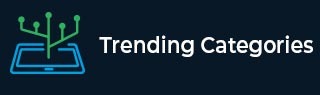
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

146 Views
It can be checked if a particular Instant object is after the other Instant object in a timeline using the isAfter() method in the Instant class in Java. This method requires a single parameter i.e. the Instant object that is to be compared. It returns true if the Instant object is after the other Instant object and false otherwise.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; import java.time.temporal.ChronoUnit; public class Demo { public static void main(String[] args) { Instant i1 = Instant.parse("2019-01-13T16:10:35.00Z"); Instant i2 = Instant.parse("2019-01-13T11:19:28.00Z"); boolean ... Read More

166 Views
It can be checked if a particular Instant object is before the other Instant object in a timeline using the isBefore() method in the Instant class in Java. This method requires a single parameter i.e. the Instant object that is to be compared. It returns true if the Instant object is before the other Instant object and false otherwise.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; import java.time.temporal.ChronoUnit; public class Demo { public static void main(String[] args) { Instant i1 = Instant.parse("2019-01-13T11:45:13.00Z"); Instant i2 = Instant.parse("2019-01-13T15:30:12.00Z"); boolean ... Read More

162 Views
An immutable copy of a Period with the number of days as required is done using the method withDays() in the Period class in Java. This method requires a single parameter i.e. the number of days in the Period and it returns the Period with the number of days as required.A program that demonstrates this is given as followsExample Live Demoimport java.time.Period; public class Demo { public static void main(String[] args) { String period = "P5Y7M15D"; Period p1 = Period.parse(period); System.out.println("The Period is: " + p1); Period p2 ... Read More

80 Views
To get the string representation of the CopyOnWriteArrayList, use the toString() method in Java.The syntax is as followsString toString()To work with CopyOnWriteArrayList class, you need to import the following packageimport java.util.concurrent.CopyOnWriteArrayList;The following is an example to implement CopyOnWriteArrayList class toString() method in JavaExample Live Demoimport java.util.concurrent.CopyOnWriteArrayList; public class Demo { public static void main(String[] args) { CopyOnWriteArrayList arrList = new CopyOnWriteArrayList(); arrList.add(100); arrList.add(250); arrList.add(0, 400); arrList.add(1, 500); arrList.add(2, 650); arrList.add(700); arrList.add(800); System.out.println("CopyOnWriteArrayList String Representation = " + arrList.toString()); } }OutputCopyOnWriteArrayList String Representation = [400, 500, 650, 100, 250, 700, 800]

118 Views
An immutable copy of a Period with the number of years as required is done using the method withYears() in the Period class in Java. This method requires a single parameter i.e. the number of years in the Period and it returns the Period with the number of years as required.A program that demonstrates this is given as followsExample Live Demoimport java.time.Period; public class Demo { public static void main(String[] args) { String period = "P5Y7M15D"; Period p1 = Period.parse(period); System.out.println("The Period is: " + p1); Period p2 ... Read More

120 Views
An immutable copy of a Period with the number of months as required is done using the method withMonths() in the Period class in Java. This method requires a single parameter i.e. the number of months in the Period and it returns the Period with the number of months as required.A program that demonstrates this is given as followsExample Live Demoimport java.time.Period; public class Demo { public static void main(String[] args) { String period = "P5Y7M15D"; Period p1 = Period.parse(period); System.out.println("The Period is: " + p1); Period p2 ... Read More

57 Views
The long value of a specified Chronofield can be obtained using the getLong() method in the MonthDay class in Java. This method requires a single parameter i.e. the ChronoField and it returns the long value of a specified Chronofield.A program that demonstrates this is given as followsExample Live Demoimport java.util.*; import java.time.*; import java.time.temporal.ChronoField; public class Demo { public static void main(String[] args) { MonthDay md = MonthDay.parse("--02-22"); System.out.println("The MonthDay is: " + md); System.out.println("The MONTH_OF_YEAR is: " + md.getLong(ChronoField.MONTH_OF_YEAR)); } }OutputThe MonthDay is: --02-22 The MONTH_OF_YEAR ... Read More

53 Views
The month of the year is obtained using getMonthValue() method in the MonthDay class in Java. This method requires no parameter and it returns the month of the year which may be in the range of 1 to 12.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { MonthDay md = MonthDay.parse("--02-22"); System.out.println("The MonthDay is: " + md); System.out.println("The month is: " + md.getMonthValue()); } }OutputThe MonthDay is: --02-22 The month is: 2Now let us understand the above ... Read More

76 Views
Using the add() method, insert the specified element at the specified position in the CopyOnWriteArrayList.The syntax is as followsvoid add(int index, E ele)Here, the parameter index is where you want to insert the element and ele is the element to be inserted to this list. To work with CopyOnWriteArrayList class, you need to import the following packageimport java.util.concurrent.CopyOnWriteArrayList;The following is an example to add elements in the CopyOnWriteArrayList class in JavaExample Live Demoimport java.util.concurrent.CopyOnWriteArrayList; public class Demo { public static void main(String[] args) { CopyOnWriteArrayList arrList = new CopyOnWriteArrayList(); arrList.add(100); arrList.add(250); ... Read More

165 Views
The month name for a particular MonthDay can be obtained using the getMonth() method in the MonthDay class in Java. This method requires no parameters and it returns the month name in the year.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { MonthDay md = MonthDay.parse("--02-22"); System.out.println("The MonthDay is: " + md); System.out.println("The month is: " + md.getMonth()); } }OutputThe MonthDay is: --02-22 The month is: FEBRUARYNow let us understand the above program.First the MonthDay is displayed. ... Read More