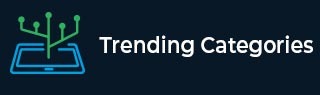
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

57 Views
The algorithm name for the KeyFactory can be obtained using the method getAlgorithm() in the class java.security.KeyFactory. This method requires no parameters and it returns the algorithm name for the KeyFactory.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; import java.security.spec.*; public class Demo { public static void main(String[] argv) throws Exception { try { KeyFactory kf = KeyFactory.getInstance("RSA"); String algorithm = kf.getAlgorithm(); System.out.println("The Algortihm is: " + algorithm); } catch (NoSuchAlgorithmException e) { ... Read More

57 Views
The algorithm name for the key pair generator can be obtained using the method getAlgorithm() in the class java.security.KeyPairGenerator. This method requires no parameters and it returns the algorithm name for the key pair generator.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { KeyPairGenerator kpGenerator = KeyPairGenerator.getInstance("DSA"); String algorithm = kpGenerator.getAlgorithm(); System.out.println("The Algorithm is: " + algorithm); } catch (NoSuchAlgorithmException e) { ... Read More

55 Views
The provider of the key pair object can be obtained using the getProvider() method in the class java.security.KeyPairGenerator. This method requires no parameters and it returns the provider of the key pair object.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { KeyPairGenerator kpGenerator = KeyPairGenerator.getInstance("DSA"); Provider p = kpGenerator.getProvider(); System.out.println("The Provider is: " + p.getName()); } catch (NoSuchAlgorithmException e) { ... Read More

139 Views
A KeyPairGenerator object with the key pairs for a particular algorithm can be obtained using the getInstance() method in the class java.security.KeyPairGenerator. This method requires a single parameter i.e. the algorithm name and it returns the KeyPairGenerator object created.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { KeyPairGenerator kpGenerator = KeyPairGenerator.getInstance("RSA"); String algorithm = kpGenerator.getAlgorithm(); System.out.println("The Algorithm is: " + algorithm); } catch (NoSuchAlgorithmException ... Read More

115 Views
A key pair can be generated using the generateKeyPair() method in the class java.security.KeyPairGenerator. This method requires no parameters and it returns the key pair that is generated. Every time the generateKeyPair() method is called, it generates a new key pair.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) throws Exception { try { KeyPairGenerator kpGenerator = KeyPairGenerator.getInstance("RSA"); KeyPair keyPair = kpGenerator.generateKeyPair(); System.out.println(keyPair); } catch (NoSuchAlgorithmException e) ... Read More

96 Views
A key pair can be generated using the genKeyPair() method in the class java.security.KeyPairGenerator. This method requires no parameters and it returns the key pair that is generated.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) throws Exception { try { KeyPairGenerator kpGenerator = KeyPairGenerator.getInstance("RSA"); KeyPair keyPair = kpGenerator.genKeyPair(); System.out.println(keyPair); } catch (NoSuchAlgorithmException e) { System.out.println("Error!!! NoSuchAlgorithmException"); ... Read More

301 Views
The number of random bytes as specified by the user can be obtained using the nextBytes() method in the class java.security.SecureRandom. This method requires a single parameter i.e. a random byte array and it returns the random bytes as specified by the user.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { SecureRandom sRandom = SecureRandom.getInstance("SHA1PRNG"); String s = "Apple"; byte[] arrB = s.getBytes(); ... Read More

90 Views
The seed bytes as required can be obtained using the method getSeed() in the class java.security.SecureRandom. This method requires a single parameter i.e. the number of seed bytes that need to be generated and it returns the seed bytes as required.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { SecureRandom sRandom = SecureRandom.getInstance("SHA1PRNG"); byte[] arrB = sRandom.getSeed(5); System.out.println("The Seed Bytes in array are: " + Arrays.toString(arrB)); ... Read More

361 Views
The random object can be reseeded using the setSeed() method in the class java.security.SecureRandom. This method requires a single parameter i.e. the required seed byte array and it returns the reseeded random object.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { SecureRandom sRandom = SecureRandom.getInstance("SHA1PRNG"); String str = "Apple"; byte[] arrB = str.getBytes(); sRandom.setSeed(arrB); byte[] arrSeed = sRandom.getSeed(5); ... Read More

59 Views
The provider for the SecureRandom object can be obtained using the method getProvider() in the class java.security.SecureRandom. This method requires no parameters and it returns the provider for the SecureRandom object.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { SecureRandom sRandom = SecureRandom.getInstance("SHA1PRNG"); Provider provider = sRandom.getProvider(); System.out.println("The Provider is: " + provider.getName()); } catch (NoSuchAlgorithmException e) { ... Read More