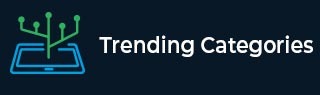
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

89 Views
The mapToLong() method of the DoubleStream class returns a LongStream consisting of the results of applying the given function to the elements of this stream.The syntax is as followsLongStream mapToLong(DoubleToLongFunction mapper)Here, the parameter mapper is a stateless function to apply to each element. The DoubleToLongFunction here is a function that accepts a double-valued argument and produces a long-valued result.To use the DoubleStream class in Java, import the following packageimport java.util.stream.DoubleStream;Create a DoubleStream and add some elementsDoubleStream doubleStream = DoubleStream.of(30.5, 45.8, 89.3);Now, use the LongStream and set a condition for the stream elementsLongStream longStream = doubleStream.mapToLong(a -> (long)a); The following is ... Read More

90 Views
The hash code value of the LocalTime can be obtained using the hashCode() method in the LocalTime class in Java. This method requires no parameters and it returns the hash code value of the LocalTime.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalTime lt = LocalTime.parse("23:15:30"); System.out.println("The LocalTime is: " + lt); System.out.println("The hash code is: " + lt.hashCode()); } }outputThe LocalTime is: 23:15:30 The hash code is: -387418074Now let us understand the above program.First the LocalTime is displayed. ... Read More

119 Views
The boxed() method of the DoubleStream class returns a Stream consisting of the elements of this stream, boxed to Double.The syntax is as followsStream boxed()Here, Double is the class that wraps a value of the primitive type double in an object. To work with DoubleStream class in Java, import the following pageimport java.util.stream.DoubleStream;The following is an example to implement DoubleStream boxed() method in JavaExample Live Demoimport java.util.*; import java.util.stream.Stream; import java.util.stream.DoubleStream; public class Demo { public static void main(String[] args) { DoubleStream doubleStream = DoubleStream.of(80.2, 84.6, 88.9, 92.9); ... Read More

92 Views
The second of minute for a particular LocalTime can be obtained using the getSecond() method in the LocalTime class in Java. This method requires no parameters and it returns the second of the minute in the range of 0 to 59.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalTime lt = LocalTime.parse("23:15:30"); System.out.println("The LocalTime is: " + lt); System.out.println("The second is: " + lt.getSecond()); } }outputThe LocalTime is: 23:15:30 The second is: 30Now let us understand ... Read More

71 Views
The noneMatch() method of the DoubleStream class returns true if none of the elements of this stream match the provided predicate.The syntax is as followsboolean noneMatch(DoublePredicate predicate)Here, predicate is a stateless predicate to apply to elements of this stream. To use the DoubleStream class in Java, import the following packageimport java.util.stream.DoubleStream;Create DoubleStream and add some elements to the streamDoubleStream doubleStream = DoubleStream.of(15.8, 28.7, 35.7, 48.1, 78.9);Now, TRUE is returned if none of the element match the conditionboolean res = doubleStream.noneMatch(num -> num > 90); The following is an example to implement DoubleStream noneMatch() method in JavaExample Live Demoimport java.util.*; import java.util.stream.DoubleStream; ... Read More

151 Views
The nanosecond of second for a particular LocalTime can be obtained using the getNano() method in the LocalTime class in Java. This method requires no parameters and it returns the nanosecond of second in the range of 0 to 999, 999, 999.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalTime lt = LocalTime.parse("23:15:30.53"); System.out.println("The LocalTime is: " + lt); System.out.println("The nanosecond is: " + lt.getNano()); } }outputThe LocalTime is: 23:15:30.530 The nanosecond is: 530000000Now let us ... Read More

136 Views
The sum() method of the DoubleStream class in Java returns the sum of elements in this stream.The syntax is as followsdouble sum()To use the DoubleStream class in Java, import the following packageimport java.util.stream.DoubleStream;Create DoubleStream and add some elementsDoubleStream doubleStream = DoubleStream.of(23.6, 45.3, 59.6, 60.6, 73.6, 84.7, 94.8);Now, sum the elements of the streamdouble sum = doubleStream.sum(); The following is an example to implement DoubleStream sum() method in JavaExample Live Demoimport java.util.*; import java.util.stream.DoubleStream; public class Demo { public static void main(String[] args) { DoubleStream doubleStream = DoubleStream.of(23.6, 45.3, 59.6, 60.6, 73.6, 84.7, 94.8); ... Read More

2K+ Views
Collections reverse order class is a reverse order method, which is encoded in the collections class. It is present in the java.util package. It returns a comparator as a result, which is a predefined comparator in nature. By using this comparator package, we can rearrange the collections of a particular data set in a reverse manner. The Collectors toSet() is a collector class, which itself returns a collector and accumulates those particular elements as an input into a new set. toSet() is an unordered collector class which is not accountable to preserve the order of the encounter of some input ... Read More