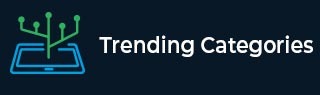
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

107 Views
The name and the version number of the provider in string form can be obtained using the method toString() in the class java.security.Provider. This method requires no parameters and it returns the name as well as the version number of the provider in string form.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { KeyPairGenerator kpGenerator = KeyPairGenerator.getInstance("DSA"); Provider p = kpGenerator.getProvider(); System.out.println("The name and version number of ... Read More

157 Views
The version number of the provider can be obtained using the method getVersion() in the class java.security.Provider. This method requires no parameter and it returns the version number of the provider as required.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { KeyPairGenerator kpGenerator = KeyPairGenerator.getInstance("DSA"); Provider p = kpGenerator.getProvider(); System.out.println("The version number is: " + p.getVersion()); } catch (NoSuchAlgorithmException e) { ... Read More

94 Views
The name of the provider can be obtained using the getName() method in the class java.security.Provider. This method requires no parameter and it returns the name of the provider as required.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { KeyPairGenerator kpGenerator = KeyPairGenerator.getInstance("DSA"); Provider p = kpGenerator.getProvider(); System.out.println("The Provider is: " + p.getName()); } catch (NoSuchAlgorithmException e) { ... Read More

403 Views
An easily readable description of the provider and its services can be obtained using the method getInfo() in the class java.security.Provider. This method requires no parameters and it returns a provider and services description.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) throws Exception { try { SecureRandom sRandom = SecureRandom.getInstance("SHA1PRNG"); Provider p = sRandom.getProvider(); System.out.println("The information is as follows:"); System.out.println(p.getInfo()); } catch ... Read More

283 Views
The value to which a key is mapped can be obtained using the get() method in the class java.security.Provider. This method requires a single parameter i.e. the key whose value is required. It returns the value to which the key is mapped or it returns null if there is no value to which the key is mapped.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { SecureRandom sRandom = SecureRandom.getInstance("SHA1PRNG"); Provider p ... Read More

77 Views
The entries in the Provider have an unmodifiable set view that can be obtained using the method entrySet() in the class java.security.Provider. This method requires no parameters and it returns the unmodifiable set view for the entries in the Provider.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { SecureRandom sRandom = SecureRandom.getInstance("SHA1PRNG"); Provider p = sRandom.getProvider(); Set set = p.entrySet(); Iterator i = ... Read More

74 Views
An enumeration of the values in the hash table can be obtained using the method elements() in the class java.security.Provider. This method requires no parameters and it returns the enumeration of the values in the hash table.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { SecureRandom sRandom = SecureRandom.getInstance("SHA1PRNG"); Provider p = sRandom.getProvider(); Enumeration enumeration; enumeration = p.elements(); System.out.println("The ... Read More

79 Views
The parameters can be generated using the method generateParameters() in the class java.security.AlgorithmParameterGenerator. This method requires no parameters and it returns the AlgorithmParameter object.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { AlgorithmParameterGenerator apGenerator = AlgorithmParameterGenerator.getInstance("DiffieHellman"); apGenerator.init(1024); AlgorithmParameters aParameters = apGenerator.generateParameters(); System.out.println(aParameters); } catch (NoSuchAlgorithmException e) { System.out.println("Error!!! NoSuchAlgorithmException"); } catch (ProviderException e) ... Read More

38 Views
The algorithm name for the parameter generator can be obtained using the method getAlgorithm() in the class java.security.AlgorithmParameterGenerator. This method requires no parameters and it returns the algorithm name in string form.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { AlgorithmParameterGenerator apGenerator = AlgorithmParameterGenerator.getInstance("DiffieHellman"); apGenerator.init(1024); String algorithm = apGenerator.getAlgorithm(); System.out.println("The Algorithm is: " + algorithm); } catch (NoSuchAlgorithmException e) { ... Read More

37 Views
The provider of the generator object can be obtained using the method getProvider() in the class java.security.AlgorithmParameterGenerator. This method requires no parameters and it returns the provider of the generator object.A program that demonstrates this is given as follows −Example Live Demoimport java.security.*; import java.util.*; public class Demo { public static void main(String[] argv) { try { AlgorithmParameterGenerator apGenerator = AlgorithmParameterGenerator.getInstance("DiffieHellman"); apGenerator.init(1024); Provider provider = apGenerator.getProvider(); System.out.println("The Provider is: " + provider); } catch (NoSuchAlgorithmException e) { ... Read More