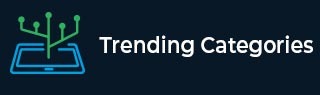
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

58 Views
The offset of the first element of the buffer inside the buffer array is obtained using the method arrayOffset() in the class java.nio.FloatBuffer. If the buffer backed by the array is read-only, then the ReadOnlyBufferException is thrown.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { FloatBuffer buffer = FloatBuffer.allocate(5); buffer.put(4.5F); buffer.put(1.2F); buffer.put(3.9F); ... Read More

65 Views
The buffer can be compacted using the compact() method in the class java.nio.FloatBuffer. This method does not require a parameter and it returns the new compacted FloatBuffer with the same content as the original buffer. If the buffer is read-only, then the ReadOnlyBufferException is thrown.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { FloatBuffer buffer = FloatBuffer.allocate(n); buffer.put(1.2F); buffer.put(3.9F); ... Read More

82 Views
A new FloatBuffer can be allocated using the method allocate() in the class java.nio.FloatBuffer. This method requires a single parameter i.e. the capacity of the buffer. It returns the new FloatBuffer that is allocated. If the capacity provided is negative, then the IllegalArgumentException is thrown.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { FloatBuffer buffer = FloatBuffer.allocate(n); buffer.put(4.5F); buffer.put(1.2F); ... Read More

90 Views
A buffer can be compared with another buffer using the method compareTo() in the class java.nio.FloatBuffer. This method returns a negative integer if the buffer is less than the given buffer, zero if the buffer is equal to the given buffer and a positive integer if the buffer is greater than the given buffer.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { FloatBuffer buffer1 = FloatBuffer.allocate(n); ... Read More

125 Views
The equality of two buffers can be checked using the method equals() in the class java.nio.IntBuffer. Two buffers are equal if they have the same type of elements, the same number of elements and same sequence of elements. The method equals() returns true if the buffers are equal and false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { IntBuffer buffer1 = IntBuffer.allocate(n); buffer1.put(8); ... Read More

60 Views
The offset of the first element of the buffer inside the buffer array is obtained using the method arrayOffset() in the class java.nio.IntBuffer. If the buffer backed by the array is read-only, then the ReadOnlyBufferException is thrown.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { IntBuffer buffer = IntBuffer.allocate(5); buffer.put(8); buffer.put(1); buffer.put(3); ... Read More

78 Views
The required value can be written at the current position of the buffer and then the current position is incremented using the method put() in the class java.nio.IntBuffer. This method requires a single parameter i.e. the value to be written in the buffer and it returns the buffer in which the value is inserted.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { IntBuffer buffer = IntBuffer.allocate(5); ... Read More

102 Views
The value at the current position of the buffer is read and then incremented using the method get() in the class java.nio.IntBuffer. This method returns the value that is at the current buffer position. Also, the BufferUnderflowException is thrown if underflow situation occurs.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { IntBuffer buffer = IntBuffer.allocate(n); buffer.put(8); buffer.put(1); ... Read More

307 Views
An int array can be wrapped into a buffer using the method wrap() in the class java.nio.IntBuffer. This method requires a single parameter i.e. the array to be wrapped into a buffer and it returns the new buffer created. If the returned buffer is modified, then the contents of the array are also similarly modified and vice versa.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { try { int[] arr = { 8, 1, 3, 7, 5 ... Read More

95 Views
A new IntBuffer with the content as a shared subsequence of the original IntBuffer can be created using the method slice() in the class java.nio.IntBuffer. This method returns the new IntBuffer that is read-only if the original buffer is read-only and direct if the original buffer is direct.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { IntBuffer buffer1 = IntBuffer.allocate(n); buffer1.put(3); ... Read More