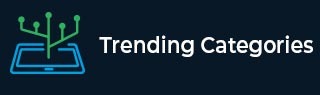
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

68 Views
The lowerEntry() method in NavigabelMap returns a key-value mapping associated with the greatest key strictly less than the given key.The following is an example to implement lowerEntry() methodExample Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); n.put(19, "Tiger"); n.put(24, "Jacob"); System.out.println("NavigableMap elements..."+n); System.out.println("Lower Entry is ... Read More

84 Views
The higherEntry() method in NavigableMap returns a key-value mapping associated with the least key strictly greater than the given key.The following is an example to implement higherEntry() method −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, "Kurt"); n.put(19, "Tiger"); n.put(24, "Jacob"); System.out.println("NavigableMap elements..."+n); System.out.println("Higher Entry ... Read More

2K+ Views
Use the put() method to add elements to LinkedHashMap collection.First, let us create a LinkedHashMap −LinkedHashMap l = new LinkedHashMap();Now, add elements −l.put("1", "Jack"); l.put("2", "Tom"); l.put("3", "Jimmy"); l.put("4", "Morgan"); l.put("5", "Tim"); l.put("6", "Brad");The following is an example to add elements to LinkedHashMap collection −Example Live Demoimport java.util.LinkedHashMap; public class Demo { public static void main(String[] args) { LinkedHashMap l = new LinkedHashMap(); l.put("1", "Jack"); l.put("2", "Tom"); l.put("3", "Jimmy"); ... Read More

174 Views
Firstly, create a HashMap −HashMap hm = new HashMap();Add some elements to the HashMap −hm.put("Shirts", new Integer(700)); hm.put("Trousers", new Integer(600)); hm.put("Jeans", new Integer(1200)); hm.put("Android TV", new Integer(450)); hm.put("Air Purifiers", new Integer(300)); hm.put("Food Processors", new Integer(950));Now, sort the HashMap based on keys using TreeMap −Map sort = new TreeMap(hm); System.out.println("Sorted Map based on key = "+sort);The following is an example to sort HasMap based on keys −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { HashMap hm = new HashMap(); hm.put("Shirts", new ... Read More

732 Views
Use the contains() method to check if a particular element exists −Set hs = new HashSet(); hs.add(30); hs.add(67); hs.add(88); hs.add(33); hs.add(54); hs.add(90); hs.add(66); hs.add(79);To check for let’s say, element 89, use the contains() method −hs.contains(89));The following is an example to check if a particular element exists in HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // create hash set Set hs = new HashSet(); hs.add(30); hs.add(67); ... Read More

260 Views
The synchronizedSet() method returns a synchronized (thread-safe) sorted set backed by the specified sorted set.First, create a HashSet and add elements −Set hs = new HashSet(); hs.add(29); hs.add(879); hs.add(88); hs.add(788); hs.add(456);Now, to get synchronized set, use the synchronizedSet() method −Set s = Collections.synchronizedSet(hs);The following is an example to get synchronized set from HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { Set hs = new HashSet(); hs.add(29); hs.add(879); ... Read More

190 Views
To get enumeration over HashSet, first declare the HashSet and add elements −HashSet hs = new HashSet(); hs.add("P"); hs.add("Q"); hs.add("R");To get enumeration −Enumeration e = Collections.enumeration(hs);The following is an example to get Enumeration over HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { HashSet hs = new HashSet(); hs.add("P"); hs.add("Q"); hs.add("R"); Enumeration e = Collections.enumeration(hs); while (e.hasMoreElements()) System.out.println(e.nextElement()); } }OutputP Q R

2K+ Views
To get the minimum element of HashSet, use the Collections.min() method.Declare the HashSet −Set hs = new HashSet();Now let us add the elements −hs.add(29); hs.add(879); hs.add(88); hs.add(788); hs.add(456);Let us now get the minimum element −Object obj = Collections.min(hs);The following is an example to find the minimum element of HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // create hash set Set hs = new HashSet(); hs.add(29); hs.add(879); ... Read More

2K+ Views
To check whether an element is in a HashSet or not in Java, use the contains() method.Create a HashSet −HashSet hs = new HashSet();Add elements to it −hs.add("R"); hs.add("S"); hs.add("T"); hs.add("V"); hs.add("W"); hs.add("Y"); hs.add("Z");To check for an element, for example, S here, use the contains() −hs.contains("S")The following is an example to check for an element in a HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { HashSet hs = new HashSet(); // add elements to the HashSet ... Read More

982 Views
To remove a single element from a HashSet, use the remove() method.First, create a HashSet −HashSet hs = new HashSet();Now, add elements to the HashSet −hs.add("R"); hs.add("S"); hs.add("T"); hs.add("V"); hs.add("W"); hs.add("Y"); hs.add("Z");Let us now remove an element −hs.remove("R");The following is an example to remove a single element from a HashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { HashSet hs = new HashSet(); // add elements to the hash set hs.add("R"); ... Read More