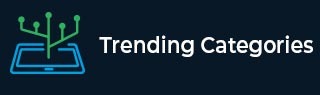
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

331 Views
Use the clear() method to remove all values from TreeMap.Let us first create a TreeMap −TreeMap m = new TreeMap();Add some elements to the TreeMap −m.put(1, "PHP"); m.put(2, "jQuery"); m.put(3, "JavaScript"); m.put(4, "Ruby"); m.put(5, "Java"); m.put(6, "AngularJS"); m.put(7, "ExpressJS");Let us now remove all the values −m.clear();The following is an example to remove all values from TreeMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { TreeMap m = new TreeMap(); m.put(1, "PHP"); m.put(2, "jQuery"); ... Read More

172 Views
To get Sub Map in Java, use the submap() method. It returns the elements in a range from the Map.First, create a TreeMap and add elements −TreeMap m = new TreeMap(); m.put(1, "PHP"); m.put(2, "jQuery"); m.put(3, "JavaScript"); m.put(4, "Ruby"); m.put(5, "Java"); m.put(6, "AngularJS"); m.put(7, "ExpressJS");Now, get the Sub Map between 4 and 6 −m.subMap(4, 6)The following is an example to get Sub Map from Tree Map in JavaExample Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeMap m = new TreeMap(); m.put(1, ... Read More

8K+ Views
An element in an ArrayList can be searched using the method java.util.ArrayList.indexOf(). This method returns the index of the first occurance of the element that is specified. If the element is not available in the ArrayList, then this method returns -1.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList = new ArrayList(); aList.add("A"); aList.add("B"); aList.add("C"); ... Read More

32K+ Views
The java.util.ArrayList.contains() method can be used to check if a Java ArrayList contains a given item or not. This method has a single parameter i.e. the item whose presence in the ArrayList is tested. Also it returns true if the item is present in the ArrayList and false if the item is not present.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList = new ArrayList(); aList.add("A"); ... Read More

572 Views
The contains() method is used to check if a particular value exists.First, create a TreeSet and add elements −TreeSet set = new TreeSet(); set.add("34"); set.add("12"); set.add("67"); set.add("54"); set.add("76"); set.add("49");Use the contains() method to check if a value exist or not i.e. 12 in this case −set.contains("12")The following is an example to check whether a particular value exist or not −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet set = new TreeSet(); set.add("34"); set.add("12"); ... Read More

150 Views
To get the Tail Set from TreeSet, use the tailSet() method. Tail Set begins from a point and returns the elements greater than the element set in the beginning.First, create a TreeSet and add elements −TreeSet set = new TreeSet(); set.add("78"); set.add("56"); set.add("88"); set.add("54"); set.add("76"); set.add("34");Now, get the Tail Set −SortedSet sorted = set.tailSet("56");The following is an example to get Tail Set from TreeSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet set = new TreeSet(); set.add("78"); set.add("56"); set.add("88"); ... Read More

698 Views
Two ArrayList can be compared to check if they are equal or not using the method java.util.ArrayList.equals(). This method has a single parameter i.e. an ArrayList that is compared with the current object. It returns true if the two ArrayList are equal and false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList1 = new ArrayList(); aList1.add("Sun"); aList1.add("Moon"); ... Read More

76 Views
Clear the IdentityHashMap using the clear() method.Create an IdentityHashMap and add elements to it −Map m = new IdentityHashMap(); m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", 150); m.put("5", 110); m.put("6", 50); m.put("7", 90); m.put("8", 250);Now, let us use the clear() method to remove all the elements −m.clear()The following is an example to remove all elements in IdentityHashMap in Java −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { Map m = new IdentityHashMap(); m.put("1", 100); ... Read More

148 Views
To get the sub set from TreeSet, use the subSet() method. Let us first create a TreeSet and add elements to it −TreeSet set = new TreeSet(); set.add("78"); set.add("56"); set.add("88"); set.add("54"); set.add("76"); set.add("34");Let us get the subset now from a range of elements −SortedSet sorted = set.subSet("54", "76");The following is an example to get sub set from Java TreeSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet set = new TreeSet(); set.add("78"); set.add("56"); ... Read More

392 Views
To get the size of TreeSet, use the size() method.Create a TreeSet and add elements to it −TreeSet tSet = new TreeSet(); tSet.add("78"); tSet.add("56"); tSet.add("88"); tSet.add("12");Now, get the size of the TreeSet −tSet.size()The following is an example to get the size of TreeSet in Java −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet tSet = new TreeSet(); tSet.add("78"); tSet.add("56"); tSet.add("88"); tSet.add("12"); ... Read More