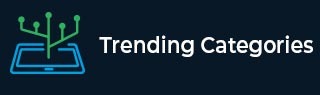
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

3K+ Views
To replace all words with another String using Java Regular Expressions, we need to use the replaceAll() method. The replaceAll() method returns a String replacing all the character sequence matching the regular expression and String after replacement.Declaration − The java.lang.String.replaceAll() method is declared as follows −public String replaceAll(String regex, String replaced) Let us see a program to replace all words with another string with Java Regular Expressions −Example Live Demopublic class Example { public static void main( String args[] ) { String str = new String("Good Harry Good"); System.out.println( "Initial String : "+ str); ... Read More

7K+ Views
To replace one string with another string using Java Regular Expressions, we need to use the replaceAll() method. The replaceAll() method returns a String replacing all the character sequence matching the regular expression and String after replacement.Declaration − The java.lang.String.replaceAll() method is declared as follows −public String replaceAll(String regex, String replaced) Let us see a program to replace one string with another string using Java Regular Expressions −Example Live Demopublic class Example { public static void main( String args[] ) { String str = new String("Good Harry Good"); System.out.println( "Initial String : "+ str); ... Read More

2K+ Views
To replace *' with '^' using Java Regular Expressions, we need to use the replaceAll() method. The replaceAll() method returns a String replacing all the character sequence matching the regular expression and String after replacement.Declaration − The java.lang.String.replaceAll() method is declared as follows −public String replaceAll(String regex, String replaced) Let us see a program to replace '*' with '^' using Java Regular Expressions −Example Live Demopublic class Example { public static void main( String args[] ) { String str = new String("H*e*l*l*o"); System.out.println( "Initial String : "+ str); // replacing '*' ... Read More

288 Views
In order to match the phone using regular expression, we use the matches method in Java. The java.lang.String.matches() method returns a boolean value which depends on the matching of the String with the regular expression.Declaration − The java.lang.String.matches() method is declared as follows −public boolean matches(String regex)Let us see a program to validate a phone number with regular expressions −Example Live Demopublic class Example { public static void main( String[] args ) { System.out.println(phone("+91 1023456789")); } // validate zip public static boolean phone( String z ) { return z.matches("\+[0-9]*\s+\d{10}" ); ... Read More

175 Views
Binary search on a long array can be implemented by using the method java.util.Arrays.binarySearch(). This method returns the index of the required long element if it is available in the array, otherwise it returns (-(insertion point) - 1) where the insertion point is the position at which the element would be inserted into the array.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] args) { long long_arr[] = { 250L, 500L, 175L, 90L, 415L }; Arrays.sort(long_arr); System.out.print("The sorted array ... Read More

383 Views
To format message with currency fillers in Java, we use the MessageFormat class. The MessageFormat class gives us a way to produce concatenated messages which are not dependent on the language. The MessageFormat class extends the Serializable and Cloneable interfaces.Declaration −The java.text.MessageFormat class is declared as follows −public class MessageFormat extends FormatThe MessageFormat.format(pattern, params) method formats the message and fills in the missing parts using the objects in the params array matching up the argument numbers and the array indices.The format method has two arguments, a pattern and an array of arguments. The pattern contains placeholder in {} curly braces ... Read More

815 Views
To format message with floating point fillers in Java, we use the MessageFormat class. The MessageFormat class gives us a way to produce concatenated messages which are not dependent on the language. The MessageFormat class extends the Serializable and Cloneable interfaces.Declaration −The java.text.MessageFormat class is declared as follows −public class MessageFormat extends FormatThe MessageFormat.format(pattern, params) method formats the message and fills in the missing parts using the objects in the params array matching up the argument numbers and the array indices.The format method has two arguments, a pattern and an array of arguments. The pattern contains placeholder in {} curly ... Read More

354 Views
In order to match the ZIP code using regular expression, we use the matches method in Java. The java.lang.String.matches() method returns a boolean value which depends on the matching of the String with the regular expression.Declaration − The java.lang.String.matches() method is declared as follows −public boolean matches(String regex)Let us see a program which validates the ZIP code with Java Regular Expressions −Example Live Demopublic class Example { public static void main( String[] args ) { System.out.println(zipIndia("400709")); System.out.println(zipUS("10060")); } // validate zip public static boolean zipIndia( String z ) { ... Read More

837 Views
In order to match the city and state using regular expression, we use the matches method in Java. The java.lang.String.matches() method returns a boolean value which depends on the matching of the String with the regular expression.Declaration − The java.lang.String.matches() method is declared as follows −Example Live Demopublic class Example { public static void main( String[] args ) { System.out.println(city("Mumbai")); System.out.println(state("Goa")); } // validating the city public static boolean city( String c ) { return c.matches( "([a - zA - Z] + |[a - zA - Z] + ... Read More

6K+ Views
In order to catch the assertion error, we need to declare the assertion statement in the try block with the second expression being the message to be displayed and catch the assertion error in the catch block.Let us see a program on how to handle Assertion Error in Java −Examplepublic class Example { public static void main(String[] args) throws Exception { try { assert args.length > 0 : "Assertion error caught!!!!"; } catch (AssertionError e) { System.out.println(e.getMessage()); } } }Output