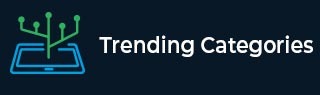
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

10K+ Views
The average() method of the IntStream class in Java returns an OptionalDouble describing the arithmetic mean of elements of this stream, or an empty optional if this stream is empty. It gets the average of the elements of the stream.The syntax is as followsOptionalDouble average()Here, OptionalDouble is a container object which may or may not contain a double value.Create an IntStream with some elementsIntStream intStream = IntStream.of(15, 13, 45, 18, 89, 70, 76, 56);Now, get the average of the elements of the streamOptionalDouble res = intStream.average();The following is an example to implement IntStream average() method in Java. The isPresent() method ... Read More

65 Views
The DoubleStream.iterate() returns an infinite sequential ordered DoubleStream produced by iterative application of a function f to an initial element seed, producing a Stream consisting of seed.The syntax is as followsstatic DoubleStream iterate(double seed, DoubleUnaryOperator f)Here, seed is the initial element and f is a function to be applied to the previous element to produce a new element.To use the DoubleStream class in Java, import the following packageimport java.util.stream.DoubleStream;The following is an example to generate infinite stream of Double in Java with DoubleStream.iterate()Exampleimport java.util.stream.DoubleStream; public class Demo { public static void main(String[] args) { ... Read More

67 Views
The build() method of the LongStream.Builder class builds the stream, transitioning this builder to the built state.The syntax is as followsLongStream build()To use the LongStream.Builder class in Java, import the following packageimport java.util.stream.LongStream;The following is an example to implement LongStream.Builder build() method in JavaExample Live Demoimport java.util.stream.LongStream; public class Demo { public static void main(String[] args) { LongStream.Builder builder = LongStream.builder(); builder.accept(255L); builder.accept(570L); builder.accept(355L); builder.accept(155L); ... Read More

88 Views
The count() method of the IntStream class in Java returns the count of elements in this streamThe syntax is as followslong count()First, create an IntStream and add some elementsIntStream intStream = IntStream.of(30, 13, 67, 56, 89, 99, 76, 56);Now, get the count of elements of the stream using the count() methodlong num = intStream.count();The following is an example to implement IntStream count() method in JavaExample Live Demoimport java.util.*; import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { IntStream intStream = IntStream.of(30, 13, 67, 56, 89, 99, 76, 56); long num = ... Read More

354 Views
The IntStream min() method in the Java IntStream class is used to get the minimum element from the stream. It returns an OptionalInt describing the minimum element of this stream, or an empty optional if this stream is empty.The syntax is as followsOptionalInt min()Here, OptionalInt is a container object which may or may not contain an int value.Create an IntStream and add some elementsIntStream intStream = IntStream.of(89, 45, 67, 12, 78, 99, 100);Now, get the minimum element of the streamOptionalInt res = intStream.min();The following is an example to implement IntStream min() method in Java. The isPresent() method of the OptionalInt ... Read More

115 Views
The forEach() method of the LongStream class in Java performs an action for each element of this stream.The syntax is as followsvoid forEach(LongConsumer action)Here, the parameter action is a non-interfering action to perform on the elements. LongConsumer represents an operation that accepts a single long-valued argument and returns no result.To use the LongStream class in Java, import the following packageimport java.util.stream.LongStream;The following is an example to implement LongStream forEach() method in JavaExample Live Demoimport java.util.*; import java.util.stream.LongStream; public class Demo { public static void main(String[] args) { LongStream longStream = LongStream.of(1000L, 12000L, ... Read More

62 Views
The lastIndexOf() method of the AbstractList return the index of the last occurrence of an element in the list. If the element does not exist in the list, then -1 is returned.The syntax is as followspublic int lastIndexOf(Object ob)Here, the parameter ob is the element to be searched. To work with the AbstractList class, import the following packageimport java.util.AbstractList;The following is an example to implement lastIndexOf() method of the AbstractlList class in JavaExample Live Demoimport java.util.ArrayList; import java.util.AbstractList; public class Demo { public static void main(String[] args) { AbstractList myList = new ArrayList(); myList.add(75); ... Read More

216 Views
The skip() method of the IntStream class in Java returns a stream consisting of the remaining elements of this stream after discarding the first n elements of the stream.The syntax is as followsIntStream skip(long n)Here, n is the number of elements to skip. The method returns the new stream.Create an IntStream and add some elements within a range using range() methodIntStream intStream = IntStream.range(20, 40);Now to skip some elements and only display the rest of them, use the skip() methodintStream.skip(15)The following is an example to implement IntStream skip() method in Java. It skips 15 elements since we have set the ... Read More

375 Views
The generate() method in the IntStream class returns an infinite sequential unordered stream where each element is generated by the provided IntSupplier.The syntax is as followsstatic IntStream generate(IntSupplier i)Here, i is the IntSupplier for generated elements. The IntSupplier represents a supplier of int-valued results.The following is an example to implement IntStream generate() method in Java. We have used the limit() method here as well to limit the number of elements we want from the streamExample Live Demoimport java.util.*; import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { IntStream intStream = IntStream.generate(() ... Read More

474 Views
The allMatch() method of the IntStream class in Java returns whether all elements of this stream match the provided predicate.The syntax is as followsboolean allMatch(IntPredicate predicate)Here, the predicate parameter is a stateless predicate to apply to elements of this stream. The IntPredicate represents a predicate of one int-valued argument.The allMatch() method returns true if either all elements of the stream match the provided predicate or the stream is empty.The following is an example to implement IntStream allMatch() method in JavaExample Live Demoimport java.util.*; import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { ... Read More