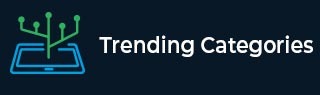
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

131 Views
public interface List extends CollectionThe List interface extends Collection and declares the behavior of a collection that stores a sequence of elements.Elements can be inserted or accessed by their position in the list, using a zero-based index.A list may contain duplicate elements.In addition to the methods defined by Collection, List defines some of its own, which are summarized in the following table.Several of the list methods will throw an UnsupportedOperationException if the collection cannot be modified, and a ClassCastException is generated when one object is incompatible with another.ExampleThe above interface has been implemented in various classes like ArrayList or LinkedList, ... Read More

8K+ Views
An ArrayList can be converted to a set object using Set constructor. The resultant set will elliminate any duplicate entry present in the list and will contains only the unique values.Set set = new HashSet(list);Or we can use set.addAll() method to add all the elements of the list to the set.set.addAll(list);Using streams as well, we can get a set from a list.set = list.stream().collect(Collectors.toSet());ExampleFollowing is the example showing the list to set conversion via multiple ways −package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.HashSet; import java.util.List; import java.util.Set; import java.util.stream.Collectors; public class CollectionsDemo { public static void ... Read More

6K+ Views
Java List provides a method indexOf() which can be used to get the location of an element in the list and contains() method to check if object is present or not.indexOf() methodint indexOf(Object o)Returns the index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element. More formally, returns the lowest index i such that (o==null ? get(i)==null : o.equals(get(i))), or -1 if there is no such index.Parameterso − Element to search for.ReturnsThe index of the first occurrence of the specified element in this list, or -1 if this ... Read More

576 Views
We can create a shallow copy of a list easily using addAll() method of List interface.Syntaxboolean addAll(Collection>? extends E> c)Appends all of the elements in the specified collection to the end of this list, in the order that they are returned by the specified collection's iterator.Type ParameterE − The runtime type of the collection passed.Parametersc − Collection containing elements to be added to this list.ReturnsTrue if this list changed as a result of the callThrowsUnsupportedOperationException − If the addAll operation is not supported by this list.ClassCastException − If the class of an element of the specified collection prevents it from ... Read More

230 Views
We can utilize listIterator() method of List interface, which allows element insertion and replacement, and bidirectional access in addition to the normal operations that the Iterator interface provides.SyntaxListIterator listIterator()Returns a list iterator over the elements in this list (in proper sequence).ExampleFollowing is the example showing the usage of listIterator() method −package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.List; import java.util.ListIterator; public class CollectionsDemo { public static void main(String[] args) throws CloneNotSupportedException { List list = new ArrayList(Arrays.asList(1, 2, 3, 4, 5)); System.out.println(list); ListIterator iterator = list.listIterator(); ... Read More

17K+ Views
indexOf() method of List is used to get the location of an element in the list.int indexOf(Object o)Returns the index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element. More formally, returns the lowest index i such that (o==null ? get(i)==null : o.equals(get(i))), or -1 if there is no such index.Parameterso − Element to search for.ReturnsThe index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element.ThrowsClassCastException − If the type of the specified element is incompatible ... Read More

1K+ Views
indexOf() method of List can be used to get the location of an element in the list.int indexOf(Object o)Returns the index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element. More formally, returns the lowest index i such that (o==null ? get(i)==null : o.equals(get(i))), or -1 if there is no such index.Parameterso − Element to search for.ReturnsThe index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element.ThrowsClassCastException − If the type of the specified element is ... Read More

38K+ Views
A List of elements can be created using multiple ways.Way #1Create a List without specifying the type of elements it can holds. Compiler will throw warning message for it.List list = new ArrayList();Create a List and specify the type of elements it can holds.Way #2List list = new ArrayList();ExampleFollowing is the example to explain the creation of List objects −package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String[] args) { List list = new ArrayList(); list.add("Zara"); list.add("Mahnaz"); list.add("Ayan"); System.out.println("List: " ... Read More

643 Views
We can utilize Arrays.asList() method to get a List of specified elements in a single statement.Syntaxpublic static List asList(T... a)Returns a fixed-size list backed by the specified array. (Changes to the returned list "write through" to the array.)Type ParameterT − The runtime type of the array.Parametersa − The array by which the list will be backed.ReturnsA list view of the specified arrayIn case we use Arrays.asList() then we cannot add/remove elements from the list. So we use this list as an input to the ArrayList constructor to make sure that list is modifiable.ExampleThe following example shows how to create ... Read More

6K+ Views
A List of elements can be created using multiple ways.Way #1Create a List without specifying the type of elements it can holds. Compiler will throw warning message for it.List list = new ArrayList();Create a List and specify the type of elements it can holds.Way #2List list = new ArrayList();Way #3Create and initialize the list in one line.List list = Arrays.asList(object1, object 2);ExampleFollowing is the example to explain the creation of List objects −package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String[] args) { List list = new ArrayList(); list.add("Zara"); ... Read More