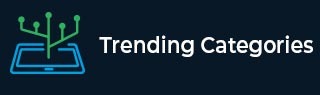
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

355 Views
To remove the highest element, use the pollLast() method.Create a TreeSet and add elements to it −TreeSet tSet = new TreeSet(); tSet.add("78"); tSet.add("56"); tSet.add("88"); tSet.add("12");Now, remove the highest element −tSet.pollLast()The following is an example to remove highest element in Java TreeSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet tSet = new TreeSet(); tSet.add("78"); tSet.add("56"); tSet.add("88"); tSet.add("12"); ... Read More

458 Views
To remove the lowest element, use the pollFirst() method.Create a TreeSet and add elements to it −TreeSet tSet = new TreeSet(); tSet.add("78"); tSet.add("56"); tSet.add("88"); tSet.add("12");Now, remove the lowest element −tSet.pollFirst()The following is an example to remove lowest element in Java TreeSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet tSet = new TreeSet(); tSet.add("78"); tSet.add("56"); tSet.add("88"); tSet.add("12"); ... Read More

509 Views
To get the last value in TreeSet, use the last() method.First, get the TreeSet and add elements to it −TreeSet tSet = new TreeSet(); tSet.add("10"); tSet.add("20"); tSet.add("30"); tSet.add("40"); tSet.add("50"); tSet.add("60");Now, get the first value −tSet.last()The following is an example to get the last value in TreeSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet tSet = new TreeSet(); tSet.add("10"); tSet.add("20"); tSet.add("30"); ... Read More

226 Views
Create a TreeSet and add elements −TreeSet tSet = new TreeSet(); tSet.add("TV"); tSet.add("Radio"); tSet.add("Internet");Now, let us copy it to object array −Object[] arr = tSet.toArray(); for (Object ob: arr) { System.out.println(ob); }The following is an example to copy all elements in TreeSet to an Object Array −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet tSet = new TreeSet(); tSet.add("TV"); tSet.add("Radio"); tSet.add("Internet"); System.out.println("TreeSet elements = "+tSet); ... Read More

95 Views
Create a TreeSet and add elements −TreeSet tSet = new TreeSet(); tSet.add("TV"); tSet.add("Radio"); tSet.add("Internet");Iterate through the elements −Iterator i = tSet.iterator(); while(i.hasNext()){ System.out.println(i.next()); }The following is an example to create a TreeSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet tSet = new TreeSet(); tSet.add("TV"); tSet.add("Radio"); tSet.add("Internet"); Iterator i = tSet.iterator(); while(i.hasNext()){ System.out.println(i.next()); } } }OutputInternet Radio TV

101 Views
Elements can be filled in a Java long array in a specified range using the java.util.Arrays.fill() method. This method assigns the required long value in the specified range to the long array in Java.The parameters required for the Arrays.fill() method are the array name, the index of the first element to be filled(inclusive), the index of the last element to be filled(exclusive) and the value that is to be stored in the array elements.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { ... Read More

267 Views
Use the clear() method to remove all the values from LinkedHashMap in Java.Create a LinkedHashMap and add some elements −LinkedHashMap l = new LinkedHashMap(); l.put("1", "Jack"); l.put("2", "Tom"); l.put("3", "Jimmy"); l.put("4", "Morgan"); l.put("5", "Tim"); l.put("6", "Brad");Now, let us remove all the values −l.clear();The following is an example to remove all values from LinkedHashMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { LinkedHashMap l = new LinkedHashMap(); l.put("1", "Jack"); l.put("2", "Tom"); ... Read More

122 Views
First, create a LinkedHashSet and add elements −LinkedHashSet l = new LinkedHashSet(); l.add(new String("1")); l.add(new String("2")); l.add(new String("3")); l.add(new String("4")); l.add(new String("5")); l.add(new String("6")); l.add(new String("7"));Now, copy it to an object array like this −// copying Object[] arr = l.toArray();The following is an example to copy all elements of a LinkedHashSet to an object array −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { LinkedHashSet l = new LinkedHashSet(); l.add(new String("1")); l.add(new String("2")); ... Read More

390 Views
Use the containsValue() method to check whether a particular value exists in LinkedHashMap or not.Create a LinkedHashMap −LinkedHashMap l = new LinkedHashMap(); l.put(1, "Mars"); l.put(2, "Earth"); l.put(3, "Jupiter"); l.put(4, "Saturn"); l.put(5, "Venus");Now, let’s say we need to check whether value “Saturn” exists or not. For that, use the containsValue() method like this −containsValue("Saturn")The following is an example to check if a particular value exists in LinkedHashMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { LinkedHashMap l = new LinkedHashMap(); l.put(1, ... Read More

210 Views
Elements can be filled in a Java double array in a specified range using the java.util.Arrays.fill() method. This method assigns the required double value in the specified range to the double array in Java.The parameters required for the Arrays.fill() method are the array name, the index of the first element to be filled(inclusive), the index of the last element to be filled(exclusive) and the value that is to be stored in the array elements.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { ... Read More