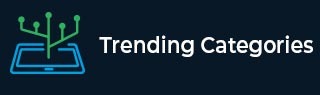
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

168 Views
A class declaration can contain a method that has a parameter in Java. A program that demonstrates this is given as follows:Example Live Democlass Message { public void messagePrint(String msg) { System.out.println("The message is: " + msg); } } public class Demo { public static void main(String args[]) { Message m = new Message(); m.messagePrint("Java is fun"); } }OutputThe message is: Java is funNow let us understand the above program.The Message class is created with a single member ... Read More

2K+ Views
Method overriding can be prevented by using the final keyword with a method. In other words, a final method cannot be overridden.A program that demonstrates this is given as follows:Exampleclass A { int a = 8; final void print() { System.out.println("Value of a: " + a); } } class B extends A { int b = 3; void print() { System.out.println("Value of b: " + b); } } public class Demo { public static void main(String args[]) { B ... Read More

862 Views
A variable cannot be modified after it is declared as final. In other words, a final variable is constant. So, a final variable must be initialized and an error occurs if there is any attempt to change the value.A program that demonstrates a final variable in Java is given as follows −Example Live Demopublic class Demo { public static void main(String[] args) { final double PI = 3.141592653589793; System.out.println("The value of pi is: " + PI); } }OutputThe value of pi is: 3.141592653589793Now let us understand the above program.In the main() method in ... Read More

132 Views
The representation of the regular expressions are available in the java.util.regex.Pattern class. This class basically defines a pattern that is used by the regex engine.A program that demonstrates using the Pattern class to match in Java is given as follows −Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("p+"); Matcher m = p.matcher("apples and peaches are tasty"); System.out.println("The input string is: apples and peaches are tasty"); System.out.println("The Regex is: p+ "); System.out.println(); ... Read More

275 Views
A class declaration can contain a single method. A program that demonstrates this is given as follows:Example Live Democlass Message { public void messagePrint() { System.out.println("This is a class with a single method"); } } public class Demo { public static void main(String args[]) { Message m = new Message(); m.messagePrint(); } }OutputThis is a class with a single methodNow let us understand the above program.The Message class is created with a single member function messagePrint(). A code snippet which demonstrates this is as follows −class Message { ... Read More

185 Views
The Matcher.find(int) method finds the subsequence in an input sequence after the subsequence number that is specified as a parameter. This method is available in the Matcher class that is available in the java.util.regex package.The Matcher.find(int) method has one parameter i.e. the subsequence number after which the subsequence is obtained and it returns true is the required subsequence is obtained else it returns false.A program that demonstrates the method Matcher.find(int) in Java regular expressions is given as follows −Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = ... Read More

108 Views
An example of a read-only collection can be an unmodifiable ArrayList. The unmodifiable view of the specified ArrayList can be obtained by using the method java.util.Collections.unmodifiableList(). This method has a single parameter i.e. the ArrayList and it returns the unmodifiable view of that ArrayList.A program that demonstrates this is given as follows −Example Live Demoimport java.util.ArrayList; import java.util.Collections; import java.util.List; public class Demo { public static void main(String args[]) throws Exception { List aList = new ArrayList(); aList.add("Apple"); aList.add("Mango"); aList.add("Guava"); aList.add("Orange"); aList.add("Peach"); ... Read More

127 Views
Multiple characters can be treated as a single unit using groups in Java regular expressions. The method java.time.Matcher.group() is used to find the subsequence in the input sequence string that is a match to the required pattern.A program that demonstrates groups in Java regular expressions is given as follows −Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("\w\d"); Matcher m = p.matcher("I am f9"); System.out.println("The input string is: I am f9"); System.out.println("The Regex is: \w\d"); ... Read More

12K+ Views
A thread can be created by implementing the Runnable interface and overriding the run() method.The current thread is the currently executing thread object in Java. The method currentThread() of the Thread class can be used to obtain the current thread. This method requires no parameters.A program that demonstrates this is given as follows −Example Live Demopublic class Demo extends Thread { public void run() { for (int i = 0; i < 5; i++) { System.out.println("The Thread name is " + Thread.currentThread().getName()); } } public static void main(String[] ... Read More

91 Views
The method java.time.Matcher.matches() matches the given region against the specified pattern. It returns true if the region sequence matches the pattern of the Matcher and false otherwise.A program that demonstrates the method Matcher.matches() in Java regular expressions is given as follows −Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("Apple"); String str1 = "apple"; String str2 = "Apple"; String str3 = "APPLE"; Matcher m1 = p.matcher(str1); Matcher m2 = p.matcher(str2); ... Read More