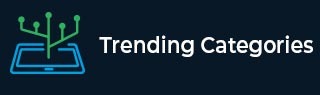
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

1K+ Views
In order to get Array Dimensions in Java, we use the getClass(), isArray() and getComponentType() methods with decision making in combination with iterative statements.The getClass() method method returns the runtime class of an object. The getClass() method is a part of the java.lang.Object class.Declaration − The java.lang.Object.getClass() method is declared as follows −public final Class getClass()The isArray() method checks whether the passed argument is an array. It returns a boolean value, either true or falseSyntax - The isArray() method has the following syntaxArray.isArray(obj)The getComponentType() method returns the Class denoting the component type of an array. If the class is not an ... Read More

460 Views
In order to get the array upperbound of a multidimensional array, we use the length() method. For a 2D array, the length() method returns the number of rows. We can access the number of columns using the array_name[0].length method.Let us see a program to get the array upperbound in Java Multidimensional arraysExample Live Demopublic class Example { public static void main(String args[]) { String[][] str = new String[5][10]; System.out.println("1st dimension : " + str.length); // displays the number of rows System.out.println("2nd dimension : " + str[0].length); // displays the number of columns } }Output1st dimension : 5 2nd dimension : 10

131 Views
In order to create and demonstrate an immutable collection in Java, we use the unmodifiableCollection() method. This method returns an unmodifiable and immutable view of the collection.Declaration − The java.util.Collections.unmodifiableCollection() method is declared as follows -public static Collection unmodifiableCollection(Collection

347 Views
In order to sort items of an ArrayList with Collections.reverseOrder() in Java, we need to use the Collections.reverseOrder() method which returns a comparator which gives the reverse of the natural ordering on a collection of objects that implement the Comparable interface.Declaration − The java.util.Collections.reverseOrder() method is declared as follows -public static Comparator reverseOrder()Let us see a program to sort an ArrayList with Collections.reverseOrder() in Java -Example Live Demoimport java.util.*; public class Example { public static void main (String[] args) { ArrayList list = new ArrayList(); list.add(10); list.add(50); ... Read More

298 Views
To rotate elements of a collection in Java, we use the Collections.rotate() method. The rotate method rotates the elements specified in the list by a specified distance. When this method is invoked, the element at index x will be the element previously at index (x - distance) mod list.size(), for all values of i between 0 and list.size()-1, inclusive.Declaration − The java.util.Collections.rotate() is declared as follows -public static void rotate(List list, int distance)Let us see a program to rotate elements of a collection in Java -Example Live Demoimport java.util.*; public class Example { public static void main(String[] args) { ... Read More

213 Views
Elements can be filled in a long array using the java.util.Arrays.fill() method. This method assigns the required long value to the long array in Java. The two parameters required are the array name and the value that is to be stored in the array elements.A program that demonstrates this is given as follows -Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { long[] longArray = new long[5]; long longValue = 125; Arrays.fill(longArray, longValue); System.out.println("The long array content is: " + Arrays.toString(longArray)); ... Read More

4K+ Views
In order to sort ArrayList in Descending order using Comparator, we need to use the Collections.reverseOrder() method which returns a comparator which gives the reverse of the natural ordering on a collection of objects that implement the Comparable interface.Declaration − The java.util.Collections.reverseOrder() method is declared as follows -public static Comparator reverseOrder()Let us see a program to sort an ArrayList in Descending order using Comparator with Java Collections −Example Live Demoimport java.util.*; public class Example { public static void main (String[] args) { ArrayList list = new ArrayList(); list.add(10); list.add(50); ... Read More

548 Views
The interfaces that are implemented by a class that is represented by an object can be determined using the java.lang.Class.getInterfaces() method. This method returns an array of all the interfaces that are implemented by the class.A program that demonstrates this is given as follows −Example Live Demopackage Test; import java.lang.*; import java.util.*; public class Demo { public static void main(String[] args) { listInterfaces(String.class); } public static void listInterfaces(Class c) { System.out.println("The Class is: " + c.getName()); Class[] interfaces = c.getInterfaces(); System.out.println("The Interfaces are: " + Arrays.asList(interfaces)); ... Read More

5K+ Views
In order to match the first name and last name using regular expression, we use the matches method in Java. The java.lang.String.matches() method returns a boolean value which depends on the matching of the String with the regular expression.Declaration −The java.lang.String.matches() method is declared as follows −public boolean matches(String regex)Let us see a program to validate the first name and last name with regular expressions −Example Live Demopublic class Example { public static void main( String[] args ) { System.out.println(firstName("Tom")); System.out.println(lastName("hanks")); } // validate first name public static boolean firstName( String ... Read More

1K+ Views
The array type can be checked using the java.lang.Class.getComponentType() method. This method returns the class that represents the component type of the array. The array length can be obtained in int form using the method java.lang.reflect.Array.getLength().A program that demonstrates this is given as follows −Example Live Demoimport java.lang.reflect.Array; public class Demo { public static void main (String args[]) { int[] arr = {6, 1, 9, 3, 7}; Class c = arr.getClass(); if (c.isArray()) { Class arrayType = c.getComponentType(); System.out.println("The array is of type: ... Read More