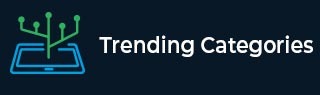
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

73 Views
The NavigableMap put() method is used to set a specific key and value in the NavigableMap.The following is an example to implement NavigableMap put() method −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); ... Read More

76 Views
To get lower key means returning the greatest key strictly less than the given key. This can be done using lowerKey() method.The following is an example to get lower key from NavigableMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); ... Read More

642 Views
Use the remove() method to remove value from HashMap.First, create a HashMap and add elements −HashMap hm = new HashMap(); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600)); hm.put("Backpack", new Integer(1200));Now, remove a value, let’s say with key “Wallet” −Object ob = hm.remove("Wallet");The following is an example to remove a value from HashMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create hash map HashMap hm = new HashMap(); hm.put("Wallet", new Integer(700)); ... Read More

294 Views
Let us first create a HashMap and add elements −HashMap hm = new HashMap(); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600));To display the content, just print the HashMap object −System.out.println("Map = "+hm);The following is an example to display content of a HashMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create hash map HashMap hm = new HashMap(); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600)); System.out.println("Map = "+hm); } }OutputMap = {Belt=600, Wallet=700}

90 Views
Use the clear() method to remove all elements from NavigableMap in Java.First, let us create NavigableMap −NavigableMap n = new TreeMap();Add elements to the NavigableMap −n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); n.put(19, "Tiger"); n.put(24, "Jacob");Remove all elements −n.clear();The following is an example to remove all elements from Java NavigableMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); ... Read More

3K+ Views
To count the number of elements in a HashSet, use the size() method.Create HashSet −String strArr[] = { "P", "Q", "R" }; Set s = new HashSet(Arrays.asList(strArr));Let us now count the number of elements in the above Set −s.size()The following is an example to count the number of elements in a HashSet −Example Live Demoimport java.util.Arrays; import java.util.HashSet; import java.util.Set; public class Demo { public static void main(String[] a) { String strArr[] = { "P", "Q", "R" }; Set s = new HashSet(Arrays.asList(strArr)); System.out.println("Elements: "+s); System.out.println("Number of Elements: ... Read More

65 Views
Check whether a Map is empty or not using the isEmpty() method.Let us first create a NavigableMap and add some elements to it −NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); n.put(19, "Tiger"); n.put(24, "Jacob");Now, use the following method to check whether the Map is empty or not. Since we added some elements above, therefore the Map is not empty −n. isEmpty();The following is an example to implement isEmpty() method −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new ... Read More

88 Views
Clear the NavigableMap in Java, using the clear() method.Let us first create a NavigableMap and add some elements to it −NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); n.put(19, "Tiger"); n.put(24, "Jacob");Now, use the clear() method −n.clear();The following is an example to implement clear() method and clear the NavigableMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); ... Read More

1K+ Views
Create an array and convert it to List −Integer[] arr = { 10, 15, 20, 10, 10, 10, 20, 30, 35, 40, 40}; List l = Arrays.asList(arr);Now, let us convert the above array to HashSet −Set s = new HashSet(l);The following is an example to convert array to HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] argv) throws Exception { Integer[] arr = { 10, 15, 20, 10, 10, 10, 20, 30, 35, 40, 40}; List l = Arrays.asList(arr); Set s = new HashSet(l); for (Iterator i = s.iterator(); i.hasNext();) { Object ele = i.next(); System.out.println(ele); } } }Output35 20 40 10 30 15

196 Views
Declare a HashSet and add elements −Set hs = new HashSet(); hs.add(20); hs.add(39); hs.add(67); hs.add(79);Now, iterate over the elements −for (Iterator i = hs.iterator(); i.hasNext();) { Object ele = i.next(); System.out.println(ele); }The following is an example that iterate over the elements of HashSet −Example Live Demoimport java.util.HashSet; import java.util.Iterator; import java.util.Set; public class Demo { public static void main(String[] argv) throws Exception { Set hs = new HashSet(); hs.add(20); hs.add(39); hs.add(67); hs.add(79); System.out.println("Elements = "); for (Iterator ... Read More