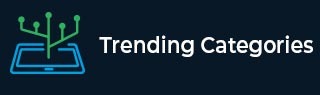
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

13K+ Views
To get the intersection of two sets, use the retainAll() method. Here are out two set −First set −HashSet set1 = new HashSet (); set1.add("Mat"); set1.add("Sat"); set1.add("Cat");Second set −HashSet set2 = new HashSet (); set2.add("Mat"); set2.add("Cat"); set2.add("Fat"); set2.add("Hat");Get the intersection −set1.retainAll(set2);The following is an example to get the intersection of two sets −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { HashSet set1 = new HashSet (); HashSet set2 = new HashSet (); set1.add("Mat"); set1.add("Sat"); set1.add("Cat"); ... Read More

2K+ Views
The location of an element in an ArrayList can be obtained using the method java.util.ArrayList.indexOf(). This method returns the index of the first occurrence of the element that is specified. If the element is not available in the ArrayList, then this method returns -1.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList = new ArrayList(); aList.add("A"); aList.add("B"); aList.add("C"); aList.add("D"); aList.add("E"); System.out.println("The ... Read More

485 Views
Use removeAll() method to get the asymmetric difference of two sets.First set −HashSet set1 = new HashSet (); set1.add("Mat"); set1.add("Sat"); set1.add("Cat");Second set −HashSet set2 = new HashSet (); set2.add("Mat");To get the asymmetric difference −set1.removeAll(set2);The following is an example that displays how to get the asymmetric difference between two sets −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { HashSet set1 = new HashSet (); HashSet set2 = new HashSet (); set1.add("Mat"); set1.add("Sat"); set1.add("Cat"); ... Read More

48 Views
Use the containsValue() method to check for the existence of a value. First, create a IdentityHashMap −Map m = new IdentityHashMap();Add some elements −m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", 150); m.put("5", 110); m.put("6", 50); m.put("7", 90); m.put("8", 250); m.put("9", 350); m.put("10", 450);Now check for the existence of a value −m.containsValue(100))The following is an example to implement containsValue() method −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { Map m = new IdentityHashMap(); m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", ... Read More

2K+ Views
The method java.util.Stack.peek() can be used to get an element from a Stack in Java without removing it. This method requires no parameters and it returns the element at the top of the stack. If the stack is empty, then the EmptyStackException is thrown.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Stack; public class Demo { public static void main (String args[]) { Stack stack = new Stack(); stack.push("Amy"); stack.push("John"); stack.push("Mary"); stack.push("Peter"); stack.push("Susan"); System.out.println("The stack ... Read More

3K+ Views
The method java.util.Stack.empty() is used to check if a stack is empty or not. This method requires no parameters. It returns true if the stack is empty and false if the stack is not empty.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Stack; public class Demo { public static void main (String args[]) { Stack stack = new Stack(); stack.push("Amy"); stack.push("John"); stack.push("Mary"); System.out.println("The stack elements are: " + stack); System.out.println("The stack is empty? " + stack.empty()); ... Read More

136 Views
The isEmpty() method is used in Java to check whether a NavigableMap is empty or not.First, create a NavigableMap and add elements to it −NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); n.put(19, "Tiger"); n.put(24, "Jacob");Now, check whether the Map is empty or not −System.out.println("Map is empty? " + n.isEmpty());The following is an example to implement isEmpty() method and check whether the Map is empty −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, ... Read More

2K+ Views
To create a Sorted Set, firstly create a Set −Set s = new HashSet();Add elements to the above set −int a[] = {77, 23, 4, 66, 99, 112, 45, 56, 39, 89}; Set s = new HashSet(); try { for(int i = 0; i < 5; i++) { s.add(a[i]); }After that, use TreeSet class to sort −TreeSet sorted = new TreeSet(s);Get the last element, using the last() method −System.out.println("Last element of the sorted set = "+ (Integer)sorted.last());The following is the code to get the last element from a Sorted Set in Java −Example Live Demoimport java.util.*; public class Demo ... Read More

2K+ Views
Fetch and remove the first element in Queue using the poll() method.Create a queue −Queue q = new LinkedList();Add some elements −q.add("abc"); q.add("def"); q.add("ghi"); q.add("jkl"); q.add("mno"); q.add("pqr"); q.add("stu"); q.add("vwx");Now, remove the first element −q.poll()The following is an example to implement the poll() method −Example Live Demoimport java.util.LinkedList; import java.util.Queue; public class Demo { public static void main(String[] args) { Queue q = new LinkedList(); q.add("abc"); q.add("def"); q.add("ghi"); q.add("jkl"); q.add("mno"); q.add("pqr"); q.add("stu"); q.add("vwx"); ... Read More

2K+ Views
To remove an element from a Queue, use the remove() method.First, set a Queue and insert some elements −Queue q = new LinkedList(); q.offer("abc"); q.offer("def"); q.offer("ghi"); q.offer("jkl"); q.offer("mno"); q.offer("pqr"); q.offer("stu"); q.offer("vwx");Remove the first element −System.out.println("Queue head = " + q.element()); System.out.println("Removing element from queue = " + q.remove());The following is an example −Example Live Demoimport java.util.LinkedList; import java.util.Queue; public class Demo { public static void main(String[] args) { Queue q = new LinkedList(); q.offer("abc"); q.offer("def"); q.offer("ghi"); q.offer("jkl"); q.offer("mno"); q.offer("pqr"); ... Read More