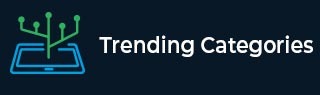
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

11K+ Views
Use the size() method to get the count of elements.Let us first create a HashMap and add elements −HashMap hm = new HashMap(); // Put elements to the map hm.put("Maths", new Integer(98)); hm.put("Science", new Integer(90)); hm.put("English", new Integer(97));Now, get the size −hm.size()The following is an example to get the count of HashMap elements −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create a hash map HashMap hm = new HashMap(); // Put elements ... Read More

6K+ Views
To add elements to HashMap, use the put() method.First, create a HashMap −HashMap hm = new HashMap();Now, let us add some elements to the HashMap −hm.put("Maths", new Integer(98)); hm.put("Science", new Integer(90)); hm.put("English", new Integer(97));The following is an example to add elements to HashMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create a hash map HashMap hm = new HashMap(); // Put elements to the map hm.put("Maths", ... Read More

13K+ Views
To retrieve the set of keys from HashMap, use the keyset() method. However, for set of values, use the values() method.Create a HashMap −HashMap hm = new HashMap(); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600)); hm.put("Backpack", new Integer(1200));Now, retrieve the keys −Set keys = hm.keySet(); Iterator i = keys.iterator(); while (i.hasNext()) { System.out.println(i.next()); }Retrieve the values −Collection getValues = hm.values(); i = getValues.iterator(); while (i.hasNext()) { System.out.println(i.next()); }The following is an example to get the set of all keys and values in HashMap −Example Live Demoimport java.util.*; public class Demo { public static ... Read More

222 Views
To create a HashMap, use the HashMap map and new −HashMap hm = new HashMap();Now, set elements −hm.put("Finance", new Double(999.87)); hm.put("Operations", new Double(298.64)); hm.put("Marketing", new Double(39.56));Display the elements now using the following code −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create a hash map HashMap hm = new HashMap(); // Put elements to the map hm.put("Finance", new Double(999.87)); hm.put("Operations", new ... Read More

54 Views
To get the size of NavigableMap, use the size() method. It returns the count of elements in the NavigableMap.Let us first create a NavigableMap and add some elements to it −NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); n.put(19, "Tiger"); n.put(24, "Jacob");Now, get the size −n.size();The following is an example to implement the size() method to get the size of the NavigableMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); ... Read More

58 Views
The NavigableMap lastEntry() method returns a key-value mapping associated with the greatest key in this map.Let us first create a NavigableMap and add some elements to it −NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); n.put(19, "Tiger"); n.put(24, "Jacob");Now, get the last entry −n.lastEntry();The following is another example to get last entry from NavigableMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put("A", 498); ... Read More

392 Views
First, use the getenv() method to get the environment variables −System.out.println("PATH = " + System.getenv("PATH"));Now, get the key and value. Loop through to get the list of environment variables −Map e = System.getenv(); for (Iterator i = e.entrySet().iterator(); i.hasNext();) { Map.Entry mapEntry = (Map.Entry) i.next(); System.out.println(mapEntry.getKey() + " = " + mapEntry.getValue()); }The following is an example to retrieve environment variables with Map Collection −Example Live Demoimport java.util.Iterator; import java.util.Map; public class Demo { public static void main(String args[]) { System.out.println("PATH = " + System.getenv("PATH")); ... Read More

111 Views
Use the subset() method to get elements from a limit. At first, create NavigableSet and add elements −NavigableSet set = new TreeSet(); set.add(10); set.add(25); set.add(40); set.add(55); set.add(70); set.add(85);Now, use the subset() method −set.subSet(40, 85)The following is an example to implement subset() method of Java NaviagbleSet class −Example Live Demoimport java.util.NavigableSet; import java.util.TreeSet; public class Demo { public static void main(String[] args) { NavigableSet set = new TreeSet(); set.add(10); set.add(25); set.add(40); set.add(55); set.add(70); set.add(85); set.add(100); System.out.println("Returned Value = " + set.subSet(40, 85)); } }OutputReturned Value = [40, 55, 70]

58 Views
The lower() method of NavigableSet returns the greatest element strictly less than the given element i.e. 35 here −lower(35);The following is an example to implement the lower() method in Java −Example Live Demoimport java.util.NavigableSet; import java.util.TreeSet; public class Demo { public static void main(String[] args) { NavigableSet set = new TreeSet(); set.add(10); set.add(25); set.add(40); set.add(55); set.add(70); set.add(85); set.add(100); System.out.println("Returned Value = " + set.lower(35)); } }OutputReturned Value = 25

1K+ Views
All the elements in a collection of objects can be traversed using the Enumeration interface. The method hasMoreElements( ) returns true if there are more elements to be enumerated and false if there are no more elements to be enumerated. The method nextElement( ) returns the next object in the enumeration.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Enumeration; import java.util.Vector; public class Demo { public static void main(String args[]) throws Exception { Vector vec = new Vector(); vec.add("John"); ... Read More