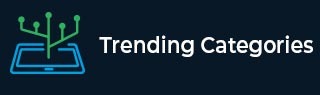
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

4K+ Views
A specific element in the LinkedList can be removed using the java.util.LinkedList.remove() method. This method removes the specified element the first time it occurs in the LinkedList and if the element is not in the LinkedList then no change occurs. The parameter required for the LinkedList.remove() method is the element to be removed.A program that demonstrates this is given as follows.Example Live Demoimport java.util.LinkedList; public class Demo { public static void main(String[] args) { LinkedList l = new LinkedList(); l.add("Apple"); l.add("Mango"); l.add("Pear"); l.add("Orange"); ... Read More

15K+ Views
A Set is a generic set of values with no duplicate elements. A TreeSet is a set where the elements are sorted.A HashSet is a set where the elements are not sorted or ordered. It is faster than a TreeSet. The HashSet is an implementation of a Set.Set is a parent interface of all set classes like TreeSet, HashSet, etc.Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { int a[] = {77, 23, 4, 66, 99, 112, 45, 56, 39, 89}; Set s = new HashSet(); ... Read More

220 Views
First, create a HashMap and add elementsHashMap hm = new HashMap(); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600)); hm.put("Backpack", new Integer(1200));Now, retrieve all the keysSet keys = hm.keySet(); System.out.println("Keys..."); Iterator i = keys.iterator(); while (i.hasNext()) { System.out.println(i.next()); }The following is an example to get the set of all key in HashMapExample Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create hash map HashMap hm = new HashMap(); hm.put("Wallet", new Integer(700)); ... Read More

4K+ Views
To remove all values from HashMap, use the clear() method.First, let us create a HashMap.HashMap hm = new HashMap();Add some elements to the HashMaphm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600)); hm.put("Backpack", new Integer(1200));Now, remove all the elementshm.clear();The following is an example to remove all values from HashMap.Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { // Create hash map HashMap hm = new HashMap(); hm.put("Wallet", new Integer(700)); hm.put("Belt", new Integer(600)); ... Read More

1K+ Views
An LinkedList can be cleared in Java using the method java.util.LinkedList.clear(). This method removes all the elements in the LinkedList. There are no parameters required by the LinkedList.clear() method and it returns no value.A program that demonstrates this is given as follows.Example Live Demoimport java.util.LinkedList; public class Demo { public static void main(String[] args) { LinkedList l = new LinkedList(); l.add("Orange"); l.add("Apple"); l.add("Peach"); l.add("Guava"); ... Read More

663 Views
The last index of a particular element in an ArrayList can be obtained by using the method java.util.ArrayList.lastIndexOf(). This method returns the index of the last occurance of the element that is specified. If the element is not available in the ArrayList, then this method returns -1.A program that demonstrates this is given as follows.Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList = new ArrayList(); aList.add("Orange"); aList.add("Apple"); aList.add("Peach"); aList.add("Orange"); aList.add("Mango"); ... Read More

26K+ Views
The index of a particular element in an ArrayList can be obtained by using the method java.util.ArrayList.indexOf(). This method returns the index of the first occurrence of the element that is specified. If the element is not available in the ArrayList, then this method returns -1.A program that demonstrates this is given as follows.Example Live Demoimport java.util.ArrayList; import java.util.List; public class Demo { public static void main(String[] args) { List aList = new ArrayList(); aList.add("Orange"); aList.add("Apple"); aList.add("Peach"); aList.add("Guava"); aList.add("Mango"); ... Read More

57 Views
The pollFirstEntry() method in NavigableMap remove and return a key-value mapping associated with the least key in this mapThe following is an example to implement pollFirstEntry() methodExample Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put(5, "Tom"); n.put(9, "John"); n.put(14, "Jamie"); n.put(1, "Tim"); n.put(4, "Jackie"); n.put(15, ... Read More

387 Views
The unmodifiable view of the specified ArrayList can be obtained by using the method java.util.Collections.unmodifiableList(). This method has a single parameter i.e. the ArrayList and it returns the unmodifiable view of that ArrayList.A program that demonstrates this is given as followsExample Live Demoimport java.util.ArrayList; import java.util.ArrayList; import java.util.Collections; import java.util.List; public class Demo { public static void main(String args[]) throws Exception { List aList = new ArrayList(); aList.add("Sally"); aList.add("George"); aList.add("John"); aList.add("Susan"); aList.add("Martha"); aList = Collections.unmodifiableList(aList); System.out.println("The ... Read More

264 Views
The elements of a Collection can be inserted at the specified index of the ArrayList using the method java.util.ArrayList.addAll(). This method has two parameters i.e. the specific index at which to start inserting the Collection elements in the ArrayList and the Collection itself.If there is an element already present at the index specified by ArrayList.addAll() then that element and all subsequent elements are shifted to the right to make the space for the Collection elements in the ArrayList.A program that demonstrates this is given as follow.Example Live Demoimport java.util.ArrayList; import java.util.Vector; public class Demo { public static void main(String[] args) ... Read More