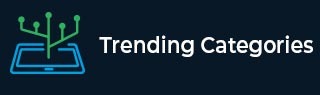
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

168 Views
The max() method of the DoubleStream class returns an OptionalDouble describing the maximum element of this stream, or an empty OptionalDouble if this stream is empty.The syntax is as follows.OptionalDouble max()Here, OptionalDouble is a container object which may or may not contain a double value.To use the DoubleStream class in Java, import the following package.import java.util.stream.DoubleStream;Create a DoubleStream and add some elements.DoubleStream doubleStream = DoubleStream.of(67.9, 89.9, 10.5, 95.8, 49.6);Now, get the maximum element from the stream.OptionalDouble res = doubleStream.max();The following is an example to implement DoubleStream max() method in Java.Example Live Demoimport java.util.OptionalDouble; import java.util.stream.DoubleStream; public class Demo { public ... Read More

96 Views
An immutable copy of the Period object where some months are added to it can be obtained using the plusMonths() method in the Period class in Java. This method requires a single parameter i.e. the number of months to be added and it returns the Period object with the added months.A program that demonstrates this is given as follows:Example Live Demoimport java.time.Period; public class Demo { public static void main(String[] args) { String period = "P5Y7M15D"; Period p1 = Period.parse(period); System.out.println("The Period is: " + p1); Period p2 ... Read More

67 Views
Use the contains() method to search for a value in the KeyValue tuple. Let us first see what we need to work with JavaTuples. To work with KeyValue class in JavaTuples, you need to import the following package.import org.javatuples.KeyValue;Note Download JavaTuples Jar library to run JavaTuples program. If you are using Eclipse IDE, then Right Click Project -> Properties -> Java Build Path -> Add External Jars and upload the downloaded JavaTuples jar file. Refer the below guide for all the steps to run JavaTuples.Steps: How to run JavaTuples program in EclipseThe following is an example to implement the contains() ... Read More

96 Views
The difference between two LocalDate objects can be obtained using the until() method in the LocalDate class in Java. This method requires a single parameter i.e. the end date for the LocalDate object and it returns the difference between two LocalDate objects using a Period object.A program that demonstrates this is given as follows:Example Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDate ld1 = LocalDate.parse("2019-01-10"); LocalDate ld2 = LocalDate.parse("2019-02-14"); System.out.println("The first LocalDate is: " + ld1); System.out.println("The second LocalDate is: " + ... Read More

67 Views
The isEmpty() method of the AbstractSequentialList class is used to check whether the list is empty or not. If it is empty, then TRUE is returned, else FALSE.The syntax is as follows.public boolean isEmpty()To work with the AbstractSequentialList class in Java, you need to import the following package.import java.util.AbstractSequentialList;The following is an example to implement AbstractSequentialList isEmpty() method in Java.Example Live Demoimport java.util.LinkedList; import java.util.AbstractSequentialList; public class Demo { public static void main(String[] args) { AbstractSequentialList absSequential = new LinkedList(); absSequential.add(110); absSequential.add(320); absSequential.add(400); absSequential.add(550); absSequential.add(600); absSequential.add(700); absSequential.add(900); System.out.println("Elements ... Read More

81 Views
To fetch the value from a LabelValue class in Java, you need to use the getValue() method. To get the key, use the getLabel() method. Let us first see what we need to work with JavaTuples. To work with LabelValue class in JavaTuples, you need to import the following package −import org.javatuples.LabelValue;Note − Download JavaTuples Jar library to run JavaTuples program. If you are using Eclipse IDE, then Right Click Project -> Properties -> Java Build Path -> Add External Jars and upload the downloaded JavaTuples jar file. Refer the below guide for all the steps to run JavaTuples −Steps ... Read More

75 Views
The concat() method of the DoubleStream class creates a stream which is concatenated. The elements of the resultant stream are all the elements of the first stream followed by all the elements of the second stream.The syntax is as follows.static DoubleStream concat(DoubleStream streamOne, DoubleStream streamTwo)Here, streamOne is the first stream whereas streamTwo is the second stream.To use the DoubleStream class in Java, import the following package.import java.util.stream.DoubleStream;Create a DoubleStream with some elements.DoubleStream doubleStream1 = DoubleStream.of(20.5, 30.6, 58.9, 66.7);Create another DoubleStream.DoubleStream doubleStream2 = DoubleStream.of(71.8, 77.9, 82.3, 91.6, 98.4);Now, concat the streamsDoubleStream.concat(doubleStream1, doubleStream2)The following is an example to implement DoubleStream concat() method ... Read More

5K+ Views
The year for a particular LocalDate can be obtained using the getYear() method in the LocalDate class in Java. This method requires no parameters and it returns the year which can range from MIN_YEAR to MAX_YEAR.A program that demonstrates this is given as followsExample Live Demoimport java.time.*; public class Demo { public static void main(String[] args) { LocalDate ld = LocalDate.parse("2019-02-14"); System.out.println("The LocalDate is: " + ld); System.out.println("The year is: " + ld.getYear()); } }OutputThe LocalDate is: 2019-02-14 The year is: 2019Now let us understand the above program.First the LocalDate ... Read More

59 Views
To create LabelValue tuple from a List collection, use the fromCollection() method. Here, we will be creating LabelValue from List, therefore use the fromCollection() method.Let us first see what we need to work with JavaTuples. To work with LabelValue class in JavaTuples, you need to import the following package −import org.javatuples.LabelValue;Note − Download JavaTuples Jar library to run JavaTuples program. If you are using Eclipse IDE, then Right Click Project -> Properties -> Java Build Path -> Add External Jars and upload the downloaded JavaTuples jar file. Refer the below guide for all the steps to run JavaTuples −Steps − ... Read More

324 Views
The average() method of the LongStream class in Java returns an OptionalDouble describing the arithmetic mean of elements of this stream, or an empty optional if this stream is empty.The syntax is as follows.OptionalDouble average()Here, OptionalDouble is a container object which may or may not contain a double value.To use the LongStream class in Java, import the following package.import java.util.stream.LongStream;Create LongStream and add elements.LongStream longStream = LongStream.of(100L, 150L, 180L, 200L, 250L, 300L, 500L);Get the average of the elements in the stream.OptionalDouble res = longStream.average();The following is an example to implement LongStream average() method in Java.Example Live Demoimport java.util.*; import java.util.stream.LongStream; public ... Read More