java. This method returns the name of the thread.Declaration − The java.lang.Thread.getName() method is declared as follows −public String getName()In order to get the identity of the Thread, we use the getId() method in Java. This method returns the identifier of the thread. The thread ID is a positive long number produced during the creation of the thread.Declaration − The java.lang.Thread.getId() method is declared as follows −public long getId()In order to get the state of the Thread, we use the getState() method in Java. This ... Read More

125 Views
To format message with short length format for date in Java, we use the MessageFormat class and the Date class. The MessageFormat class gives us a way to produce concatenated messages which are not dependent on the language. The MessageFormat class extends the Serializable and Cloneable interfaces.Declaration − The java.text.MessageFormat class is declared as follows −public class MessageFormat extends FormatThe MessageFormat.format(pattern, params) method formats the message and fills in the missing parts using the objects in the params array matching up the argument numbers and the array indices.The format method has two arguments, a pattern and an array of arguments. ... Read More

196 Views
Elements can be filled in a float array using the java.util.Arrays.fill() method. This method assigns the required float value to the float array in Java. The two parameters required are the array name and the value that is to be stored in the array elements.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { float[] floatArray = new float[5]; float floatValue = 8.5F; Arrays.fill(floatArray, floatValue); System.out.println("The float array content is: " + Arrays.toString(floatArray)); ... Read More

116 Views
To format message with medium style format of date in Java, we use the MessageFormat class and the Date class. The MessageFormat class gives us a way to produce concatenated messages which are not dependent on the language. The MessageFormat class extends the Serializable and Cloneable interfaces.Declaration − The java.text.MessageFormat class is declared as follows −public class MessageFormat extends FormatThe MessageFormat.format(pattern, params) method formats the message and fills in the missing parts using the objects in the params array matching up the argument numbers and the array indices.The format method has two arguments, a pattern and an array of arguments. ... Read More

610 Views
Binary search on a char array can be implemented by using the method java.util.Arrays.binarySearch(). This method returns the index of the required char element if it is available in the array, otherwise, it returns (-(insertion point) - 1) where the insertion point is the position at which the element would be inserted into the array.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] args) { char c_arr[] = { 'b', 's', 'l', 'e', 'm' }; Arrays.sort(c_arr); System.out.print("The sorted array ... Read More

177 Views
To format message with long style format of date in Java, we use the MessageFormat class and the Date class. The MessageFormat class gives us a way to produce concatenated messages which are not dependent on the language. The MessageFormat class extends the Serializable and Cloneable interfaces.Declaration − The java.text.MessageFormat class is declared as follows −public class MessageFormat extends FormatThe MessageFormat.format(pattern, params) method formats the message and fills in the missing parts using the objects in the params array matching up the argument numbers and the array indices.The format method has two arguments, a pattern and an array of arguments. ... Read More