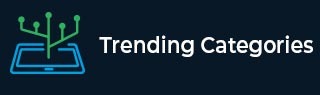
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

734 Views
A file can be set to read-only by using the method java.io.File.setReadOnly(). This method requires no parameters and it returns true if the file is set to read-only and false otherwise. The method java.io.File.canWrite() is used to check whether the file can be written to in Java and if not, then the file is confirmed to be read-only.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { boolean flag; try { File file = new File("demo1.txt"); ... Read More

232 Views
A file or directory can be deleted on termination of the program i.e. after the virtual machine terminates using the method java.io.File.deleteOnExit(). This method requires no parameters and it does not return a value.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); System.out.println("File: " + file); file.deleteOnExit(); } catch(Exception e) { e.printStackTrace(); ... Read More

143 Views
The contents of a directory can be obtained using the method java.io.File.listFiles(). This method requires no parameters and it returns the abstract path names that specify the files and directories in the required directory.A program that demonstrates this is given as follows −Exampleimport java.io.File; public class Demo { public static void main(String[] args) { File directory = new File("C:\JavaProgram"); File[] contents = directory.listFiles(); for (File c : contents) { if(c.isFile()) System.out.println(c + " is a file"); ... Read More

236 Views
The name of the specified file or directory can be obtained using the method java.io.File.getName(). The getName() returns the name of the file or the directory and it requires no parameters.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { File file = new File("C:" + File.separator + "JavaProgram" + File.separator, "demo1.txt"); System.out.println("File name: " + file.getName()); } }The output of the above program is as follows −OutputFile name: demo1.txtNow let us understand the above program.The name of the specified ... Read More

207 Views
The name of the parent directory of the file or directory can be obtained using the method java.io.File.getParent(). This method returns the parent directory pathname string or null if there is no parent named.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { File file = new File("C:" + File.separator + "JavaProgram" + File.separator, "demo1.txt"); System.out.println("File: " + file); System.out.println("Parent: " + file.getParent()); } }The output of the above program is as follows −OutputFile: C:\JavaProgram\demo1.txt Parent: C:\JavaProgramNow ... Read More

2K+ Views
The method java.io.File.canRead() is used to check if a file or directory is readable in Java. This method returns true if the file specified by the abstract path name can be read by an application and false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); System.out.println("The file can be read? " + file.canRead()); } catch(Exception e) { ... Read More

6K+ Views
The method java.io.File.exists() is used to check whether a file or a directory exists or not. This method returns true if the file or directory specified by the abstract path name exists and false if it does not exist.A program that demonstrates this is given as follows −Exampleimport java.io.File; public class Demo { public static void main(String[] args) { try { File file = new File("c:/JavaProgram/demo1.txt"); file.createNewFile(); System.out.println(file.exists()); } catch(Exception e) { e.printStackTrace(); } ... Read More

385 Views
The method java.io.File.isFile() is used to check whether the given file is an existing file in Java. Similarly, the method java.io.File.isDirectory() is used to check whether the given file is a directory in Java. Both of these methods require no parameters.A program that demonstrates this is given as follows −Examplepublic class Demo { public static void main(String[] args) { try { File file = new File("demo1.txt"); file.createNewFile(); boolean fileFlag = file.isFile(); if (fileFlag) { ... Read More

105 Views
The method java.io.File.mkdirs() is used to create the specified directories, including the necessary parent directories. This method requires no parameters and it returns true on the success of the directories creation or false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static void main(String[] args) { String recursiveDirectories = "D:\a\b\c\d"; File file = new File(recursiveDirectories); boolean flag = file.mkdirs(); System.out.println("The directories are created recursively? " + flag); } }The output of the above program is as follows ... Read More

3K+ Views
The method removeExtension() is used to strip a filename of its extension after the last dot. This method requires a single parameter i.e. the file name and it returns the file name without its extension.A program that demonstrates this is given as follows −Example Live Demoimport java.io.File; public class Demo { public static String removeExtension(String fname) { int pos = fname.lastIndexOf('.'); if(pos > -1) return fname.substring(0, pos); else return fname; } public static void main(String[] args) { System.out.println(removeExtension("c:\JavaProgram\demo1.txt")); ... Read More