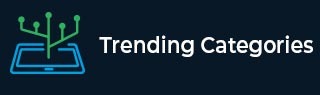
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

393 Views
The extreme elements in a list are the maximum and minimum elements. These can be obtained using the java.util.Collections.max() and java.util.Collections.min() methods respectively.The Collections.max() method contains a single parameter i.e. the list whose maximum element is to be found and it returns the maximum element from the list. The Collections.min() method contains a single parameter i.e. the list whose minimum element is to be found and it returns the minimum element from the list.A program that demonstrates this is given as follows:Exampleimport java.util.Arrays; import java.util.Collections; import java.util.List; public class Demo { public static void main(String args[]) { ... Read More

8K+ Views
A list can be sorted in reverse order i.e. descending order using the java.util.Collections.sort() method. This method requires two parameters i.e. the list to be sorted and the Collections.reverseOrder() that reverses the order of an element collection using a Comparator.The ClassCastException is thrown by the Collections.sort() method if there are mutually incomparable elements in the list.A program that demonstrates this is given as follows:Example Live Demoimport java.util.ArrayList; import java.util.Collections; import java.util.List; public class Demo { public static void main(String args[]) { List aList = new ArrayList(); ... Read More

94 Views
A list can be sorted in ascending order using the java.util.Collections.sort() method. This method requires a single parameter i.e. the list to be sorted and no value is returned. The ClassCastException is thrown by the Collections.sort() method if there are mutually incomparable elements in the list.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.Collections; import java.util.List; public class Demo { public static void main(String args[]) { List aList = new ArrayList(); aList.add("James"); aList.add("Harry"); aList.add("Susan"); aList.add("Emma"); aList.add("Peter"); ... Read More

220 Views
Binary Search can be performed in Java using the method java.util.Collections.binarySearch(). This method requires two parameters i.e. the list in which binary search is to be performed and the element that is to be searched. It returns the index of the element if it is in the list and returns -1 if it is not in the list.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.Collections; import java.util.List; public class Demo { public static void main(String args[]) { List aList = new ArrayList(); aList.add("James"); aList.add("George"); ... Read More

549 Views
The objects of a user defined class can be ordered using the Comparator interface in Java. The java.util.Collections.reverseOrder() method reverses the order of an element collection using a Comparator.A program that demonstrates this is given as follows −Exampleimport java.util.Arrays; import java.util.Collections; import java.util.Comparator; public class Demo { public static void main(String args[]) throws Exception { Comparator comparator = Collections.reverseOrder(); { "John", "Amy", "Susan", "Peter" }; int n = str.length; System.out.println("The array elements are: "); for (int i = 0; i < n; i++) { ... Read More

77 Views
A Vector can be cloned using the java.util.Vector.clone() method. This method does not take any parameters but returns a clone of the specified Vector instance as an object.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Vector; public class Demo { public static void main(String args[]) { Vector vec1 = new Vector(); vec1.add(7); vec1.add(3); vec1.add(5); vec1.add(9); vec1.add(2); Vector vec2 = (Vector) vec1.clone(); System.out.println("The Vector vec1 elements are: " + vec1); ... Read More

929 Views
A Vector can be converted into an Array using the java.util.Vector.toArray() method. This method requires no parameters and it returns an Array that contains all the elements of the Vector in the correct order.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Vector; public class Demo { public static void main(String args[]) { Vector vec = new Vector(); vec.add(7); vec.add(3); vec.add(5); vec.add(2); vec.add(8); Object[] arr = vec.toArray(); System.out.println("The Array elements are: "); ... Read More

359 Views
The minimum element of a Vector can be obtained using the java.util.Collections.min() method. This method contains a single parameter i.e. the Vector whose minimum element is determined and it returns the minimum element from the Vector.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Collections; import java.util.Vector; public class Demo { public static void main(String args[]) { Vector vec = new Vector(); vec.add(7); vec.add(3); vec.add(9); vec.add(5); vec.add(8); System.out.println("The Vector elements are: " + vec); ... Read More

817 Views
The maximum element of a Vector can be obtained using the java.util.Collections.max() method. This method contains a single parameter i.e. the Vector whose maximum element is determined and it returns the maximum element from the Vector.A program that demonstrates this is given as follows −Example Live Demoimport java.util.Collections; import java.util.Vector; public class Demo { public static void main(String args[]) { Vector vec = new Vector(); vec.add(7); vec.add(3); vec.add(9); vec.add(5); vec.add(8); System.out.println("The Vector elements are: " + vec); ... Read More

105 Views
A ListIterator can be used to traverse the elements in the forward direction as well as the reverse direction in a Vector. The method hasNext( ) in ListIterator returns true if there are more elements in the Vector while traversing in the forward direction and false otherwise. The method next( ) returns the next element in the Vector and advances the cursor position.A program that demonstrates this is given as follows −Exampleimport java.util.ListIterator; import java.util.Vector; public class Demo { public static void main(String args[]) { Vector vec = new Vector(); vec.add(3); ... Read More