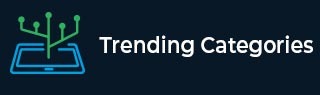
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

362 Views
The value at the current position of the buffer is read and then incremented using the method get() in the class java.nio.ByteBuffer. This method returns the value that is at the current buffer position. Also, the BufferUnderflowException is thrown if underflow situation occurs.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ByteBuffer buffer = ByteBuffer.allocate(n); ... Read More

305 Views
A duplicate buffer of a buffer can be created using the method duplicate() in the class java.nio.ByteBuffer. This duplicate buffer is identical to the original buffer. The method duplicate() returns the duplicate buffer that was created.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ByteBuffer buffer1 = ByteBuffer.allocate(5); buffer1.put((byte)1); buffer1.put((byte)2); buffer1.put((byte)3); buffer1.put((byte)4); ... Read More

175 Views
The buffer can be compacted using the compact() method in the class java.nio.ByteBuffer. This method does not require a parameter and it returns the new compacted ByteBuffer with the same content as the original buffer. If the buffer is read-only, then the ReadOnlyBufferException is thrown.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ByteBuffer buffer ... Read More

109 Views
A view of the ByteBuffer can be created as a ShortBuffer using the asShortBuffer() method in the class java.nio.ByteBuffer. This method requires no parameters and it returns a short buffer as required. This buffer reflects the changes made to the original buffer and vice versa.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 50; try { ByteBuffer bufferB = ByteBuffer.allocate(n); ShortBuffer bufferS = bufferB.asShortBuffer(); ... Read More

369 Views
The equality of two buffers can be checked using the method equals() in the class java.nio.ByteBuffer. Two buffers are equal if they have the same type of elements, the same number of elements and the same sequence of elements. The method equals() returns true if the buffers are equal and false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ... Read More

116 Views
The offset of the first element of the buffer inside the buffer array is obtained using the method arrayOffset() in the class java.nio.ByteBuffer. If the buffer backed by the array is read-only, then the ReadOnlyBufferException is thrown.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ByteBuffer buffer = ByteBuffer.allocate(5); ... Read More

67 Views
It can be checked if a buffer has the backing of an accessible short array by using the method hasArray() in the class java.nio.ShortBuffer. This method returns true if the buffer has the backing of an accessible double array and false otherwise.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ShortBuffer buffer = ShortBuffer.allocate(5); ... Read More

56 Views
A buffer can be compared with another buffer using the method compareTo() in the class java.nio.ShortBuffer. This method returns a negative integer if the buffer is less than the given buffer, zero if the buffer is equal to the given buffer and a positive integer if the buffer is greater than the given buffer.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ... Read More

61 Views
A duplicate buffer of a buffer can be created using the method duplicate() in the class java.nio.ShortBuffer. This duplicate buffer is identical to the original buffer. The method duplicate() returns the duplicate buffer that was created.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ShortBuffer buffer1 = ShortBuffer.allocate(5); buffer1.put((short)12); buffer1.put((short)91); buffer1.put((short)25); buffer1.put((short)18); ... Read More

66 Views
A read-only short buffer can be created using the contents of a buffer with the method asReadOnlyBuffer() in the class java.nio.ShortBuffer. The new buffer cannot have any modifications as it is a read-only buffer. However, the capacity, positions, limits etc. of the new buffer are the same as the previous buffer.A program that demonstrates this is given as follows −Example Live Demoimport java.nio.*; import java.util.*; public class Demo { public static void main(String[] args) { int n = 5; try { ShortBuffer buffer = ShortBuffer.allocate(5); buffer.put((short)12); ... Read More