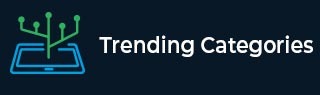
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

124 Views
To display the first key from NavigableMap in Java, use the firstKey() method.Let us first create NavigableMap −NavigableMap n = new TreeMap(); n.put("A", 498); n.put("B", 389); n.put("C", 868); n.put("D", 988); n.put("E", 686); n.put("F", 888); n.put("G", 999); n.put("H", 444); n.put("I", 555); n.put("J", 666);Get the first key now −n.firstKey()The following is an example to get the first key from NavigableMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { NavigableMap n = new TreeMap(); n.put("A", 498); n.put("B", ... Read More

873 Views
To remove the first entry of the TreeMap, use the pollFirstEntry() method.Let us first create a TreeMap and add elements −TreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); m.put(5, "Canada");Remove the first entry now −m.pollFirstEntry()The following is an example to remove the first entry of the TreeMap.Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { TreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); ... Read More

93 Views
Use the remove() method to remove a key from a TreeMap only if it is associated with a given value. Let us first create a TreeMap and add some elements −TreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); m.put(5, "Canada");To remove a key, set the key and the associated value here. If the associate value exists, then the key will get removed −m.remove(3, "Australia")The following is an example to remove a key from a TreeMap only if it is associated with a given value −Example Live Demoimport java.util.*; public class Demo { public static void ... Read More

437 Views
Use the remove() method to remove a key from TreeMap.Let us first create a TreeMap and add some elements −TreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); m.put(5, "Canada");Let us remove a key now. Here, we are removing key 3 now −m.remove(3)The following is an example to remove a key from TreeMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { TreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); ... Read More

1K+ Views
A TreeMap cannot contain duplicate keys. TreeMap cannot contain the null key. However, It can have null values.Let us first see how to create a TreeMap −TreeMap m = new TreeMap();Add some elements in the form of key-value pairs −m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); m.put(4, "Netherlands"); m.put(5, "Canada");The following is an example to create a TreeMap and add key-value pairs −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { TreeMap m = new TreeMap(); m.put(1, "India"); m.put(2, "US"); m.put(3, "Australia"); ... Read More

629 Views
Use the remove() method to remove a value from TreeMap.Create a TreeMap first and add some elements −TreeMap m = new TreeMap(); m.put(1, "PHP"); m.put(2, "jQuery"); m.put(3, "JavaScript"); m.put(4, "Ruby"); m.put(5, "Java"); m.put(6, "AngularJS"); m.put(7, "ExpressJS");Now, let’s say you need to remove a value with key 5. For that, use the remove() method −Object ob = m.remove(5);The following is an example to remove a value from TreeMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { TreeMap m = new TreeMap(); m.put(1, "PHP"); m.put(2, "jQuery"); ... Read More

130 Views
Get the TreeMap size using the size() method.First, create a TreeMap and add elements to it −TreeMap m = new TreeMap(); m.put(1, "PHP"); m.put(2, "jQuery"); m.put(3, "JavaScript"); m.put(4, "Ruby"); m.put(5, "Java"); m.put(6, "AngularJS"); m.put(7, "ExpressJS");Count the number of elements in the above TreeMap or get the size using the size() method as shown below −m.size()To get the size of TreeMap, the following is the code −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { TreeMap m = new TreeMap(); m.put(1, "PHP"); m.put(2, "jQuery"); ... Read More

1K+ Views
Use the lastKey() method to get the highest key stored in TreeMap.Let us first set the TreeMap and add some elements to it −TreeMap m = new TreeMap(); m.put(1, "PHP"); m.put(2, "jQuery"); m.put(3, "JavaScript"); m.put(4, "Ruby"); m.put(5, "Java"); m.put(6, "AngularJS"); m.put(7, "ExpressJS");Now get the highest key −m.lastKey()The following is an example to get the highest key in TreeMap −Example Live Demoimport java.util.*; public class Demo { public static void main(String args[]) { TreeMap m = new TreeMap(); m.put(1, "PHP"); m.put(2, "jQuery"); m.put(3, "JavaScript"); m.put(4, "Ruby"); ... Read More

2K+ Views
The last element of a Linked List can be retrieved using the method java.util.LinkedList.getLast() respectively. This method does not require any parameters and it returns the last element of the LinkedList.A program that demonstrates this is given as follows −Example Live Demoimport java.util.LinkedList; public class Demo { public static void main(String[] args) { LinkedList l = new LinkedList(); l.add("Andy"); l.add("Sara"); l.add("James"); l.add("Betty"); l.add("Bruce"); System.out.println("The last element of the Linked List is : " + l.getLast()); } }OutputThe last ... Read More

313 Views
The first element can be deleted from a LinkedList by using the method java.util.LinkedList.removeFirst(). This method does not have any parameters and it returns the first element of the LinkedList.The last element can be deleted from a LinkedList by using the method java.util.LinkedList.removeLast(). This method does not have any parameters and it returns the last element of the LinkedList.A program that demonstrates this is given as follows −Example Live Demoimport java.util.LinkedList; public class Demo { public static void main(String[] args) { LinkedList l = new LinkedList(); l.add("Apple"); l.add("Mango"); ... Read More