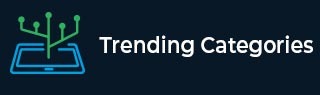
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

90 Views
An Instant can be combined with a timezone to create a ZonedDateTime object using the atZone() method in the Instant class in Java. This method requires a single parameter i.e. the ZoneID and it returns the ZonedDateTime object.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { Instant i = Instant.parse("2019-01-13T18:35:19.00Z"); System.out.println("The Instant object is: " + i); ZonedDateTime zdt = i.atZone(ZoneId.of("Australia/Melbourne")); System.out.println("The ZonedDateTime object is: " + zdt); } }OutputThe Instant object is: 2019-01-13T18:35:19Z ... Read More

87 Views
The skip() method of the DoubleStream class in Java returns a stream consisting of the remaining elements of this stream after discarding the first numEle elements of the stream. The numEle is a parameter which you can set to skip any number of elements from the stream.The syntax is as follows −DoubleStream skip(long numEle)Here, numEle is the number of elements to skip. The leading elements are skipped.To use the DoubleStream class in Java, import the following package −import java.util.stream.DoubleStream;The following is an example to implement DoubleStream skip() method in Java −Example Live Demoimport java.util.stream.DoubleStream; public class Demo { ... Read More

132 Views
The anyMatch() method of the LongStream class in Java returns whether any elements of this stream match the provided predicate.The syntax is as follows.boolean anyMatch(LongPredicate predicate)Here, the parameter predicate is the stateless predicate to apply to elements of this stream. The LongPredicate represents a predicate of one long-valued argument.To use the LongStream class in Java, import the following package.import java.util.stream.LongStream;The following is an example to implement LongStream anyMatch() method in Java.Example Live Demoimport java.util.stream.LongStream; public class Demo { public static void main(String[] args) { LongStream longStream = LongStream.of(100L, 150L, 200L, 300L, 400L, 500L); boolean ... Read More

103 Views
The LongStream class in Java the following two forms of the of() method.The following of() method returns a sequential LongStream containing a single element. Here is the syntaxstatic LongStream of(long t)Here, parameter t is the single element.The following of() method returns a sequential ordered stream whose elements are the specified values.static LongStream of(long… values)Here, the parameter values are the elements of the new stream.To use the LongStream class in Java, import the following package.import java.util.stream.LongStream;The following is an example to implement LongStream of() method in Java.Example Live Demoimport java.util.stream.LongStream; public class Demo { public static void main(String[] args) { ... Read More

2K+ Views
Two Instant objects can be compared using the compareTo() method in the Instant class in Java. This method requires a single parameter i.e. the Instant object to be compared.If the first Instant object is greater than the second Instant object it returns a positive number, if the first Instant object is lesser than the second Instant object it returns a negative number and if both the Instant objects are equal it returns zero.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { Instant i1 = ... Read More

88 Views
The findFirst() method of the LongStream class in Java returns an OptionalLong describing the first element of this stream, or an empty OptionalLong if the stream is empty.The syntax is as follows.OptionalLong findFirst()Here, OptionalLong is a container object which may or may not contain a long value. For OptionalLong, import the following package.import java.util.OptionalLong;To use the LongStream class in Java, import the following package.import java.util.stream.LongStream;Create a LongStream and add elements.LongStream longStream = LongStream.of(25000L, 35000L, 40000L, 50000L, 60000L);Now, get the first element from the stream.OptionalLong res = longStream.findFirst();The following is an example to implement LongStream findFirst() method in Java.Example Live Demoimport java.util.OptionalLong; ... Read More

2K+ Views
The toList() method of the Collectors class returns a Collector that accumulates the input elements into a new List.The syntax is as follows −static Collector toList()Here, parameter T is the type of input elements.To work with Collectors class in Java, import the following package −import java.util.stream.Collectors;The following is an example to implement Collectors toList() method in Java −Example Live Demoimport java.util.List; import java.util.stream.Collectors; import java.util.stream.Stream; public class Demo { public static void main(String[] args) { Stream stream = Stream.of("25", "40", "90", "150", "180", "220", "350"); ... Read More

794 Views
The equality of two Instant objects can be determined using the equals() method in the Instant class in Java. This method requires a single parameter i.e. the Instant to be compared. Also it returns true if both the Instant objects are equal and false otherwise.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; import java.time.temporal.ChronoUnit; public class Demo { public static void main(String[] args) { Instant i1 = Instant.parse("2019-01-13T16:10:35.00Z"); Instant i2 = Instant.parse("2019-01-13T16:10:35.00Z"); System.out.println("Instant object i1 is: " + i1); System.out.println("Instant object i2 is: " ... Read More

408 Views
The filter() method of the IntStream class in Java returns a stream consisting of the elements of this stream that match the given predicate.The syntax is as follows −IntStream filter(IntPredicate predicate)Here, the predicate parameter is a stateless predicate to apply to each element to determine if it should be included. The IntPredicate above is the predicate of one int-valued argument.Create an IntStream and add elements −IntStream intStream = IntStream.of(20, 34, 45, 67, 89, 100);Now, set a condition and filter stream elements based on it using the filter() method −intStream.filter(a -> a < 50).The following is an example to implement IntStream ... Read More

80 Views
The empty() method of the LongStream class in Java returns an empty sequential LongStream.The syntax is as follows.static LongStream empty()To use the LongStream class in Java, import the following package.import java.util.stream.LongStream;The following is an example to implement LongStream empty() method in Java.Example Live Demoimport java.util.stream.LongStream; public class GFG { public static void main(String[] args) { LongStream longStream = LongStream.empty(); System.out.println("The count of elements in the stream: "+longStream.count()); } }OutputThe count of elements in the stream: 0