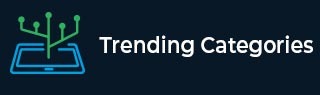
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
In order to reverse order of all elements of ArrayList with Java Collections, we use the Collections.reverse() method.Declaration −The java.util.Collections.reverse method is declared as follows −public static void reverse(List list)Let us see a program to reverse order of all elements of ArrayList with Java Collections −Example Live Demoimport java.util.*; public class Example { public static void main (String[] args) { ArrayList list = new ArrayList(); list.add(1); list.add(2); list.add(7); list.add(8); list.add(3); list.add(9); System.out.println("Original list : " + ... Read More

1K+ Views
In order to replace all occurrences of specified element of ArrayList with Java Collections, we use the Collections.replaceAll() method. This method returns true if list contains one or more elements e such that (oldVal==null ? e==null : oldVal.equals(e)).Declaration −The java.util.Collections.replaceAll() is declared as follows −public static boolean replaceAll(List list, T oldVal, T newVal)where oldVal is the element value in the list to be replaced, newVal is the element value with which it is replaced and list is the list in which replacement takes place.Let us see a program to replace all occurrences of specified element of ArrayList with Java ... Read More

2K+ Views
In order to replace all elements of ArrayList with Java Collections, we use the Collections.fill() method. The static void fill(List list, Object element) method replaces all elements in the list with the specified element in the argument.Declaration −The java.util.Collections.fill() method is declared as follows −public static void fill(List

1K+ Views
In order to perform Binary Search on ArrayList with Java Collections, we use the Collections.binarySearch() method.Declaration −The java.util.Collections.binarySearch() method is declared as follows −public static int binarySearch(List list, T key)The above method returns the position of the key in the list sorted in ascending order. If we use a Comparator c to sort the list, the binarySearch() method will be declared as follows −public static int binarySearch(List list, T key, Comparator c)If key is not present, the it returns ((insertion point) + 1) *(-1).Let us see a program to perform binarySearch() on ArrayList −Example Live Demoimport java.util.*; public class Example { ... Read More

241 Views
Two short arrays can be compared in Java using the java.util.Arrays.equals() method. This method returns true if the arrays are equal and false otherwise. The two arrays are equal if they contain the same number of elements in the same order.A program that compares two short arrays using the Arrays.equals() method is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { boolean flag = Arrays.equals(new short[] { 2, 8, 5 }, new short[] { 2, 8, 5 }); System.out.println("The two short arrays are equal? ... Read More

444 Views
In order to get enumeration over ArrayList with Java Collections, we use the java.util.Collections.enumeration() method.Declaration −The java.util.Collections.enumeration() method is declared is as follows −public static Enumeration enumeration(Collection c)where c is the collection object for which an enumeration is returnedLet us see a program to get enumeration over ArrayList −Example Live Demoimport java.util.*; public class Example { public static void main (String[] args) { ArrayList list = new ArrayList(); list.add(14); list.add(2); list.add(73); Enumeration en = Collections.enumeration(list); // getting enumeration over ArrayList list ... Read More

3K+ Views
In order to compute minimum element of ArrayList with Java Collections, we use the Collections.min() method. The java.util.Collections.min() returns the minimum element of the given collection. All elements must be mutually comparable and implement the comparable interface. They shouldn’t throw a ClassCastException.Declaration −The Collections.min() method is declared as follows −public static T min(Collection c)where c is the collection object whose minimum is to be found.Let us see a program to find the minimum element of ArrayList with Java collections −Example Live Demoimport java.util.*; public class Example { public static void main (String[] args) { List list ... Read More

8K+ Views
In order to copy elements of ArrayList to another ArrayList, we use the Collections.copy() method. It is used to copy all elements of a collection into another.Declaration −The java.util.Collections.copy() method is declared as follows −public static void copy(List

289 Views
In order to copy elements of ArrayList to Java Vector, we use the Collections.copy() method. It is used to copy all elements of a collection into another.Declaration −The java.util.Collections.copy() method is declared as follows −public static void copy(List

4K+ Views
In order to sort elements in an ArrayList in Java, we use the Collections.sort() method in Java. This method sorts the elements available in the particular list of the Collection class in ascending order.Declaration −The java.util.Collections.sort() method is declared as follows −public static void sort(List list)where list is an object on which sorting needs to be performed.Let us see a program to sort elements in an ArrayList in Java −Example Live Demoimport java.util.*; public class Example { public static void main (String[] args) { List zoo = new ArrayList(); zoo.add("Zebra"); zoo.add("Lion"); ... Read More