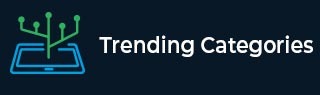
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
Use the firstEntry() method in TreeMap to retrieve the first entry.Create a TreeMap and add some elementsTreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); m.put(5, "Canada");Now, retrieve the first entrym.firstEntry()The following is an example to retrieve first entry in the TreeMapExample Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); m.put(5, "Canada"); ... Read More

65 Views
To get floor key means to return the greatest key less than or equal to the given key, or null if there is no such key. This can be done with the floorKey() metho.The following is an example to get floor key from NavigableMapExample Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put("A", 498); n.put("B", 389); n.put("C", 868); ... Read More

65 Views
The navigableKeySet() method is used to get view of the Map from NavigableMap.Let us first create a NavigableMapNavigableMap n = new TreeMap();Add some elements in the NavigableMapn.put("A", 498); n.put("B", 389); n.put("C", 868); n.put("D", 988); n.put("E", 686); n.put("F", 888); n.put("G", 999); n.put("H", 444); n.put("I", 555); n.put("J", 666);Get Navigable Key Set nown.navigableKeySet()The following is an example to get navigable key setExample Live Demoimport java.util.ArrayList; import java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put("A", ... Read More

26K+ Views
An element can be retrieved from the ArrayList in Java by using the java.util.ArrayList.get() method. This method has a single parameter i.e. the index of the element that is returned.A program that demonstrates this is given as followsExample Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String args[]) throws Exception { List aList = new ArrayList(); aList.add("James"); aList.add("George"); aList.add("Bruce"); aList.add("Susan"); ... Read More

185 Views
Elements can be filled in a Java char array in a specified range using the java.util.Arrays.fill() method. This method assigns the required char value in the specified range to the char array in Java.The parameters required for the Arrays.fill() method are the array name, the index of the first element to be filled(inclusive), the index of the last element to be filled(exclusive) and the value that is to be stored in the array elements.A program that demonstrates this is given as followsExample Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { ... Read More

66 Views
Returning a shallow copy means to copy elements of one IdentityHashMap to another. For this, clone() method is used.The following is an example to return a shallow copy of IdentityHashMapExample Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { IdentityHashMap m = newIdentityHashMap(); m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", 150); m.put("5", 110); m.put("6", 50); m.put("7", 90); m.put("8", 250); m.put("9", 350); m.put("10", 450); System.out.println("IdentityHashMap elements"+ m); System.out.println("Size = " + m.size()); System.out.println("Cloned Map = " + m.clone()); 0System.out.println("Size = " + m.size()); } }OutputIdentityHashMap elements {2=200, 4=150, 9=350, 7=90, 10=450, 6=50, 5=110, 8=250, 1=100, 3=300} Size = 10 Cloned Map = {2=200, 4=150, 9=350, 7=90, 10=450, 6=50, 5=110, 8=250, 1=100, 3=300} Size = 10

92 Views
Use the containsValue() method to check whether a particular value exists in IdentityHashMap or not.Create a IdentityHashMapMap m = newIdentityHashMap(); m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", 150); m.put("5", 110); m.put("6", 50); m.put("7", 90); m.put("8", 250); m.put("9", 350); m.put("10", 450);Now, let’s say we need to check whether value 100 exists or not. For that, use the containsValue() method like thism.containsValue(100)The following is an example to check for the existence of a value in IdentityHashMapExample Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { Map m = newIdentityHashMap(); ... Read More

79 Views
Use the containsKey() method to check whether a particular key exists in IdentityHashMap or not.Create a IdentityHashMapMap m = newIdentityHashMap(); m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", 150); m.put("5", 110); m.put("6", 50); m.put("7", 90); m.put("8", 250); m.put("9", 350); m.put("10", 450);Now, let’s say we need to check whether key 7 exists or not. For that, use the containsKey() method like thism.containsKey(“7”)The following is an example to check for the existence of a key in IdentityHashMapExample Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { Map m = newIdentityHashMap(); m.put("1", 100); ... Read More

80 Views
Use the clear() method to remove all the elements from IdentityHashMap in Java.Create a IdentityHashMap and add some elementsMap m = newIdentityHashMap(); m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", 150); m.put("5", 110); m.put("6", 50); m.put("7", 90); m.put("8", 250); m.put("9", 350); m.put("10", 450);Now, let us remove all the elementm.clear();The following is an example to remove all elements in IdentityHashMap in JavaExample Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { Map m = newIdentityHashMap(); m.put("1", 100); m.put("2", 200); m.put("3", 300); m.put("4", 150); ... Read More

1K+ Views
To get the first value in TreeSet, use the first() method.First, get the TreeSet and add elements to itTreeSet tSet = new TreeSet(); tSet.add("10"); tSet.add("20"); tSet.add("30"); tSet.add("40"); tSet.add("50"); tSet.add("60");Now, get the first valuetSet.first()The following is an example to get the first value in TreeSetExample Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet tSet = new TreeSet(); tSet.add("10"); tSet.add("20"); tSet.add("30"); tSet.add("40"); tSet.add("50"); tSet.add("60"); System.out.println("TreeSet elements..."); Iterator i = tSet.iterator(); ... Read More