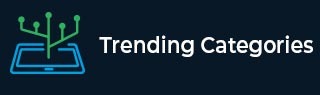
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

6K+ Views
In order to generate Random Integer Numbers in Java, we use the nextInt() method of the java.util.Random class. This returns the next random integer value from this random number generator sequence.Declaration − The java.util.Random.nextInt() method is declared as follows −public int nextInt()Let us see a program to generate random integer numbers in Java −Example Live Demoimport java.util.Random; public class Example { public static void main(String[] args) { Random rd = new Random(); // creating Random object System.out.println(rd.nextInt()); } }Output27100093Note - The output might vary on Online Compilers.

4K+ Views
In order to get the number of available processors in Java, we use the availableProcessors() method. The java.lang.Runtime.availableProcessors() method returns the number of processors which are available to the Java virtual machine. This number may vary during a particular call of the virtual machine.Declaration − The java.lang.Runtime.availableProcessors() method is declared as follows −public int availableProcessors()Let us see a program to get the number of available processors in Java −Example Live Demopublic class Example { public static void main(String[] args) { // print statement at the start of the program System.out.println("Start..."); System.out.print("Number ... Read More

23K+ Views
The getProperty(String key) method in Java is used to returns the system property denoted by the specified key passed as its argument.It is a method of the java.lang.System Class.Declaration − The java.lang.System.getProperty(String key) is declared as follows −public static String getProperty(String key)where key is the name of the System property.Some values of the key are as follows −file.separatorjava.specification.versionjava.vm.versionjava.class.pathjava.vendorjava.class.versionos.archjava.compilerline.separatorjava.versionjava.vendor.urlos.nameLet us see a program showing the use of getProperty() method in Java −Example Live Demopublic class Example { public static void main(String[] args) { System.out.println("System property: " + System.getProperty("user.dir")); System.out.println("Operating System: " + System.getProperty("os.name")); ... Read More

320 Views
In order to display the amount of free memory in the Java Virtual Machine, we use the freeMemory() method. It is a method of the java.lang.Runtime Class. It returns the amount of free memory in the Java Virtual Machine. If we call the gc method, there is a possibility of increase of free memory.Declaration − The java.lang.Runtime.freeMemory() method is declared as follows −public long freeMemory()Let us see a program to display the amount of free memory in the Java Virtual Machine −Example Live Demopublic class Example { public static void main(String[] args) { // print statement at ... Read More

214 Views
In order to display the maximum amount of memory in Java, we use the maxMemory() method. It is a method of the java.lang.Runtime Class. It returns the maximum amount of memory that the Java Virtual Machine will try to use.Declaration − The java.lang.Runtime.maxMemory() method is declared as follows −public long maxMemory()Let us see a program to display the maximum amount of memory in Java −Example Live Demopublic class Example { public static void main(String[] args) { // print statement at the start of the program System.out.println("Start..."); // displays the maximum memory ... Read More

501 Views
In order to determine the existence a preference node in Java, we use the nodeExists() method. The nodeExists() method returns a boolean value. It returns true when the specified preference node exists in the same tree as this node.Declaration − The java.util.prefs.Preferences.remove() method is declared as follows −public abstract boolean nodeExists(String pathname)throws BackingStoreExceptionwhere pathname is the path name of the node whose existence needs to be determined.Let us see a program to determine if a preference node exists in Java −Example Live Demoimport java.util.prefs.Preferences; public class Example { public static void main(String[] args) throws Exception { boolean ... Read More

484 Views
In order to export preferences to an XML file in Java, we need to use the exportSubtree() method. This method emits an XML document showing all of the preferences contained in this node and all of its descendants. This XML document provides an offline backup of the subtree rooted at the node.Declaration − The java.util.prefs.Preferences.exportSubtree method is declared as follows −public abstract void exportSubtree(OutputStream os) throws IOException, BackingStoreExceptionLet us see a program to export preferences to an XML file in Java −import java.io.FileOutputStream; import java.util.prefs.Preferences; public class Example { public static void main(String args[]) throws Exception { Preferences ... Read More

154 Views
Elements can be filled in a short array using the java.util.Arrays.fill() method. This method assigns the required short value to the short array in Java. The two parameters required are the array name and the value that is to be stored in the array elements.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { short[] shortArray = new short[5]; short shortValue = 1; Arrays.fill(shortArray, shortValue); System.out.println("The short array content is: " + Arrays.toString(shortArray)); ... Read More

321 Views
In order to remove a preference from a preference node in Java, we use the remove() method. The remove method() removes all the values associated with the specified key in the preference node.Declaration − The java.util.prefs.Preferences.remove() method is declared as follows −public abstract void remove (String key)where key is the key whose preference is to be removedThe remove methods throws the following exceptions −NullPointerExceptionThis exception occurs when the key is nullIllegalStateExceptionThis exception is thrown when the ancestor node is removed by the removeNode() method.Let us see a program to remove the preference from a preference node −Example Live Demoimport java.util.prefs.Preferences; public ... Read More

561 Views
An array of field objects is returned by the method java.lang.Class.getFields(). These field objects include the accessible public fields of the class that is represented by the class object.Also, the getFields() method returns a zero length array if the class or interface has no public fields that are accessible or if a primitive type, array class or void is represented in the Class object.A program that demonstrates this is given as follows −Example Live Demoimport java.lang.reflect.*; public class Demo { public static void main(String[] argv) throws Exception { Class c = java.lang.Thread.class; Field[] fields ... Read More