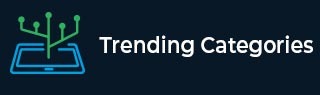
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

51K+ Views
The size of an ArrayList can be obtained by using the java.util.ArrayList.size() method as it returns the number of elements in the ArrayList i.e. the size.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList = new ArrayList(); aList.add("Apple"); aList.add("Mango"); aList.add("Guava"); aList.add("Orange"); aList.add("Peach"); ... Read More

306 Views
At first, create the first HashSet and add elements to it −// create hash set 1 HashSet hs1 = new HashSet(); hs1.add("G"); hs1.add("H"); hs1.add("I"); hs1.add("J"); hs1.add("K");Create the second HashSet and add elements to it −// create hash set 2 HashSet hs2 = new HashSet(); hs2.add("G"); hs2.add("H"); hs2.add("I"); hs2.add("J"); hs2.add("K");To check whether both the HashSet are equal or not, use the equals() method −hs1.equals(hs2)The following is an example to check whether two HashSet are equal −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // create hash set ... Read More

263 Views
First, create a HashSet and elements to it −HashSet hs = new HashSet(); // add elements to the hash set hs.add("B"); hs.add("A"); hs.add("D"); hs.add("E"); hs.add("C"); hs.add("F"); hs.add("K");Let us now convert the above HashSet to an array −Object[] ob = hs.toArray();The following is an example to convert elements in a HashSet to an array −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // create a hash set HashSet hs = new HashSet(); // add elements ... Read More

1K+ Views
To check whether a HashSet is empty or not, use the isEmpty() method.Create a HashSet −HashSet hs = new HashSet();Add elements to the HashSet −hs.add("B"); hs.add("A"); hs.add("D"); hs.add("E"); hs.add("C"); hs.add("F"); hs.add("K"); hs.add("M"); hs.add("N");Now, check whether the HashSet is empty or not. Since we added elements above, it won’t be empty −hs.isEmpty();The following is an example that checks whether a HashSet is empty or not −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // create a hash set HashSet hs = ... Read More

181 Views
Use the size() method to get the size of HashMap. Let us first create a HashMapHashMap hm = new HashMap();Now, add some elements −hm.put("Bag", new Integer(1100)); hm.put("Sunglasses", new Integer(2000)); hm.put("Franes", new Integer(800)); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600));Since, we added 5 elements above, therefore, the size() method will give 5 as the result −set.size()The following is an example to find the size of HashMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create a hash map HashMap hm = ... Read More

113 Views
Elements can be filled in a Java float array in a specified range using the java.util.Arrays.fill() method. This method assigns the required float value in the specified range to the float array in Java.The parameters required for the Arrays.fill() method are the array name, the index of the first element to be filled(inclusive), the index of the last element to be filled(exclusive) and the value that is to be stored in the array elements.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { ... Read More

379 Views
Elements can be filled in a Java byte array in a specified range using the java.util.Arrays.fill() method. This method assigns the required byte value in the specified range to the byte array in Java.The parameters required for the Arrays.fill() method are the array name, the index of the first element to be filled(inclusive), the index of the last element to be filled(exclusive) and the value that is to be stored in the array elements.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { ... Read More

427 Views
Use the isEmpty() method to check of a HashMap is empty or not. Let us first create the HashMap −HashMap hm = new HashMap();Now, add some elements −hm.put("Bag", new Integer(1100)); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600));Since, we added elements above, therefore, the HashMap isn’t empty. Let us check −set.isEmpty()The following is an example to check if a HashMap is empty or not −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create a hash map HashMap hm = new HashMap(); ... Read More

953 Views
To create a HashMap, use the HashMap class −HashMap hm = new HashMap();Add elements to the HashMap in the form of key-value pair −hm.put("Bag", new Integer(1100)); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600));The following is an example to create HashMap and add key-value pair −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create a hash map HashMap hm = new HashMap(); // Put elements to the map hm.put("Bag", new Integer(1100)); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600)); ... Read More

453 Views
Create a HashMap −HashMap hm = new HashMap();Add elements to the HashMap that we will be displaying afterward −hm.put("Maths", new Integer(98)); hm.put("Science", new Integer(90)); hm.put("English", new Integer(97)); hm.put("Physics", new Integer(91));Now, to display the HashMap elements, use Iterator. The following is an example to display HashMap elements −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create a hash map HashMap hm = new HashMap(); // Put elements to the map ... Read More