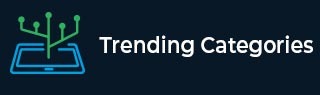
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

1K+ Views
A Vector can be created from an Array using the java.util.Arrays.asList() method.A program that demonstrates this is given as follows:Example Live Demoimport java.util.Arrays; import java.util.Vector; public class Demo { public static void main(String args[]) { Integer[] arr = { 3, 1, 9, 6, 4, 8, 7 }; Vector vec = new Vector(Arrays.asList(arr)); System.out.println("The Vector elements are: " + vec); } }OutputThe Vector elements are: [3, 1, 9, 6, 4, 8, 7]Now let us understand the above program.The Integer Array arr[] is defined. Then a Vector is created using the ... Read More

217 Views
A nested class in Java is of two types i.e. Static nested class and Inner class. A static nested class is a nested class that is declared as static. A nested nested class cannot access the data members and methods of the outer class.A program that demonstrates a static nested class is given as follows:Example Live Demopublic class Class1 { static class Class2 { public void func() { System.out.println("This is a static nested class"); } } public static void main(String args[]) { Class1.Class2 obj = ... Read More

175 Views
The static field variable is a class level variable and it belongs to the class not the class object.So the static field variable is common to all the class objects i.e. a single copy of the static field variable is shared among all the class objects.A program that demonstrates referencing the static field variable after declaration is given as follows:Example Live Demopublic class Demo { static int a = 7; static int b = a + 5; public static void main(String[] args) { System.out.println("a = " + a); System.out.println("b = " + ... Read More

7K+ Views
The static variable is a class level variable and it is common to all the class objects i.e. a single copy of the static variable is shared among all the class objects.A static method manipulates the static variables in a class. It belongs to the class instead of the class objects and can be invoked without using a class object.The static initialization blocks can only initialize the static instance variables. These blocks are only executed once when the class is loaded.A program that demonstrates this is given as follows:Example Live Demopublic class Demo { static int x = 10; ... Read More

8K+ Views
Instance variables are initialized using initialization blocks. However, the static initialization blocks can only initialize the static instance variables. These blocks are only executed once when the class is loaded. There can be multiple static initialization blocks in a class that is called in the order they appear in the program.A program that demonstrates a static initialization block in Java is given as follows:Example Live Demopublic class Demo { static int[] numArray = new int[10]; static { System.out.println("Running static initialization block."); for (int i = 0; i < numArray.length; i++) { ... Read More

2K+ Views
Instance variables are initialized using initialization blocks. These blocks are executed when the class object is created and before the invocation of the class constructor. Also, it is not necessary to have initialization blocks in the class.A program that demonstrates a non-static initialization block in Java is given as follows:Example Live Demopublic class Demo { static int[] numArray = new int[10]; { System.out.println("Running non-static initialization block."); for (int i = 0; i < numArray.length; i++) { numArray[i] = (int) (100.0 * Math.random()); } } ... Read More

222 Views
The this keyword in Java is mainly used to refer to the current instance variable of the class. It can also be used to implicitly invoke the method or to invoke the constructor of the current class.A program that demonstrates the this keyword in Java is given as follows:Example Live Democlass Student { private int rno; private String name; public Student(int rno, String name) { this.rno = rno; this.name = name; } public void display() { System.out.println("Roll Number: " + rno); System.out.println("Name: " + ... Read More

9K+ Views
The fibonacci series is a series in which each number is the sum of the previous two numbers. The number at a particular position in the fibonacci series can be obtained using a recursive method.A program that demonstrates this is given as follows:Example Live Demopublic class Demo { public static long fib(long n) { if ((n == 0) || (n == 1)) return n; else return fib(n - 1) + fib(n - 2); } public static void main(String[] args) { System.out.println("The ... Read More

25K+ Views
An interface contains variables and methods like a class but the methods in an interface are abstract by default unlike a class. An interface extends another interface like a class implements an interface in interface inheritance.A program that demonstrates extending interfaces in Java is given as follows:Example Live Demointerface A { void funcA(); } interface B extends A { void funcB(); } class C implements B { public void funcA() { System.out.println("This is funcA"); } public void funcB() { System.out.println("This is funcB"); } } public class Demo { ... Read More

608 Views
A method with variable length arguments(Varargs) can have zero or multiple arguments. Also, Varargs methods can be overloaded if required.A program that demonstrates this is given as follows:Example Live Demopublic class Demo { public static void Varargs(int... args) { System.out.println("Number of int arguments are: " + args.length); System.out.println("The int argument values are: "); for (int i : args) System.out.println(i); } public static void Varargs(char... args) { System.out.println("Number of char arguments are: " + args.length); System.out.println("The char argument values are: "); ... Read More