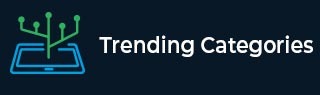
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

3K+ Views
Use the containsValue() method to check if a given value exists or not in a HashMap.First, let us create a HashMap and add some elements −HashMap hm = new HashMap(); // Put elements to the map hm.put("Bag", new Integer(1100)); hm.put("Sunglasses", new Integer(2000)); hm.put("Frames", new Integer(800)); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600));Now let us check whether a given value exist or not. Here, we are checking for the value “800” −hm.containsValue(800);The following is an example to check if a given value exists in a HashMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { ... Read More

289 Views
A range of elements can be removed from a LinkedList by using the method java.util.LinkedList.clear() along with the method java.util.LinkedList.subList(). A program that demonstrates this is given as follows −Example Live Demoimport java.util.LinkedList; public class Demo { public static void main(String[] args) { LinkedList l = new LinkedList(); l.add("Clark"); l.add("Bruce"); l.add("Diana"); l.add("Wally"); l.add("Oliver"); System.out.println("The LinkedList is: ... Read More

240 Views
First, create a HashSet −HashSet hs = new HashSet();Now, add some elements using the add() method. Set the elements as a parameter. Here, we have set string −hs.add("B"); hs.add("A"); hs.add("D"); hs.add("E"); hs.add("C"); hs.add("F"); hs.add("K"); hs.add("M");The following is an example to add elements to a HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { HashSet hs = new HashSet(); // add elements to the hash set hs.add("B"); hs.add("A"); ... Read More

62 Views
The size() method is used in IdentityHashMap to get the size of the map i.e. the count of elements.Let us first create IdentityHashMap and add some elements −Map m = new IdentityHashMap(); m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", 150); m.put("5", 110); m.put("6", 50);Now, get the size −m.size()The following is an example to get the size of the IdentityHashMap using size() method −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { Map m = new IdentityHashMap(); m.put("1", 100); ... Read More

88 Views
To get the count of NavigableMap in Java, use the size() method.Let us first create NavigableMap and add elements −NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie");Now, get the count −n.size()The following is an example to implement the size() method to get the count of the NavigableMap elements −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); ... Read More

335 Views
The elements of a Collection can be appended at the end of the ArrayList using the method java.util.ArrayList.addAll(). This method takes a single parameter i.e. the Collection whose elements are added to the ArrayList.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.Vector; public class Demo { public static void main(String[] args) { ArrayList aList = new ArrayList(); aList.add("John"); aList.add("Sally"); aList.add("Harry"); aList.add("Martha"); ... Read More

4K+ Views
Elements can be added in the middle of an ArrayList by using the java.util.ArrayList.add() method. This method has two parameters i.e. the index at which to insert the element in the ArrayList and the element itself. If there is an element already present at the index specified by ArrayList.add() then that element and all subsequent elements shift to the right by one.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String args[]) throws Exception { List aList = new ... Read More

8K+ Views
Use the containsKey() method and check if a given key exists in the HashMap or not.Let us first create HashMap and add some elements −// Create a hash map HashMap hm = new HashMap(); // Put elements to the map hm.put("Bag", new Integer(1100)); hm.put("Sunglasses", new Integer(2000)); hm.put("Frames", new Integer(800)); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600));Now, let’s say we need to check whether the key “Bag” exists or not, For that, use the containsKey() method like this −hm.containsKey("Bag")The following is an example to check if a given key exists in HashMap −Example Live Demoimport java.util.*; public class Demo { ... Read More

3K+ Views
To get the maximum element of HashSet, use the Collections.max() method.Declare the HashSet −Set hs = new HashSet();Now let us add the elements −hs.add(29); hs.add(879); hs.add(88); hs.add(788); hs.add(456);Let us now get the maximum element −Object obj = Collections.max(hs);The following is an example to find maximum element of HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // create hash set Set hs = new HashSet(); hs.add(29); hs.add(879); ... Read More

359 Views
An ArrayList can be cloned using the java.util.ArrayList.clone() method. This method does not take any parameters but returns a shallow copy the specified ArrayList instance. This means that the new ArrayList created using the ArrayList.clone() method refers to the same elements as the original ArrayList but it does not duplicate the elements.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList1 = new ArrayList(); aList1.add("Apple"); ... Read More