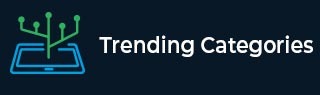
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

102 Views
The ceiling() method returns the least element greater than or equal to the given element i.e. 30 hereceiling(30)The following is an example to implement the ceiling method in JavaExample Live Demoimport java.util.NavigableSet; import java.util.TreeSet; public class Demo { public static void main(String[] args) { NavigableSet set = new TreeSet(); set.add(10); set.add(25); set.add(40); set.add(55); set.add(70); set.add(85); set.add(100); System.out.println("Returned Value = " + set.ceiling(30)); } }OutputReturned Value = 40

97 Views
Use Iterator class to fetch elements of TreeSet.Create a TreeSet and add elements to itTreeSet set = new TreeSet(); set.add("13"); set.add("11"); set.add("12"); set.add("16"); set.add("19"); set.add("23"); set.add("21"); set.add("20"); set.add("30");Now, to display the elements, use Iterator classIterator i = set.iterator(); while(i.hasNext()){ System.out.println(i.next()); }The following is an example that fetch elements of TreeSet using IterationExample Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet set = new TreeSet(); set.add("13"); set.add("11"); ... Read More

142 Views
To get the element ordered last i.e. the last element in TreeSet, use the last() method.Create a TreeSet and add elements to itTreeSet set = new TreeSet (); set.add("65"); set.add("45"); set.add("19"); set.add("27"); set.add("89"); set.add("57");Now, get the last elementset.last()The following is an example to get the element ordered last in TreeStExample Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeSet set = new TreeSet (); set.add("65"); set.add("45"); set.add("19"); ... Read More

677 Views
The elements of the ArrayList can be accessed one by one by using a for loop. A program that demonstrates this is given as followsExample Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { ArrayList aList = new ArrayList(); aList.add("Sun"); aList.add("Moon"); aList.add("Star"); aList.add("Planet"); aList.add("Comet"); System.out.println("The ArrayList elements are:"); for (String s : aList) { System.out.println(s); } } }OutputThe output of the above ... Read More

6K+ Views
The iterator can be used to iterate through the ArrayList wherein the iterator is the implementation of the Iterator interface. Some of the important methods declared by the Iterator interface are hasNext() and next().The hasNext() method returns true if there are more elements in the ArrayList and otherwise returns false. The next() method returns the next element in the ArrayList.A program that demonstrates iteration through ArrayList using the Iterator interface is given as followsExample Live Demoimport java.util.ArrayList; import java.util.Iterator; import java.util.List; public class Demo { public static void main(String[] args) { List aList = new ArrayList(); ... Read More

88 Views
Let us first create a NavigableMapNavigableMap n = new TreeMap();Now, let us add some elementsn.put("A", 888); n.put("B", 999); n.put("C", 444); n.put("D", 555); n.put("E", 666); n.put("F", 888);The following is the complete example to create NavigableMap, add elements and display themExample Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put("A", 888); n.put("B", 999); n.put("C", 444); ... Read More

579 Views
To remove the last entry of the TreeMap, use the pollLastEntry() method.Let us first create a TreeMap and add elementsTreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); m.put(5, "Canada");Remove the last entry nowm.pollLastEntry()The following is an example to remove the last entry of the TreeMapExample Live Demoimport java.util.*; public class Demo { public static void main(String args[]){ TreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); m.put(5, "Canada"); System.out.println("TreeMap Elements ... Read More

31K+ Views
The java.util.ArrayList.contains() method can be used to check if an element exists in an ArrayList or not. This method has a single parameter i.e. the element whose presence in the ArrayList is tested. Also it returns true if the element is present in the ArrayList and false if the element is not present.A program that demonstrates this is given as followsExample Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList = new ArrayList(); aList.add("A"); aList.add("B"); aList.add("C"); aList.add("D"); ... Read More

107 Views
The method java.util.ArrayList.removeAll() removes all the the elements from the ArrayList that are available in another collection. This method has a single parameter i.e. the Collection whose elements are to be removed from the ArrayList.A program that demonstrates this is given as followsExample Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String args[]) throws Exception { List aList1 = new ArrayList(); aList1.add("Anna"); aList1.add("John"); aList1.add("Mary"); ... Read More

653 Views
The sub-list of an ArrayList can be obtained using the java.util.ArrayList.subList() method. This method takes two parameters i.e. the start index for the sub-list(inclusive) and the end index for the sub-list(exclusive) from the required ArrayList. If the start index and the end index are the same, then an empty sub-list is returned.A program that demonstrates this is given as followsExample Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { ArrayList aList = new ArrayList(); aList.add("Apple"); ... Read More