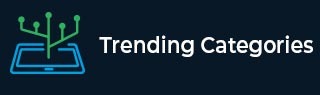
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

462 Views
A method that accepts a variable number of Object arguments in Java is a form of variable length arguments(Varargs). These can have zero or multiple Object type arguments.A program that demonstrates this is given as follows:Example Live Demopublic class Demo { public static void Varargs(Object... args) { System.out.println("Number of Object arguments are: " + args.length); System.out.println("The Object argument values are: "); for (Object i : args) System.out.println(i); } public static void main(String args[]) { Varargs("Apples", "4", "All"); Varargs("Half of", 3, ... Read More

100 Views
A method with variable length arguments(Varargs) in Java can have zero or multiple arguments. Variable length arguments(Varargs) can also be used with standard arguments However they must be the last argument in the argument list. Moreover, there can only be one variable length argument in the method.A program that demonstrates this is given as follows:Example Live Demopublic class Demo { public static void Varargs(int i, String... str) { System.out.println("Number of Vararg are: " + i); System.out.println("The argument values are: "); for (String s : str) System.out.println(s); ... Read More

4K+ Views
A method with variable length arguments(Varargs) in Java can have zero or multiple arguments. Variable length arguments are most useful when the number of arguments to be passed to the method is not known beforehand. They also reduce the code as overloaded methods are not required.A program that demonstrates this is given as follows:Example Live Demopublic class Demo { public static void Varargs(String... str) { System.out.println("Number of arguments are: " + str.length); System.out.println("The argument values are: "); for (String s : str) System.out.println(s); } ... Read More

777 Views
In method overloading, the class can have multiple methods with the same name but the parameter list of the methods should not be the same.Overloaded methods can be used to print an array of different types in Java by making sure that the parameter list of the methods contains different types of arrays that can be printed by the method.A program that demonstrates this is given as follows −Example Live Demopublic class Demo { public static void arrPrint(Integer[] arr) { System.out.print("The Integer array is: "); for (Integer i : arr) ... Read More

2K+ Views
In method overloading, the class can have multiple methods with the same name but the parameter list of the methods should not be the same. One way to make sure that the parameter list is different is to change the order of the arguments in the methods.A program that demonstrates this is given as follows −Example Live Democlass PrintValues { public void print(int val1, char val2) { System.out.println("The int value is: " + val1); System.out.println("The char value is: " + val2); } public void print(char val1, int val2) { ... Read More

2K+ Views
Method overloading in a class contains multiple methods with the same name but the parameter list of the methods should not be the same. One of these methods can have a long parameter in their parameter list.A program that demonstrates this is given as follows −Example Live Democlass PrintValues { public void print(int val) { System.out.println("The int value is: " + val); } public void print(long val) { System.out.println("The long value is: " + val); } } public class Demo { public static void main(String[] args) { ... Read More

411 Views
A class can have multiple methods with the same name but the parameter list of the methods should not be the same. This is known as method overloading. Method overloading is somewhat similar to constructor overloading.A program that demonstrates this is given as follows −Example Live Democlass PrintValues { public void print(int val) { System.out.println("The value is: " + val); } public void print(double val) { System.out.println("The value is: " + val); } public void print(char val) { System.out.println("The value is: " + val); } } ... Read More

310 Views
A copy constructor can be used to duplicate an object in Java. The copy constructor takes a single parameter i.e. the object of the same class that is to be copied. However, the copy constructor can only be explicitly created by the programmer as there is no default copy constructor provided by Java.A program that demonstrates this is given as follows −Example Live Democlass NumberValue { private int num; public NumberValue(int n) { num = n; } public NumberValue(NumberValue obj) { num = obj.num; } public void display() { ... Read More

11K+ Views
There can be multiple constructors in a class. However, the parameter list of the constructors should not be same. This is known as constructor overloading.A program that demonstrates this is given as follows −Example Live Democlass NumberValue { private int num; public NumberValue() { num = 6; } public NumberValue(int n) { num = n; } public void display() { System.out.println("The number is: " + num); } } public class Demo { public static void main(String[] args) { NumberValue obj1 = ... Read More

503 Views
The method java.time.Matcher.group() is used to find the subsequence in the input sequence string that is a match to the required pattern. This method returns the subsequence that is matched by the previous match which can even be empty.A program that demonstrates the method Matcher.group() in Java regular expressions is given as follows:Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("\w\d"); Matcher m = p.matcher("This is gr8"); System.out.println("The input ... Read More