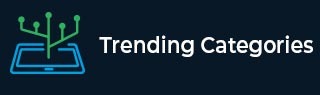
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

263 Views
The specified string can be split around a particular match for a regex using the String.split() method. This method has a single parameter i.e. regex and it returns the string array obtained by splitting the input string around a particular match for the regex.A program that demonstrates splitting a string is given as follows:Example Live Demopublic class Demo { public static void main(String args[]) { String regex = "_"; String strInput = "The_sky_is_blue"; System.out.println("Regex: " + regex); System.out.println("Input string: " + strInput); String[] strArr = ... Read More

63 Views
The specified input sequence can be split around a particular match for a pattern using the java.util.regex.Pattern.split() method. This method has a single parameter i.e. the input sequence to split and it returns the string array obtained by splitting the input sequence around a particular match for a pattern.A program that demonstrates the method Pattern.split() in Java regular expressions is given as follows:Example Live Demoimport java.util.regex.Pattern; public class Demo { public static void main(String[] args) { String regex = "_"; String input = "Oranges_are_orange"; System.out.println("Regex: " + regex); ... Read More

3K+ Views
The Email address can be validated using the java.util.regex.Pattern.matches() method. This method matches the regular expression for the E-mail and the given input Email and returns true if they match and false otherwise.A program that demonstrates this is given as follows:Example Live Demopublic class Demo { static boolean isValid(String email) { String regex = "^[\w-_\.+]*[\w-_\.]\@([\w]+\.)+[\w]+[\w]$"; return email.matches(regex); } public static void main(String[] args) { String email = "john123@gmail.com"; System.out.println("The E-mail ID is: " + email); System.out.println("Is the above E-mail ID valid? " + ... Read More

148 Views
The java.util.regex.Pattern.matches() method matches the regular expression and the given input. It has two parameters i.e. the regex and the input. It returns true if the regex and the input match and false otherwise.A program that demonstrates the method Pattern.matches() in Java regular expressions is given as follows:Example Live Demoimport java.util.regex.Pattern; public class Demo { public static void main(String args[]) { String regex = "a*b"; String input = "aaab"; System.out.println("Regex: " + regex); System.out.println("Input: " + input); boolean match = Pattern.matches(regex, input); ... Read More

67 Views
The Matcher can be reset using the java.util.regex.Matcher.reset() method. This method returns the reset Matcher.A program that demonstrates the method Matcher.reset() in Java regular expressions is given as follows:Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class MainClass { public static void main(String args[]) { Pattern p = Pattern.compile("(a*b)"); Matcher m = p.matcher("caaabcccab"); System.out.println("The input string is: caaabcccab"); System.out.println("The Regex is: (a*b)"); System.out.println(); while (m.find()) { System.out.println(m.group()); } m.reset(); System.out.println("The ... Read More

343 Views
The find() method finds the subsequence in an input sequence that matches the pattern required. This method is available in the Matcher class that is available in the java.util.regex package.A program that demonstrates the method Matcher.find() in Java regular expressions is given as follows:Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("Sun"); Matcher m = p.matcher("The Earth revolves around the Sun"); System.out.println("Subsequence: Sun"); System.out.println("Sequence: The Earth revolves around the Sun"); if (m.find()) ... Read More

89 Views
The offset value after the last character is matched from the sequence according to the regex is returned by the method java.util.regex.Matcher.end(). This method requires no arguments. If no match occurs or if the match operation fails then the IllegalStateException is thrown.A program that demonstrates the method Matcher.end() Java regular expressions is given as follows:Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("(a*b)"); Matcher m = p.matcher("caaabccaab"); System.out.println("The input string is: caaabccaab"); System.out.println("The Regex is: (a*b)"); ... Read More

113 Views
A character class is set of characters that are enclosed inside square brackets. The characters in a character class specify the characters that can match with a character in an input string for success. An example of a character class is [a-z] which denotes the alphabets a to z.A program that demonstrates a character class in Java Regular Expressions is given as follows:Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("[a-z]+"); Matcher m = p.matcher("the sky is blue"); System.out.println("The input ... Read More

125 Views
In general, the ? quantifier represents 0 or 1 occurrences of the specified pattern. For example - X? means 0 or 1 occurrences of X.The regex "t.+?m" is used to find the match in the string "tom and tim are best friends" using the ? quantifier.A program that demonstrates this is given as follows:Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("t.+?m"); Matcher m = p.matcher("tom and tim are best friends"); System.out.println("The input string is: tom and tim are best ... Read More

50 Views
The find() method finds the subsequence in an input sequence that matches the pattern required. This method is available in the Matcher class that is available in the java.util.regex packageA program that uses the find() method to find a subsequence in Java is given as follows:Example Live Demoimport java.util.regex.Matcher; import java.util.regex.Pattern; public class Demo { public static void main(String args[]) { Pattern p = Pattern.compile("cool"); Matcher m = p.matcher("Java is cool"); System.out.println("Subsequence: cool"); System.out.println("Sequence: Java is cool"); if (m.find()) System.out.println("Subsequence found"); ... Read More