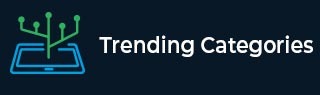
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

173 Views
The boxed() method of the LongStream class returns a Stream consisting of the elements of this stream, each boxed to a Long.The syntax is as follows.Stream boxed()Here, Stream represents a sequence of elements. To use the LongStream class in Java, import the following package.import java.util.stream.LongStream;Create an IntStream and add some elements in a range using the range() method.LongStream intStream = LongStream.range(20800L, 20805L);Now, use the boxed() methodStream s = intStream.boxed();The following is an example to implement LongStream boxed() method in Java.Example Live Demoimport java.util.stream.Stream; import java.util.stream.LongStream; public class Demo { public static void main(String[] args) { LongStream intStream ... Read More

83 Views
The summaryStatistics() method in the LongStream class in Java returns a LongSummaryStatistics describing various summary data about the elements of this stream.The syntax is as follows −LongSummaryStatistics summaryStatistics()Here, LongSummaryStatistics is a state object for collecting statistics such as count, min, max, etc.To use the LongStream class in Java, import the following packageimport java.util.stream.LongStream;Create LongStream and add some elements −LongStream longStream = LongStream.of(30000L, 15000L, 20000l, 25000l, 30000l);Now, get the statistics −LongSummaryStatistics info = longStream.summaryStatistics(); The following is an example to implement LongStream summaryStatistics() method in Java −Example Live Demoimport java.util.stream.LongStream; import java.util.LongSummaryStatistics; public class Demo { public static ... Read More

93 Views
The toArray() method of the LongStream class returns an array containing the elements of this stream.The syntax is as follows.long[] toArray()To use the LongStream class in Java, import the following package.import java.util.stream.LongStream;Create LongStream and add some elements.LongStream longStream = LongStream.of(25000L, 28999L, 6767788L);Create a Long array and use the toArray() method to return the elements of the stream as array elements.long[] myArr = longStream.toArray();The following is an example to implement LongStream toArray() method in Java.Example Live Demoimport java.util.*; import java.util.stream.LongStream; public class Demo { public static void main(String[] args) { LongStream longStream = LongStream.of(25000L, 28999L, 6767788L); ... Read More

64 Views
The toArray() method is used to return an array containing all the elements in this list in proper sequence.The syntax is as follows −Object[] toArray()To work with CopyOnWriteArrayList class, you need to import the following package −import java.util.concurrent.CopyOnWriteArrayList;The following is an example to implement CopyOnWriteArrayList class toArray() method in Java−Example Live Demoimport java.util.Arrays; import java.util.concurrent.CopyOnWriteArrayList; public class Demo { public static void main(String[] args) { CopyOnWriteArrayList arrList = new CopyOnWriteArrayList(); arrList.add(220); arrList.add(250); arrList.add(400); ... Read More

76 Views
To create Ennead Tuple from an array, use the fromArray() method. Using this method, create an Ennead Tuple using arrays in Java.Let us first see what we need to work with JavaTuples. To work with Ennead class in JavaTuples, you need to import the following package.import org.javatuples.Ennead;Note Download JavaTuples Jar library to run JavaTuples program. If you are using Eclipse IDE, then Right Click Project -> Properties -> Java Build Path -> Add External Jars and upload the downloaded JavaTuples jar file. Refer the below guide for all the steps to run JavaTuples.Steps: How to run JavaTuples program in EclipseThe ... Read More

74 Views
You can create Octel Tuple from another collection i.e. List or arrays. For List, use the fromCollection() method.Let us first see what we need to work with JavaTuples. To work with Octet class in JavaTuples, you need to import the following package −import org.javatuples.Octet;Note: Download JavaTuples Jar library to run JavaTuples program. If you are using Eclipse IDE, then Right Click Project -> Properties -> Java Build Path -> Add External Jars and upload the downloaded JavaTuples jar file. Refer the below guide for all the steps to run JavaTuples −Steps: How to run JavaTuples program in EclipseThe following is an ... Read More

2K+ Views
The boxed() method of the IntStream class returns a Stream consisting of the elements of this stream, each boxed to an Integer.The syntax is as follows.Stream boxed()At first, create an IntStreamIntStream intStream = IntStream.range(20, 30);Now, use the boxed() method to return a Stream consisting of the elements of this stream, each boxed to an Integer.Stream s = intStream.boxed();The following is an example to implement IntStream boxed() method in Java.Example Live Demoimport java.util.*; import java.util.stream.Stream; import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { IntStream intStream = IntStream.range(20, 30); Stream s = ... Read More

94 Views
The indexOf() method is used to return the index of the first occurrence of the specified element in this list. If the list is empty, it returns -1.The syntax is as follows.int indexOf(Object ob)Here, the parameter ob is the element to search for.To work with the AbstractList class, import the following package.import java.util.AbstractList;The following is an example to implement indexOf() method of the AbstractlList class in Java.Example Live Demoimport java.util.ArrayList; import java.util.AbstractList; public class Demo { public static void main(String[] args) { AbstractList myList = new ArrayList(); myList.add(5); myList.add(20); ... Read More

2K+ Views
The joining() method of the Collectors class in Java 8 returns a Collector that concatenates the input elements into a String, in encounter order.The syntax is as follows −public static Collector joining()Here, CharSequence is a readable sequence of char values, whereas String class represents character strings.To work with Collectors class in Java, import the following package −import java.util.stream.Collectors;The following is an example to implement Collectors.joining() method in Java 8 −Example Live Demoimport java.util.stream.Collectors; import java.util.stream.Stream; import java.util.Arrays; import java.util.List; public class Demo { public static void main(String[] args) { List list ... Read More

82 Views
The subList() method returns a part of this list between the specified fromIndex, inclusive, and toIndex, exclusive. Get a sublist using the method by setting the range as the two parameters.The syntax is as follows.public List subList(int fromIndex, int toIndex)Here, the parameter fromIndex is the low endpoint (inclusive) of the subList and toIndex is the high endpoint (exclusive) of the subList.To work with the AbstractList class, import the following package.import java.util.AbstractList;The following is an example to implement subList() method of the AbstractlList class in Java.Exampleimport java.util.ArrayList; import java.util.AbstractList; public class Demo { public static void main(String[] args) { ... Read More