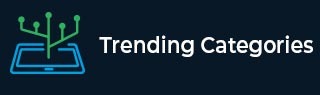
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

71 Views
A LocalTime object can be obtained using the nanoseconds of the day with the ofNanoOfDay() method in the LocalTime class in Java. This method requires a single parameter i.e. the nanoseconds of the day and it returns the LocalTime object for the nanoseconds of the dayA program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args){ long nanoSeconds = 1000000000; System.out.println("The nanoseconds of the day: " + nanoSeconds); System.out.println("The LocalTime is: " + LocalTime.ofNanoOfDay(nanoSeconds)); } }outputThe nanoseconds of the day: ... Read More

139 Views
The DoubleStream class in Java the following two forms of the of() methodThe following of() method returns a sequential DoubleStream containing a single element. Here is the syntaxstatic DoubleStream of(double t)Here, parameter t is the single element.The following of() method returns a sequential ordered stream whose elements are the specified valuesstatic DoubleStream of(double… values)Here, the parameter values are the elements of the new stream.To use the DoubleStream class in Java, import the following packageimport java.util.stream.DoubleStream;The following is an example to implement DoubleStream of() method in JavaExample Live Demoimport java.util.stream.DoubleStream; public class Demo { public static void main(String[] args) ... Read More

87 Views
The filter() method of the DoubleStream class returns a stream consisting of the elements of this stream that match the given predicate.The syntax is as followsDoubleStream filter(DoublePredicate predicate)The parameter predicate is a stateless predicate to apply to each element to determine if it should be included.To use the DoubleStream class in Java, import the following packageimport java.util.stream.DoubleStream;Create a DoubleStream and add some elementsDoubleStream doubleStream = DoubleStream.of(20.5, 35.7, 50.8, 67.9, 89.8, 93.1);Filter and display the element equal to a 50.8, if it’s presentdoubleStream.filter(a -> a == 50.8) The following is an example to implement DoubleStream filter() method in JavaExample Live Demoimport java.util.stream.DoubleStream; ... Read More

139 Views
The asDoubleStream() method in the IntStream class returns a DoubleStream consisting of the elements of this stream, converted to double.The syntax is as followsDoubleStream asDoubleStream()First, create an IntStreamIntStream intStream = IntStream.of(20, 30, 40, 50, 60, 70, 80);After that, convert it to double with asDoubleStream() methodDoubleStream doubleStream = intStream.asDoubleStream();The following is an example to implement IntStream asDoubleStream() method in JavaExample Live Demoimport java.util.*; import java.util.stream.IntStream; import java.util.stream.DoubleStream; public class Demo { public static void main(String[] args) { IntStream intStream = IntStream.of(20, 30, 40, 50, 60, 70, 80); DoubleStream doubleStream = intStream.asDoubleStream(); doubleStream.forEach(System.out::println); } }Output20.0 30.0 40.0 50.0 60.0 70.0 80.0

1K+ Views
Two LocalTime objects can be compared using the compareTo() method in the LocalTime class in Java. This method requires a single parameter i.e. the LocalTime object to be compared.If the first LocalTime object is greater than the second LocalTime object it returns a positive number, if the first LocalTime object is lesser than the second LocalTime object it returns a negative number and if both the LocalTime objects are equal it returns zero.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Main{ public static void main(String[] args){ LocalTime lt1 = LocalTime.parse("11:37:12"); ... Read More

315 Views
The forEachOrdered() method in Java assures that each element is processed in order for streams that have a defined encounter order.The syntax is as followsvoid forEachOrdered(IntConsumer action)Here, the action parameter is a non-interfering action to be performed on the elements.Create an IntStream and add elements to the streamIntStream intStream = IntStream.of(50, 70, 80, 100, 130, 150, 200);Now, use the forEachOrdered() method to display the stream elements in orderintStream.forEachOrdered(System.out::println);The following is an example to implement IntStream forEachOrdered() method in JavaExample Live Demoimport java.util.*; import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { ... Read More

59 Views
The clear() method is inherited from the AbstractList class. It allows you to remove all the elements from the list.The syntax is as followspublic void clear()To work with the AbstractSequentialList class in Java, you need to import the following packageimport java.util.AbstractSequentialList;The following is an example to implement AbstractSequentialList clear() method in JavaExample Live Demoimport java.util.LinkedList; import java.util.AbstractSequentialList; public class Demo { public static void main(String[] args) { AbstractSequentialList absSequential = new LinkedList(); absSequential.add(250); absSequential.add(320); absSequential.add(400); absSequential.add(550); absSequential.add(600); absSequential.add(700); ... Read More

87 Views
The contains() method of AbstractSequentialList in Java is used to check whether an element is in the Collection.The syntax is as followspublic boolean contains(Object ob)Here, ob is the element to be checked. To work with the AbstractSequentialList class in Java, you need to import the following packageimport java.util.AbstractSequentialList;The following is an example to implement AbstractSequentialList contains() method in JavaExample Live Demoimport java.util.LinkedList; import java.util.AbstractSequentialList; public class Demo { public static void main(String[] args) { AbstractSequentialList absSequential = new LinkedList(); absSequential.add(250); ... Read More

92 Views
The noneMatch() method of the LongStream class in Java returns whether no elements of this stream match the provided predicate.The syntax is as followsboolean noneMatch(LongPredicate predicate)Here, the parameter predicate is a stateless predicate to apply to elements of this stream. However, LongPredicate in the syntax represents a predicate (boolean-valued function) of one long-valued argument.To use the LongStream class in Java, import the following packageimport java.util.stream.LongStream;The method returns true if either no elements of the stream match the provided predicate or the stream is empty. The following is an example to implement LongStream noneMatch() method in JavaExample Live Demoimport java.util.stream.LongStream; public class ... Read More

561 Views
The peek() method in the IntStream class in Java returns a stream consisting of the elements of this stream. It additionally performs the provided action on each element as elements are consumed from the resulting stream.The syntax is as followsIntStream peek(IntConsumer action)Here, the parameter action is a non-interfering action to perform on the elements as they are consumed from the stream. The IntConsumer represents an operation that accepts a single int-valued argument and returns no result.The following is an example to implement IntStream peek() method in JavaExample Live Demoimport java.util.*; import java.util.stream.IntStream; public class Demo { public static ... Read More