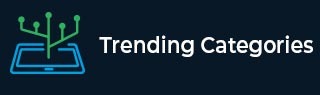
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

928 Views
A new instance of a two dimensional array can be created using the java.lang.reflect.Array.newInstance() method. This method basically creates the two-dimensional array with the required component type as well as length.A program that demonstrates the creation of a two-dimensional array using the Array.newInstance() method is given as follows −Example Live Demoimport java.lang.reflect.Array; public class Demo { public static void main (String args[]) { int size[] = {3, 3}; int arr[][] = (int[][])Array.newInstance(int.class, size); System.out.println("The two-dimensional array is:"); for(int[] i: arr) { for(int j: i ... Read More

5K+ Views
A fully-qualified class name in Java contains the package that the class originated from. Also, an inner class is a class that is another class member. So, the fully-qualified name of an inner class can be obtained using the getName() method.A program that demonstrates this is given as follows −Example Live Demopublic class Main { public static void main(String[] argv) throws Exception { Class c = java.lang.Character.Subset.class; String innerClassName = c.getName(); System.out.println("The fully qualified name of the inner class is: " + innerClassName); } }OutputThe fully qualified name of the ... Read More

9K+ Views
A fully-qualified class name in Java contains the package that the class originated from. An example of this is java.util.ArrayList. The fully-qualified class name can be obtained using the getName() method.A program that demonstrates this is given as follows −Example Live Demopublic class Demo { public static void main(String[] argv) throws Exception { Class c = java.util.ArrayList.class; String className = c.getName(); System.out.println("The fully-qualified name of the class is: " + className); } }OutputThe fully-qualified name of the class is: java.util.ArrayListNow let us understand the above program.The getName() method is used ... Read More

427 Views
A qualified class name in Java contains the package that the class originated from. In contrast to this, the unqualified class name contains only the class name without any package information. A program that gets the unqualified name of a class is given as follows:Example Live Demopublic class Demo { public static void main(String[] argv) throws Exception { Class c = java.util.ArrayList.class; String className = c.getName(); System.out.println("The qualified class name is: " + className); if (className.lastIndexOf('.') < 0) { className = className.substring(className.lastIndexOf('.') + 1); ... Read More

4K+ Views
The getName() method is used to get the names of the entities such as interface, class, array class, void etc. that are represented by the class objects. These names are returned in the form of a string.A program that gets the name of the member objects using getName() method is given as follows −Example Live Demoimport java.lang.reflect.Constructor; import java.lang.reflect.Field; import java.lang.reflect.Method; public class Main { public static void main(String[] argv) throws Exception { Class c = java.lang.Integer.class; Method m = c.getMethods()[0]; Field f = c.getFields()[0]; Constructor cons = ... Read More

150 Views
The modifiers for a class or an interface are returned by the java.lang.Class.getModifiers() method. These modifiers are encoded as an integer and they consist of the JVM’s constants for public, private, protected, final, abstract, static and interface.A program that demonstrates this is given as follows −Example Live Demoimport java.lang.reflect.Modifier; public class Main { public static void main(String[] argv) throws Exception { int modifiers = String.class.getModifiers(); String modifierString = Modifier.toString(modifiers); System.out.println("The Modifier is: " + modifierString); } }OutputThe Modifier is: public finalNow let us understand the above program.The method getModifiers() is ... Read More

532 Views
The immediate superclass of any entity such as an object, class, primitive type, interface etc. can be obtained using the method java.lang.Class.getSuperclass(). This method contains no parameters.A program that demonstrates this is given as follows −Example Live Demopublic class Main { public static void main(String[] args) { Object obj1 = new String("Hello"); Object obj2 = new Integer(15); Class c1 = obj1.getClass().getSuperclass(); System.out.println("Super Class = " + c1); Class c2 = obj2.getClass().getSuperclass(); System.out.println("Super Class = " + c2); } }OutputSuper Class = ... Read More

520 Views
The availability can be checked using the method java.lang.Class.forName(). The class object associated with the class with the given string name can be returned using the method java.lang.Class.forName(String name, boolean initialize, ClassLoader loader), using the class loader that is used to load the class.A program that demonstrates this is given as follows −Example Live Demopublic class Main { public static void main(String args[]) { System.out.println(Availability("java.lang.String")); } public static boolean Availability(String name) { boolean flag = false; try { Class.forName(name, false, null); ... Read More

739 Views
The package for an object of a class can be obtained using the getPackage() method with the help of the class loader of the class. If there is no package object created by the class loader of the class, then null is returned.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.LinkedList; import java.util.HashSet; public class Main { public static void main(String[] args) { System.out.println("The package is: " + new ArrayList().getClass().getPackage().getName()); System.out.println("The package is: " + new LinkedList().getClass().getPackage().getName()); System.out.println("The package is: " + new HashSet().getClass().getPackage().getName()); ... Read More

93 Views
The package for a class can be obtained using the java.lang.Class.getPackage() method with the help of the class loader of the class.The getPackage() method returns null for a primitive or array in unnamed package. A program that demonstrates this is given as follows −Example Live Demopublic class Main { public static void main(String[] argv) throws Exception { Package pack1 = int.class.getPackage(); System.out.println(pack1); Package pack2 = int[].class.getPackage(); System.out.println(pack2); } }Outputnull nullNow let us understand the above program.The getPackage() method is used to obtain the package for the class. ... Read More