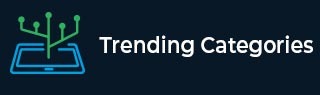
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

102 Views
The method java.time.Matcher.pattern() returns the pattern that is matched upon by the Matcher. This method accepts no parameters.A program that demonstrates the method Matcher.pattern() in Java regular expressions is given as follows −Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { String regex = "Apple"; Pattern p = Pattern.compile(regex); Matcher m = p.matcher("AppleIsAFruit"); System.out.println("Pattern: " + m.pattern()); } }The output of the above program is as follows −Pattern: AppleNow let us understand the above program.The pattern that is matched upon ... Read More

10K+ Views
A class contains a constructor to initialize instance variables in Java. This constructor is called when the class object is created.A program that demonstrates this is given as follows −Example Live Democlass Student { private int rno; private String name; public Student(int r, String n) { rno = r; name = n; } public void display() { System.out.println("Roll Number: " + rno); System.out.println("Name: " + name); } } public class Demo { public static void main(String[] args) { Student ... Read More

125 Views
The elements of a Collection can be appended at the end of the Vector using the method java.util.Vector.addAll(). This method takes a single parameter i.e. the Collection whose elements are added to the Vector and it returns true if the Vector is changed.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Vector; public class Demo { public static void main(String args[]) { Vector vec1 = new Vector(); vec1.add(7); vec1.add(3); vec1.add(5); vec1.add(9); vec1.add(2); System.out.println("The Vector vec1 elements ... Read More

391 Views
Elements can be added in the middle of a Vector by using the java.util.Vector.insertElementAt() method. This method has two parameters i.e. the element that is to be inserted in the Vector and the index at which it is to be inserted. If there is an element already present at the index specified by Vector.insertElementAt() then that element and all subsequent elements shift to the right by one.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Vector; public class Demo { public static void main(String args[]) { Vector vec = new Vector(5); ... Read More

1K+ Views
A dynamic array is implemented by a Vector. This means an array that can grow or reduce in size as required.Elements can be added at the end of the Vector using the java.util.Vector.add() method. This method has a single parameter i.e. the element to be added. It returns true if the element is added to the Vector as required and false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Vector; public class Demo { public static void main(String args[]) { Vector vec = new Vector(5); vec.add(4); vec.add(1); ... Read More

251 Views
To get the size of LinkedHashSet, use the size() method.First, set the LinkedHashSet and add elements −LinkedHashSet set = new LinkedHashSet(); set.add(10); set.add(20); set.add(30); set.add(40); set.add(50); set.add(60);Display the size of the LinkedHashSet created above −set.size()The following is an example to get the size of LinkedHashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { LinkedHashSet set = new LinkedHashSet(); set.add(10); set.add(20); set.add(30); set.add(40); set.add(50); set.add(60); System.out.println("LinkedHashSet elements..."); System.out.println(set); ... Read More

252 Views
First, create a LinkedHashSet and add elements to it −LinkedHashSet set = new LinkedHashSet(); set.add(10); set.add(20); set.add(30); set.add(40); set.add(50); set.add(60);Now, use the Iterator to iterate through the elements −Iterator i = set.iterator(); while (i.hasNext()) { System.out.println(i.next()); }The following is an example to iterate through elements of LinkedHashSet −Example Live Demoimport java.util.*; public class Demo { public static void main(String[] args) { LinkedHashSet set = new LinkedHashSet(); set.add(10); set.add(20); set.add(30); set.add(40); set.add(50); set.add(60); System.out.println("LinkedHashSet..."); ... Read More

273 Views
An ArrayList can be created from another collection using the java.util.Arrays.asList() method. A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.Arrays; import java.util.List; public class Demo { public static void main(String args[]) throws Exception { String str[] = { "John", "Macy", "Peter", "Susan", "Lucy" }; List aList = new ArrayList(Arrays.asList(str)); System.out.println("The ArrayList elements are: " + aList); } }OutputThe ArrayList elements are: [John, Macy, Peter, Susan, Lucy]Now let us understand the above program.The string array str[] is defined. Then an ArrayList is created using ... Read More

549 Views
Elements can be filled in a Java int array in a specified range using the java.util.Arrays.fill() method. This method assigns the required int value in the specified range to the int array in Java.The parameters required for the Arrays.fill() method are the array name, the index of the first element to be filled(inclusive), the index of the last element to be filled(exclusive) and the value that is to be stored in the array elements.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Arrays; public class Demo { public static void main(String[] argv) throws Exception { ... Read More

81 Views
The headset() method returns elements up to a limit defined as a parameter.First, create a NavigableSet and add elements −NavigableSet set = new TreeSet(); set.add(10); set.add(25); set.add(40); set.add(55); set.add(70); set.add(85); set.add(100);Now, use the headset() method −set.headSet(55));The following is an example −Example Live Demoimport java.util.NavigableSet; import java.util.TreeSet; public class Demo { public static void main(String[] args) { NavigableSet set = new TreeSet(); set.add(10); set.add(25); set.add(40); set.add(55); set.add(70); set.add(85); set.add(100); System.out.println("Returned Value = " + ... Read More