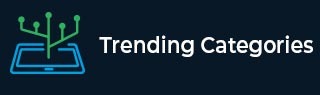
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

6K+ Views
An Iterator can be used to loop through an LinkedList. The method hasNext( ) returns true if there are more elements in LinkedList and false otherwise. The method next( ) returns the next element in the LinkedList and throws the exception NoSuchElementException if there is no next element.A program that demonstrates this is given as follows.Example Live Demoimport java.util.LinkedList; import java.util.Iterator; public class Demo { public static void main(String[] args) { LinkedList l = new LinkedList(); l.add("John"); l.add("Sara"); l.add("Susan"); l.add("Betty"); l.add("Nathan"); ... Read More

17K+ Views
An element can be removed from a Collection using the Iterator method remove(). This method removes the current element in the Collection. If the remove() method is not preceded by the next() method, then the exception IllegalStateException is thrown.A program that demonstrates this is given as follows.Example Live Demoimport java.util.ArrayList; import java.util.Iterator; public class Demo { public static void main(String[] args) { ArrayList aList = new ArrayList(); aList.add("Apple"); aList.add("Mango"); aList.add("Guava"); aList.add("Orange"); aList.add("Peach"); System.out.println("The ArrayList elements are: "); ... Read More

170 Views
An Iterator can be used to loop through a HashMap. The method hasNext( ) returns true if there are more elements in HashMap and false otherwise. The method next( ) returns the next key element in the HashMap and throws the exception NoSuchElementException if there is no next element.A program that demonstrates this is given as follows.Example Live Demoimport java.util.HashMap; import java.util.Iterator; import java.util.Map; public class Demo { public static void main(String[] args) { Map student = new HashMap(); student.put("101", "Harry"); student.put("102", "Amy"); student.put("103", "John"); ... Read More

4K+ Views
An Iterator can be used to loop through an ArrayList. The method hasNext( ) returns true if there are more elements in ArrayList and false otherwise. The method next( ) returns the next element in the ArrayList and throws the exception NoSuchElementException if there is no next element.A program that demonstrates this is given as follows.Example Live Demoimport java.util.ArrayList; import java.util.Iterator; public class Demo { public static void main(String[] args) { ArrayList aList = new ArrayList(); aList.add("Apple"); aList.add("Mango"); aList.add("Guava"); aList.add("Orange"); aList.add("Peach"); ... Read More

336 Views
An object array can be created from the elements of a LinkedList using the method java.util.LinkedList.toArray(). This method returns the object array with all the LinkedList elements in the correct order.A program that demonstrates this is given as follows.Example Live Demoimport java.util.LinkedList; public class Demo { public static void main(String[] args) { LinkedList l = new LinkedList(); l.add("Amy"); l.add("Sara"); l.add("Joe"); l.add("Betty"); l.add("Nathan"); Object[] objArr = l.toArray(); System.out.println("The object array elements are: "); for ... Read More

176 Views
A ListIterator can be used to traverse the elements in the forward direction as well as the reverse direction in a LinkedList.The method hasNext( ) in ListIterator returns true if there are more elements in the LinkedList while traversing in the forward direction and false otherwise. The method next( ) returns the next element in the LinkedList and advances the cursor position.The method hasPrevious( ) in ListIterator returns true if there are more elements in the LinkedList while traversing in the reverse direction and false otherwise. The method previous( ) returns the previous element in the LinkedList and reduces the ... Read More

977 Views
An element in ArrayList can be replaced using the ListIterator method set(). This method has a single parameter i.e. the element that is to be replaced and the set() method replaces it with the last element returned by the next() or previous() methods.A program that demonstrates this is given as follows.Example Live Demoimport java.util.ArrayList; import java.util.ListIterator; public class Demo { public static void main(String[] args) { ArrayList aList = new ArrayList(); aList.add("Amanda"); aList.add("Taylor"); aList.add("Justin"); aList.add("Emma"); aList.add("Peter"); System.out.println("The ArrayList elements ... Read More

767 Views
An element can be removed from an ArrayList using the ListIterator method remove(). This method removes the current element in the ArrayList. If the remove() method is not preceded by the next() method, then the exception IllegalStateException is thrown.A program that demonstrates this is given as follows.Example Live Demoimport java.util.ArrayList; import java.util.ListIterator; public class Demo { public static void main(String[] args) { ArrayList aList = new ArrayList(); aList.add("Apple"); aList.add("Mango"); aList.add("Guava"); aList.add("Orange"); aList.add("Peach"); System.out.println("The ArrayList elements are: "); ... Read More

878 Views
The previous index and next index in an ArrayList can be obtained using the methods previousIndex() and nextIndex() respectively in the ListIterator Interface.The previousIndex() method returns the index of the element that is returned by the previous() method while the nextIndex() method returns the index of the element that is returned by the next() method. Neither of these methods require any parameters.A program that demonstrates this is given as followsExample Live Demoimport java.util.ArrayList; import java.util.ListIterator; public class Demo { public static void main(String[] args) { ArrayList aList = new ArrayList(); aList.add("Amy"); ... Read More

737 Views
A ListIterator can be used to traverse the elements in the forward direction as well as the reverse direction in a LinkedList. The method hasPrevious( ) in ListIterator returns true if there are more elements in the LinkedList while traversing in the reverse direction and false otherwise. The method previous( ) returns the previous element in the LinkedList and reduces the cursor position backward.A program that demonstrates this is given as follows.Example Live Demoimport java.util.LinkedList; import java.util.List; import java.util.ListIterator; public class Demo { public static void main(String[] args) { List l = new LinkedList(); ... Read More