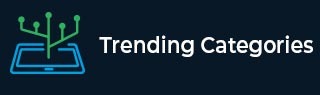
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

156 Views
A file or directory with the required abstract pathname can be deleted when the program ends i.e. after the virtual machine terminates using the method java.io.File.deleteOnExit(). This method requires no parameters and it does not return a value.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); System.out.println("File: " + file); file.deleteOnExit(); } catch(Exception e) { ... Read More

90 Views
The java.io.File class has display constants File.separatorChar and File.pathSeparatorChar mainly. The File.separatorChar is ‘/’ and the File.pathSeparatorChar is ‘:’ for Unix.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { System.out.println("File.pathSeparatorChar = " + File.pathSeparatorChar); System.out.println("File.separatorChar = " + File.separatorChar); } catch(Exception e) { e.printStackTrace(); } } }The output of the above program is as follows −OutputFile.pathSeparatorChar = : File.separatorChar = /Now let ... Read More

10K+ Views
A new empty file with the required abstract path name can be created using the method java.io.File.createNewFile(). This method requires no parameters and it returns true if the file is newly created and it did not exist previously. If the file existed previously, it returns false.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); System.out.println("File: " + file); } catch(Exception ... Read More

695 Views
A directory can be created with the required abstract path name using the method java.io.File.mkdir(). This method requires no parameters and it returns true on the success of the directory creation or false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("c:\demo1\"); file.createNewFile(); boolean flag = file.mkdir(); System.out.print("Directory created? " + flag); } catch(Exception e) { ... Read More

2K+ Views
The method java.io.File.renameTo() is used to rename a file or directory. This method requires a single parameter i.e. the name that the file or directory is renamed to and it returns true on the success of the renaming or false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file1 = new File("demo1.txt"); File file2 = new File("demo2.txt"); file1.createNewFile(); file2.createNewFile(); ... Read More

73 Views
The length of the current file specified by the abstract path name can be displayed using the method java.io.File.length(). The method returns the file length in bytes and does not require any parameters.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); System.out.println("Length of file: " + file.length()); } catch(Exception e) { e.printStackTrace(); } ... Read More

985 Views
The method java.io.File.getAbsoluteFile() is used to get the File object with the absolute path for the directory or file. This method requires no parameters.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { File file = new File("C:" + File.separator + "jdk11.0.2" + File.separator, "demo1.java"); System.out.println("The absolute file is: " + file.getAbsoluteFile()); } }The output of the above program is as follows −OutputThe absolute file is:/C:/jdk11.0.2/demo1.javaNow let us understand the above program.The file object with the absolute path is obtained ... Read More

2K+ Views
The method java.io.File.getAbsolutePath() is used to obtain the absolute path name of a file or directory in the form of a string. This method requires no parameters.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { File file = new File("C:" + File.separator + "jdk11.0.2" + File.separator, "demo1.java"); System.out.println("The absolute path name is: " + file.getAbsolutePath()); } }The output of the above program is as follows −OutputThe absolute path name is:/C:/jdk11.0.2/demo1.javaNow let us understand the above program.The absolute pathname of ... Read More

589 Views
The method java.io.File.canWrite() is used to check whether the file can be written to in Java. This method returns true if the file specified by the abstract path name can be written to by an application and false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); System.out.println("The file can be written to? " + file.canWrite()); } catch(Exception e) { ... Read More

429 Views
The method java.io.File.canRead() is used to check whether the file can be read in Java. This method returns true if the file specified by the abstract path name can be read by an application and false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); System.out.println("The file can be read? " + file.canRead()); } catch(Exception e) { ... Read More