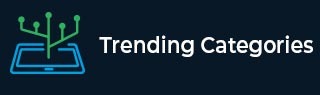
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

151 Views
An immutable copy of a LocalDateTime where the required duration is added to it can be obtained using the plus() method in the LocalDateTime class in Java. This method requires two parameters i.e. the duration to be added and the TemporalUnit of the duration. Also, it returns the LocalDateTime object with the required duration added to it.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; import java.time.temporal.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.now(); System.out.println("The LocalDateTime is: " + ldt); ... Read More

2K+ Views
An immutable copy of a LocalDateTime where the required duration is subtracted from it can be obtained using the minus() method in the LocalDateTime class in Java. This method requires two parameters i.e. the duration to be subtracted and the TemporalUnit of the duration. Also, it returns the LocalDateTime object with the required duration subtracted from it.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; import java.time.temporal.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.now(); System.out.println("The LocalDateTime is: " + ldt); ... Read More

159 Views
The LocalDateTime instance can be obtained from a string value using the parse() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the string which is to be parsed. This string cannot be null. Also, it returns the LocalDateTime instance obtained from the string value that was passed as a parameter.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.parse("2019-02-18T23:15:30"); System.out.println("The LocalDateTime is: " + ldt); } }OutputThe LocalDateTime is: 2019-02-18T23:15:30Now ... Read More

80 Views
The LocalDateTime object can be queried as required using the query method in the LocalDateTime class in Java. This method requires a single parameter i.e. the query to be invoked and it returns the result of the query.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; import java.time.temporal.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.parse("2019-02-18T23:15:30"); System.out.println("The LocalDateTime is: " + ldt); String precision = ldt.query(TemporalQueries.precision()).toString(); System.out.println("The Precision for the LocalDateTime is: "+ precision); } }OutputThe LocalDateTime ... Read More

2K+ Views
The current date-time can be obtained from the system clock in the default time zone using the now() method in the LocalDateTime class in Java. This method requires no parameters and it returns the current date-time from the system clock in the default time zoneA program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.now(); System.out.println("The LocalDateTime is: " + ldt); } }OutputThe LocalDateTime is: 2019-02-18T06:04:31.369Now let us understand the above program.The current date-time is obtained from ... Read More

3K+ Views
The difference between two LocalDateTime objects can be obtained using the until() method in the LocalDateTime class in Java. This method requires two parameters i.e. the end date for the LocalDateTime object and the Temporal unit. Also, it returns the difference between two LocalDateTime objects in the Temporal unit specified.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; import java.time.temporal.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt1 = LocalDateTime.parse("2019-02-18T23:15:30"); LocalDateTime ldt2 = LocalDateTime.parse("2019-02-19T12:21:30"); System.out.println("The first LocalDateTime is: " + ldt1); ... Read More

126 Views
The range of values for a ChronoField can be obtained using the range() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the ChronoField for which the range of values is required and it returns the range of values.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; import java.time.temporal.ChronoField; import java.time.temporal.ValueRange; public class Main { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.parse("2019-02-18T11:19:50"); System.out.println("The LocalDateTime is: " + ldt); ValueRange dowRange = ldt.range(ChronoField.DAY_OF_WEEK); System.out.println("The range of DAY_OF_WEEK: ... Read More

83 Views
An immutable copy of a LocalDateTime object where some seconds are added to it can be obtained using the plusSeconds() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the number of seconds to be added and it returns the LocalDateTime object with the added seconds.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.now(); System.out.println("The current LocalDateTime is: " + ldt); System.out.println("The LocalDateTime with 5 seconds added is: ... Read More

172 Views
An immutable copy of a LocalDateTime object where some days are added to it can be obtained using the plusDays() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the number of days to be added and it returns the LocalDateTime object with the added days.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.now(); System.out.println("The current LocalDateTime is: " + ldt); System.out.println("The LocalDateTime with 10 days added is: ... Read More

104 Views
An immutable copy of a LocalDateTime object where some months are added to it can be obtained using the plusMonths() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the number of months to be added and it returns the LocalDateTime object with the added months.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.now(); System.out.println("The current LocalDateTime is: " + ldt); System.out.println("The LocalDateTime with 5 months added is: ... Read More