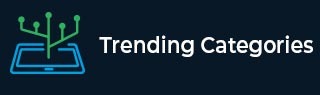
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

39 Views
The minute of the hour for a particular LocalDateTime can be obtained using the getMinute() method in the LocalDateTime class in Java. This method requires no parameters and it returns the minute of the hour in the range of 0 to 59.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.parse("2019-02-18T23:15:30"); System.out.println("The LocalDateTime is: " + ldt); System.out.println("The minute is: " + ldt.getMinute()); } }OutputThe LocalDateTime is: 2019-02-18T23:15:30 The minute is: 15Now let ... Read More

79 Views
The nanosecond of second for a particular LocalDateTime can be obtained using the getNano() method in the LocalDateTime class in Java. This method requires no parameters and it returns the nanosecond of second in the range of 0 to 999, 999, 999.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.parse("2019-02-18T23:15:30.53"); System.out.println("The LocalDateTime is: " + ldt); System.out.println("The nanosecond is: " + ldt.getNano()); } }OutputThe LocalDateTime is: 2019-02-18T23:15:30.530 The nanosecond is: 530000000Now let ... Read More

169 Views
The month name for a particular LocalDateTime can be obtained using the getMonth() method in the LocalDateTime class in Java. This method requires no parameters and it returns the month name in the year.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.parse("2019-02-18T23:15:30"); System.out.println("The LocalDateTime is: " + ldt); System.out.println("The month is: " + ldt.getMonth()); } }OutputThe LocalDateTime is: 2019-02-18T23:15:30 The month is: FEBRUARYNow let us understand the above program.First the LocalDateTime is ... Read More

219 Views
Two LocalDateTime objects can be compared using the compareTo() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the LocalDateTime object to be compared.If the first LocalDateTime object is greater than the second LocalDateTime object it returns a positive number, if the first LocalDateTime object is lesser than the second LocalDateTime object it returns a negative number and if both the LocalDateTime objects are equal it returns zero.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Main { public static void main(String[] args) { LocalDateTime ldt1 ... Read More

48 Views
An instance of a LocalDateTime object can be obtained from a Temporal object using the from() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the Temporal object and it returns the LocalDateTime object that is obtained from the Temporal object.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Main { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.from(ZonedDateTime.now()); System.out.println("The LocalDateTime is: " + ldt); } }OutputThe LocalDateTime is: 2019-02-18T09:55:05.489Now let us understand the above program.The instance of the LocalDateTime ... Read More

39 Views
An immutable copy of a LocalDateTime with the month altered as required is done using the method withMonth() in the LocalDateTime class in Java. This method requires a single parameter i.e. the month that is to be set in the LocalDateTime and it returns the LocalDateTime with the month altered as required.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Main { public static void main(String[] args) { LocalDateTime ldt1 = LocalDateTime.parse("2019-02-18T23:15:30"); System.out.println("The LocalDateTime is: " + ldt1); LocalDateTime ldt2 = ldt1.withMonth(7); ... Read More

19 Views
An immutable copy of a LocalDateTime with the minutes altered as required is done using the method withMinute() in the LocalDateTime class in Java. This method requires a single parameter i.e. the minute that is to be set in the LocalDateTime and it returns the LocalDateTime with the minute altered as required.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Main { public static void main(String[] args) { LocalDateTime ldt1 = LocalDateTime.parse("2019-02-18T23:15:30"); System.out.println("The LocalDateTime is: " + ldt1); LocalDateTime ldt2 = ldt1.withMinute(45); ... Read More

71 Views
An immutable copy of a LocalDateTime object where some minutes are added to it can be obtained using the plusMinutes() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the number of minutes to be added and it returns the LocalDateTime object with the added minutes.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.now(); System.out.println("The current LocalDateTime is: " + ldt); System.out.println("The LocalDateTime with 15 minutes added is: ... Read More

33 Views
An immutable copy of a LocalDateTime with the hour altered as required is done using the method withHour() in the LocalDateTime class in Java. This method requires a single parameter i.e. the hour that is to be set in the LocalDateTime and it returns the LocalDateTime with the hour altered as required.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Main { public static void main(String[] args) { LocalDateTime ldt1 = LocalDateTime.parse("2019-02-18T23:15:30"); System.out.println("The LocalDateTime is: " + ldt1); LocalDateTime ldt2 = ldt1.withHour(5); ... Read More

90 Views
An immutable copy of a LocalDateTime object where some hours are added to it can be obtained using the plusHours() method in the LocalDateTime class in Java. This method requires a single parameter i.e. the number of hours to be added and it returns the LocalDateTime object with the added hours.A program that demonstrates this is given as follows −Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDateTime ldt = LocalDateTime.now(); System.out.println("The current LocalDateTime is: " + ldt); System.out.println("The LocalDateTime with 3 hours added is: ... Read More